Interviewing as a JavaScript Developer
Navigating the interview process as a JavaScript Developer is akin to demonstrating your expertise in a language that's become the backbone of the web. With JavaScript's ubiquity in front-end, back-end, and full-stack development, interviews often probe a wide spectrum of skills—from core language proficiency to framework familiarity and problem-solving acumen.
In this guide, we'll dissect the array of questions you might encounter, from intricate coding challenges to conceptual discussions that reveal your understanding of JavaScript's quirks and features. We'll provide you with the tools to articulate your experience effectively, showcase your technical prowess, and convey your potential as a collaborative team player. This resource is crafted to sharpen your interview readiness, ensuring you can confidently articulate what makes you an exceptional JavaScript Developer and secure your next career move.
Types of Questions to Expect in a JavaScript Developer Interview
In the dynamic world of JavaScript development, interviews are designed to probe not just your technical knowledge, but also your problem-solving abilities, coding standards, and understanding of best practices. Recognizing the different types of questions you may encounter can help you prepare more effectively and demonstrate your full potential as a JavaScript developer. Here's an overview of the question categories that are commonly featured in JavaScript Developer interviews.
Core Language Knowledge
Questions in this category will test your understanding of JavaScript as a language. Expect to answer queries about data types, scope, hoisting, closures, and the event loop. These questions assess your foundational knowledge and your ability to write efficient, clean code. They are intended to ensure that you have a solid grasp of the language's nuances and can apply JavaScript concepts effectively.
Framework and Library Proficiency
With the JavaScript ecosystem being rich in frameworks and libraries, you'll likely face questions about your experience with tools like React, Angular, Vue.js, or Node.js. These inquiries evaluate your ability to work within these technologies, understand their lifecycle methods, state management, and more. They are designed to gauge how well you can leverage these tools to build robust, scalable applications.
Practical Coding Challenges
These are hands-on problems where you'll be asked to write code on a whiteboard, paper, or a computer. The challenges might range from simple algorithmic problems to complex tasks that mimic real-world scenarios. These exercises test your coding skills, logic, and your approach to problem-solving under time constraints. They also provide insight into your coding style and adherence to best practices.
System Design and Architecture Questions
For more senior roles, you might be asked about system design principles, patterns, and best practices. These questions assess your ability to architect scalable and maintainable systems. You'll discuss how to structure applications, manage state, handle asynchronous operations, and optimize for performance. These inquiries aim to understand your higher-level thinking and your approach to making architectural decisions.
Behavioral and Communication Skills
These questions delve into your past experiences, teamwork, and how you handle conflict and pressure. You might be asked about a time when you had to deal with a difficult bug or collaborate with a non-technical team member. These are intended to evaluate your soft skills, which are crucial for effective collaboration in a team environment. They seek to understand your personal attributes and how they align with the company's culture and values.
Debugging and Problem-Solving Techniques
Interviewers will want to know how you approach fixing bugs and troubleshooting issues in your code. You may be given a block of code with an error and asked to identify and solve the problem. These questions test your analytical skills, attention to detail, and your methodology for resolving issues efficiently.
Preparing for these types of questions can help you navigate a JavaScript Developer interview with confidence. Understanding what each category aims to uncover about your skills and experience allows you to tailor your study and practice accordingly, showcasing your strengths and addressing any areas for improvement.
Preparing for a JavaScript Developer Interview
Preparing for a JavaScript Developer interview is a critical step in showcasing your technical expertise, problem-solving abilities, and passion for the field. It's not just about proving you can code; it's about demonstrating a deep understanding of JavaScript's nuances, best practices, and its ecosystem. A well-prepared candidate can articulate how they use JavaScript to solve complex problems, optimize performance, and build scalable, maintainable applications. By investing time in preparation, you increase your chances of standing out in a competitive job market and landing the role you desire.
How to do Interview Prep as a JavaScript Developer
- Master the JavaScript Fundamentals: Ensure you have a strong grasp of core JavaScript concepts such as closures, prototypes, this keyword, event bubbling, and scope. Understanding ES6 features like arrow functions, promises, and modules is also essential.
- Understand Asynchronous JavaScript: Be prepared to discuss and demonstrate your knowledge of asynchronous JavaScript, including callbacks, promises, async/await, and handling asynchronous operations with JavaScript.
- Know the Browser Environment: Familiarize yourself with the Document Object Model (DOM), browser APIs, event handling, and how JavaScript interacts with HTML and CSS to create dynamic web pages.
- Study Frameworks and Libraries: If the job description mentions specific frameworks or libraries like React, Angular, Vue.js, or Node.js, make sure you understand their core principles, lifecycle methods, state management, and common use cases.
- Practice Coding Challenges: Sharpen your problem-solving skills by practicing coding challenges on platforms like LeetCode, HackerRank, or CodeSignal. Focus on algorithms, data structures, and JavaScript-specific questions.
- Review Testing Strategies: Be prepared to discuss testing in JavaScript. Understand unit testing, integration testing, and end-to-end testing, as well as testing frameworks like Jest or Mocha.
- Prepare for System Design Questions: You may be asked to design a system or feature using JavaScript. Be ready to discuss how you would structure your code, manage state, and ensure the application's scalability and performance.
- Brush Up on Best Practices: Be able to talk about code readability, maintainability, and performance optimizations. Understand design patterns in JavaScript and when to use them.
- Prepare Your Own Questions: Develop thoughtful questions about the company's tech stack, development processes, and culture. This shows your genuine interest in the role and the organization.
- Mock Interviews: Practice with mock interviews focusing on JavaScript-specific questions. Get feedback from peers or mentors to improve your communication and technical explanation skills.
By following these steps, you'll be able to demonstrate not just your technical abilities, but also your commitment to the role and your potential as a valuable team member. Good preparation is the key to showing that you're not just another developer, but the right JavaScript developer for the job.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free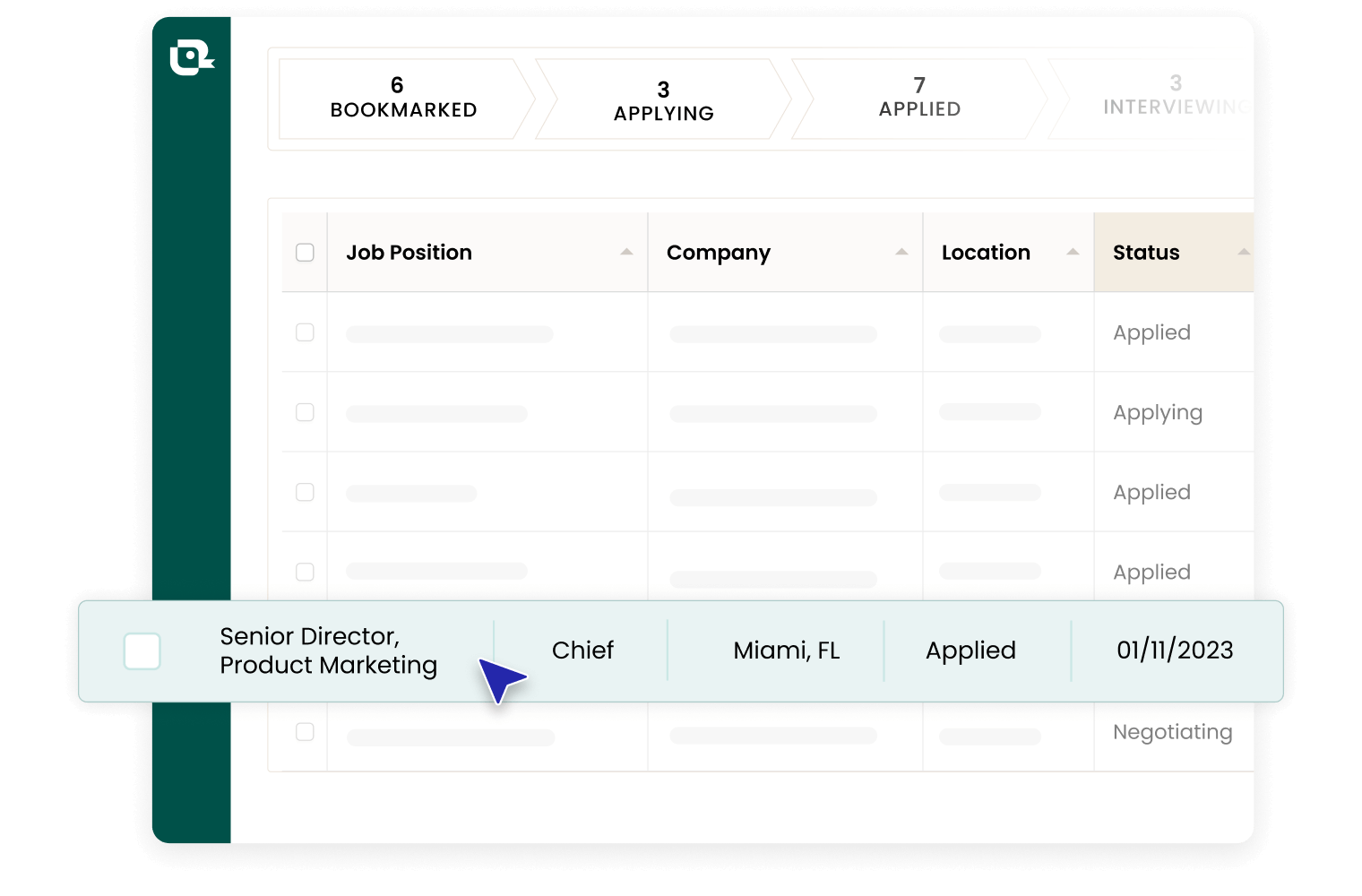
JavaScript Developer Interview Questions and Answers

"What are JavaScript closures and how would you use them?"
This question tests your understanding of a fundamental JavaScript concept that is crucial for managing scope in applications.
How to Answer It
Explain what closures are, why they are important, and provide an example of a practical use case where a closure might be beneficial.
Example Answer
"Closures are functions that have access to the outer (enclosing) function’s variables even after the outer function has returned. They are important for data encapsulation and preserving state across asynchronous operations. For instance, closures are useful when creating factory functions that can configure and return customized functions for different clients, maintaining private state for each instance."

"Can you explain the difference between '==' and '===' in JavaScript?"
This question assesses your knowledge of JavaScript's type coercion and equality comparison rules.
How to Answer It
Describe the technical difference between the two operators and when you would use one over the other.
Example Answer
"The '==' operator tests for abstract equality and will perform type coercion if the values being compared are not of the same type. On the other hand, '===' tests for strict equality without type coercion. I use '===' to avoid unexpected results due to type coercion, ensuring that the values being compared are of the same type and value."

"How do you handle asynchronous code in JavaScript?"
This question evaluates your understanding of asynchronous programming patterns in JavaScript.
How to Answer It
Discuss different approaches like callbacks, promises, and async/await, and explain when and why you would use each method.
Example Answer
"In JavaScript, asynchronous code can be handled using callbacks, promises, or async/await. Callbacks are the traditional method but can lead to 'callback hell.' Promises are more readable and chainable. Async/await is the most recent addition, allowing us to write asynchronous code in a synchronous manner. In a recent project, I used async/await to handle API calls, which improved the readability and maintainability of the code."

"What is event delegation in JavaScript and why is it useful?"
This question probes your knowledge of DOM events and event handling patterns.
How to Answer It
Explain the concept of event delegation and its benefits, particularly in the context of dynamic content or performance optimization.
Example Answer
"Event delegation is a technique where a single event listener is attached to a parent element rather than individual child elements. It leverages the event bubbling phase to handle events at a higher level. This is useful for dynamically added elements, as it avoids the need to attach event listeners to each child. It also improves performance by reducing the number of event listeners. For example, in a to-do list application, I used event delegation on the list container to manage click events for all list items."

"How do you ensure your JavaScript code is performant and efficient?"
This question assesses your ability to write optimized code and your knowledge of performance best practices.
How to Answer It
Discuss specific strategies and tools you use to measure and improve JavaScript performance.
Example Answer
"To ensure my JavaScript code is performant, I focus on optimizing loops, reducing DOM manipulations, and using efficient algorithms. I also leverage browser dev tools and performance profiling to identify bottlenecks. For instance, in a recent project, I used web workers for computationally intensive tasks to avoid blocking the main thread, which significantly improved the responsiveness of the application."

"Explain the concept of prototypal inheritance in JavaScript."
This question tests your understanding of JavaScript's object-oriented features and inheritance model.
How to Answer It
Describe what prototypal inheritance is and how it differs from classical inheritance. Provide an example to illustrate your explanation.
Example Answer
"Prototypal inheritance is a feature in JavaScript where objects can inherit properties and methods from other objects, known as prototypes. Unlike classical inheritance, where classes inherit from other classes, JavaScript uses a prototype chain. For example, when creating a new object using the 'Object.create()' method, the newly created object inherits from the specified prototype object."

"What are JavaScript modules and how do they improve code organization?"
This question evaluates your understanding of code modularity and structure in JavaScript.
How to Answer It
Explain the concept of modules, their syntax, and their benefits for maintaining a clean codebase.
Example Answer
"JavaScript modules are individual pieces of code that encapsulate specific functionality and can be exported and imported where needed. They improve code organization by breaking code into smaller, reusable components. This enhances maintainability and scalability. For example, in my last project, I organized utility functions into separate modules, which simplified testing and allowed for easy reuse across the application."

"How do you handle cross-browser compatibility issues in JavaScript?"
This question checks your experience with and strategies for dealing with browser inconsistencies.
How to Answer It
Discuss the tools and techniques you use to ensure that your JavaScript code works across different browsers.
Example Answer
"To handle cross-browser compatibility issues, I use feature detection libraries like Modernizr to gracefully fallback when a feature is not supported. I also rely on transpilers like Babel to convert modern JavaScript into a form that's compatible with older browsers. Additionally, I conduct thorough testing with tools like BrowserStack to ensure consistent behavior across various browsers and devices. For example, in a recent project, I used polyfills to provide equivalent functionality for promises in browsers that did not natively support them."Which Questions Should You Ask in a JavaScript Developer Interview?
In the dynamic world of JavaScript development, the questions you ask in an interview are as crucial as the technical expertise you bring to the table. They serve a dual purpose: showcasing your depth of knowledge and genuine interest in the role, while also providing you with essential insights into the company's culture, practices, and expectations. As a JavaScript Developer, the inquiries you make can reflect your technical acumen, your eagerness to engage with the team, and your strategic thinking about code and collaboration. By asking informed questions, you not only position yourself as a thoughtful candidate but also take an active role in determining whether the opportunity aligns with your career objectives and personal values.
Good Questions to Ask the Interviewer
"Can you describe the current JavaScript frameworks and libraries the team primarily uses, and what led to those choices?"
This question demonstrates your technical knowledge and interest in the company's tech stack. It also gives you insight into the company's decision-making process and how adaptable or open they are to new technologies.
"How does the development team handle code reviews, and what is the process for ensuring code quality?"
Asking about code reviews and quality assurance processes shows that you care about writing maintainable, high-quality code. It also helps you understand the collaborative dynamics of the team and the emphasis they place on best practices.
"What opportunities are there for professional development and continuing education in JavaScript and related technologies?"
This question indicates your desire to grow and stay current in your field. It also allows you to gauge the company's commitment to investing in their employees' skills and career advancement.
"Could you share a recent challenge the development team faced with a project and how it was resolved?"
Inquiring about challenges and their resolutions reveals your problem-solving interest and helps you assess the team's approach to overcoming obstacles. It also provides a realistic view of the types of issues you might encounter and the support you can expect.
What Does a Good JavaScript Developer Candidate Look Like?
In the realm of web development, a proficient JavaScript developer is a linchpin in creating dynamic and interactive user experiences. Employers and hiring managers are on the lookout for candidates who not only have a firm grasp of JavaScript fundamentals but also exhibit a passion for technology, a knack for problem-solving, and the ability to adapt to new frameworks and libraries. A good JavaScript developer candidate is someone who can write clean, efficient code and also possesses a strong understanding of web performance, accessibility, and security considerations. They are expected to collaborate effectively with team members from various disciplines, bringing a project from conception to completion with a user-centric mindset.
Technical Proficiency
A strong candidate must demonstrate deep knowledge of JavaScript, including ES6+ features, and the ability to write high-quality, maintainable code. They should also be familiar with popular frameworks and libraries like React, Angular, or Vue.js.
Understanding of Web Fundamentals
It's essential for a JavaScript developer to have a solid understanding of HTML, CSS, and core web technologies. This includes responsive design principles, accessibility standards, and best practices for web performance and SEO.
Problem-Solving Skills
Good JavaScript developers are excellent problem solvers with an analytical mindset. They should be able to debug effectively and optimize code for efficiency and scalability.
Adaptability and Continuous Learning
The JavaScript ecosystem is constantly evolving, so a willingness to learn and adapt to new tools and technologies is crucial. A candidate should show a track record of continuous learning and staying up-to-date with industry trends.
Collaborative Spirit
JavaScript developers often work in teams, so strong communication skills and the ability to work collaboratively with other developers, designers, and stakeholders are vital. This includes experience with version control systems like Git.
Understanding of Testing and Debugging
A proficient JavaScript developer should be familiar with testing frameworks and methodologies. They must be able to write unit tests, understand test automation, and perform thorough debugging to ensure code quality.
Attention to User Experience
Developers need to be mindful of the end user's experience. This means writing code that not only functions well but also creates an intuitive and engaging interface for the user.
By embodying these qualities, a JavaScript developer candidate can demonstrate their readiness to tackle the challenges of modern web development and make a significant impact on any project they are a part of.
Interview FAQs for JavaScript Developers
What is the most common interview question for JavaScript Developers?
"What are closures in JavaScript, and how do you use them?" This question probes your understanding of scope and data encapsulation. A compelling answer should illustrate your grasp of maintaining state in asynchronous code and creating private variables. Demonstrate your skill by explaining closures' ability to access outer function scopes from an inner function, and provide a practical example, such as implementing a factory function or data privacy in a module pattern.
What's the best way to discuss past failures or challenges in a JavaScript Developer interview?
To demonstrate problem-solving skills in a JavaScript Developer interview, detail a complex coding challenge you faced. Explain your systematic debugging process, how you broke down the problem into manageable parts, and the innovative coding solutions you implemented. Highlight your use of JavaScript-specific tools or frameworks, and emphasize the positive outcomes, such as performance improvements or enhanced user experience, to illustrate the effectiveness of your problem-solving approach.
How can I effectively showcase problem-solving skills in a JavaScript Developer interview?
To demonstrate problem-solving skills in a JavaScript Developer interview, detail a complex coding challenge you faced. Explain your systematic debugging process, how you broke down the problem into manageable parts, and the innovative coding solutions you implemented. Highlight your use of JavaScript-specific tools or frameworks, and emphasize the positive outcomes, such as performance improvements or enhanced user experience, to illustrate the effectiveness of your problem-solving approach.
Up Next
JavaScript Developer Job Title Guide
Copy Goes Here.
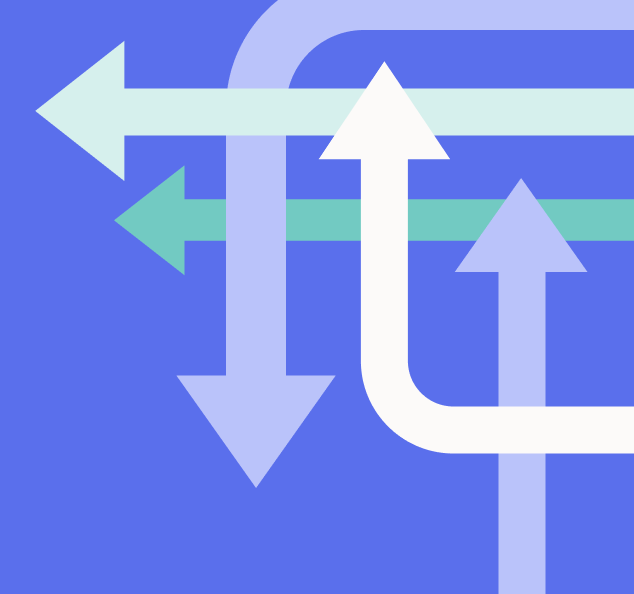