Interviewing as a Angular Developer
Navigating the path to becoming an Angular Developer is akin to mastering a complex web of dynamic coding challenges and architectural decisions. In the crucible of interviews, your technical prowess, problem-solving skills, and understanding of Angular's core principles are put to the test.
This guide is meticulously crafted to arm you with insights into the questions that shape Angular Developer interviews. We'll dissect the intricacies of technical queries, the significance of behavioral questions, and the expectations behind coding tasks. You'll gain clarity on what interviewers seek in a standout candidate and learn how to showcase your expertise effectively. Whether you're preparing for your first interview or looking to refine your approach, this guide is your strategic companion to excel in the Angular Developer interview landscape and elevate your career trajectory.
Types of Questions to Expect in a Angular Developer Interview
Angular Developer interviews are designed to probe not only your technical expertise but also your problem-solving abilities and alignment with the development team's culture and practices. Recognizing the different types of questions you may encounter can help you prepare more effectively and demonstrate your full potential as a candidate. Here's an overview of the question categories that are commonly featured in Angular Developer interviews.
Core Angular Concepts
Questions in this category will test your understanding of Angular's fundamental principles and features. Expect to discuss topics such as components, services, directives, modules, and data binding. These questions assess your technical knowledge and experience with the framework, ensuring you have a solid foundation to build upon.
Programming and Coding Challenges
To evaluate your hands-on coding skills, you'll likely face live coding exercises or take-home assignments. These challenges can range from simple syntax questions to complex algorithmic problems that require you to write code on the spot or explain your approach to a given solution. They serve to assess your proficiency in TypeScript, HTML, CSS, and Angular-specific coding practices.
Behavioral Questions
These questions delve into your past experiences and how you approach various situations in a professional setting. You might be asked about your most challenging project, how you handle tight deadlines, or conflict resolution within a team. The goal is to understand your soft skills, such as communication, teamwork, and adaptability, which are crucial for a collaborative environment.
System Design and Architecture
Expect to discuss how you would structure an application or solve architectural challenges using Angular. These questions test your ability to design scalable, maintainable, and efficient systems. You'll need to demonstrate your understanding of best practices, design patterns, and how to make decisions that impact the overall application architecture.
Testing and Debugging
Angular developers must ensure their code is reliable and bug-free. Questions in this area will focus on your familiarity with testing frameworks like Jasmine and Karma, and your approach to writing unit tests, integration tests, and end-to-end tests. You may also be asked about your strategies for debugging and troubleshooting issues within an Angular application.
State Management and Data Flow
In this section, interviewers are interested in your knowledge of managing state within an Angular application. You might be asked about your experience with libraries like NgRx or RxJS, and how you handle data flow and asynchronous operations. These questions assess your ability to manage complex application states and create a seamless user experience.
By understanding these question types and preparing your responses, you can showcase your Angular expertise and problem-solving skills effectively. Tailoring your study and practice to these categories will help you enter the interview with confidence and a clear idea of what to expect.
Preparing for a Angular Developer Interview
Preparing for an Angular Developer interview is a critical step in showcasing your technical prowess and passion for front-end development. It's not just about proving your coding skills; it's about demonstrating a deep understanding of the Angular framework, its ecosystem, and how it fits within the broader context of web development. A well-prepared candidate can effectively communicate their knowledge, experience, and problem-solving abilities, making a strong impression on potential employers. By investing time in preparation, you not only increase your confidence but also demonstrate your commitment to excellence in your craft.
How to do Interview Prep as an Angular Developer
- Master the Core Concepts: Ensure you have a solid grasp of Angular's core concepts such as modules, components, services, directives, dependency injection, and data binding. Understanding these will help you answer technical questions with confidence.
- Stay Updated with Angular: Angular is constantly evolving, so make sure you're familiar with the latest version and its features. Be prepared to discuss updates, improvements, and any deprecated features.
- Review Your Past Projects: Be ready to talk about your previous work with Angular. Highlight specific challenges you faced, how you overcame them, and what you learned from those experiences.
- Understand Related Technologies: Angular doesn't exist in a vacuum. Brush up on related technologies like TypeScript, RxJS, and state management libraries (such as NgRx or Akita) that are often used in conjunction with Angular.
- Practice Coding Challenges: Sharpen your coding skills with challenges and exercises, especially those that involve common Angular scenarios. This will help you think on your feet during the technical portion of the interview.
- Know the Testing Tools: Be familiar with testing frameworks and tools like Jasmine, Karma, and Protractor, and be able to discuss how you test your Angular applications.
- Prepare for Behavioral Questions: Reflect on your experiences working in teams, handling tight deadlines, and managing conflicts. Employers often look for developers who are not only technically proficient but also fit well within their team culture.
- Explore the Company's Tech Stack: Research the company's technology stack and any specific Angular practices they might use. This shows your interest in the company and can help you tailor your responses to their context.
- Develop Insightful Questions: Prepare thoughtful questions about the company's development processes, project methodologies, and expectations for the role. This demonstrates your proactive approach and genuine interest in the position.
- Conduct Mock Interviews: Practice with peers or mentors to get feedback on your responses and to refine your communication skills. This will help you become more articulate and reduce interview anxiety.
By following these steps, you'll be able to enter your Angular Developer interview with the knowledge, skills, and confidence needed to stand out from the competition. Remember, the goal is not just to answer questions, but to engage in a dialogue that positions you as a valuable asset to the team.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free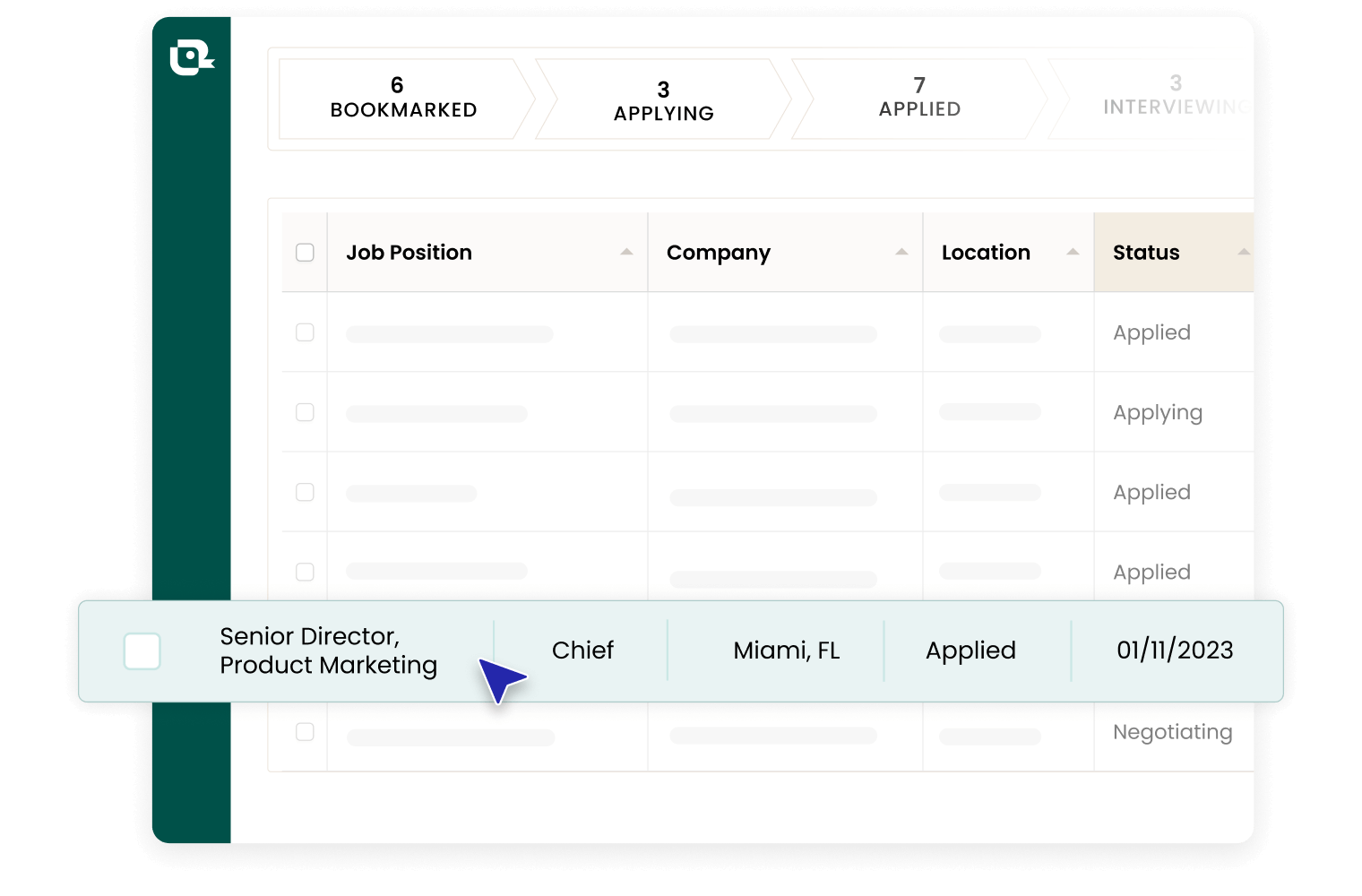
Angular Developer Interview Questions and Answers

"What are the core concepts of Angular, and how do they improve front-end development?"
This question assesses your understanding of Angular's fundamental principles and your ability to articulate the advantages they bring to front-end development.
How to Answer It
Discuss the key concepts such as modules, components, services, data binding, directives, and dependency injection. Explain how these concepts contribute to a structured and maintainable codebase.
Example Answer
"Angular's core concepts like modularity, components, and services help in creating a clear project structure, which makes it easier to maintain and scale. For instance, components promote reusability, while services ensure a separation of concerns. Data binding and directives make the UI dynamic and responsive without the need for additional scripting. Dependency injection simplifies the way objects are managed and allows for more testable code."

"How do you manage state in an Angular application?"
This question evaluates your knowledge of state management patterns and practices in Angular applications.
How to Answer It
Discuss the different strategies for managing state, such as using services, RxJS Observables, or state management libraries like NgRx or Akita. Mention the pros and cons of each approach.
Example Answer
"In my previous projects, I've used RxJS Observables for managing state in smaller applications due to their simplicity and the reactive programming paradigm they offer. For larger, more complex applications, I've implemented NgRx, which provides a robust and predictable state management solution following the Redux pattern. This approach greatly simplifies state synchronization across components and makes the application easier to debug."

"Can you explain the Angular lifecycle hooks and how you've utilized them in your projects?"
This question probes your understanding of the component lifecycle in Angular and your practical experience in leveraging lifecycle hooks.
How to Answer It
Describe the lifecycle hooks like OnInit, OnChanges, OnDestroy, etc., and provide examples of how you've used them to perform tasks at specific timings in a component's life.
Example Answer
"In my last project, I used OnInit to fetch initial data required for the component, ensuring that the data is available as soon as the component is initialized. I used OnChanges to respond to changes in input properties, which allowed for dynamic updates to the component. OnDestroy was crucial for unsubscribing from Observables and preventing memory leaks when the component was destroyed."

"How do you ensure your Angular applications are performant and efficient?"
This question assesses your ability to optimize Angular applications for performance.
How to Answer It
Discuss performance optimization techniques such as change detection strategies, lazy loading, trackBy function in ngFor, and ahead-of-time (AOT) compilation.
Example Answer
"To ensure performance, I implement lazy loading to load feature modules on demand, reducing the initial load time. I use the OnPush change detection strategy to minimize unnecessary checks, and I leverage the trackBy function to optimize list rendering. Additionally, I always use AOT compilation for production builds to decrease the application's load time and improve runtime performance."

"Describe your experience with Angular's form handling capabilities."
This question gauges your familiarity with Angular's forms API and your ability to handle complex form interactions.
How to Answer It
Explain the differences between reactive and template-driven forms, and discuss your experience with form validation, dynamic form controls, and form submission handling.
Example Answer
"In my experience, reactive forms offer more flexibility and are easier to unit test, so I prefer them for complex scenarios. I've implemented custom validators to ensure data integrity and used dynamic form controls to build forms that adapt to user input. For form submissions, I've integrated with backend APIs, handling both success and error states gracefully."

"How do you handle security concerns in Angular applications?"
This question explores your knowledge of security best practices in Angular development.
How to Answer It
Discuss common security concerns such as cross-site scripting (XSS) and how Angular addresses them. Mention strategies like using the built-in DOM sanitization and implementing secure HTTP calls.
Example Answer
"Angular has built-in protection against XSS through DOM sanitization, which automatically escapes untrusted values. I ensure that all user input is validated both client-side and server-side. For HTTP interactions, I use HTTPS and implement token-based authentication to secure API calls. I also keep Angular and its dependencies up to date to protect against known vulnerabilities."

"Explain how you would optimize an Angular application for SEO."
This question checks your understanding of search engine optimization in the context of Angular applications, which are typically single-page applications (SPAs).
How to Answer It
Discuss server-side rendering with Angular Universal and other techniques like meta tags manipulation and pre-rendering for improving SEO.
Example Answer
"To optimize for SEO, I use Angular Universal for server-side rendering, which allows search engines to crawl the application more effectively. I also dynamically update meta tags and provide meaningful URL structures with the Angular Router. For static pages, I implement pre-rendering to serve quickly loaded content to search engines."

"How do you approach testing in Angular applications?"
This question evaluates your experience with testing strategies and tools in Angular.
How to Answer It
Explain the types of tests you write (unit, integration, end-to-end) and the tools you use (Jasmine, Karma, Protractor). Discuss how you ensure code quality and application reliability through testing.
Example Answer
"I follow a test-driven development approach, writing Jasmine unit tests alongside my code to ensure each component and service functions correctly. I use Karma as a test runner for executing the tests in different browsers. For end-to-end testing, I've used Protractor to simulate user interactions and verify the integrated functionality of the application. This comprehensive testing strategy helps me catch issues early and maintain high code quality."Which Questions Should You Ask in a Angular Developer Interview?
In the dynamic world of Angular development, the questions you ask in an interview are as crucial as the technical expertise you bring to the table. They serve a dual purpose: showcasing your depth of knowledge and genuine interest in Angular, and also ensuring the role aligns with your career trajectory and personal values. For Angular Developers, the inquiries made can reflect your understanding of the framework, your foresight in technological trends, and your potential fit within the team. By asking insightful questions, you not only leave a lasting impression but also critically evaluate if the opportunity is conducive to your professional growth and aspirations.
Good Questions to Ask the Interviewer
"Can you describe the current project architecture and the decision-making process behind the chosen technologies?"
This question demonstrates your technical curiosity and eagerness to understand the rationale behind the project's technical decisions. It also gives you insight into the company's tech stack and how open they are to adopting new technologies or methodologies.
"How does the team stay updated with the latest Angular versions and handle migrations?"
Staying current with Angular's frequent updates is essential. This question shows your commitment to best practices and continuous learning, while also giving you an idea of the company's dedication to maintaining modern development standards.
"What are the most significant challenges the development team has faced with Angular, and how were they overcome?"
Understanding the hurdles faced by the team can help you gauge the complexity of problems you'll be solving. It also highlights the team's problem-solving abilities and whether they work collaboratively to tackle technical challenges.
"Could you explain the team's workflow and how Angular developers collaborate with other roles in the company?"
This question allows you to understand the dynamics between different departments and how integrated the development process is. It also shows your interest in teamwork and your ability to fit into a cross-functional team environment.
What Does a Good Angular Developer Candidate Look Like?
In the realm of web development, Angular developers play a pivotal role in crafting dynamic and responsive applications. A good Angular developer candidate is not only proficient in technical skills but also exhibits a deep understanding of user experience, performance optimization, and clean code principles. Employers and hiring managers seek individuals who can blend technical prowess with a strategic approach to application development, ensuring that the end product is both efficient and aligned with business objectives.
A strong Angular developer candidate is someone who is well-versed in modern web development practices and can effectively utilize Angular's robust framework to deliver scalable and maintainable applications. They must be problem solvers who can anticipate and address technical challenges, while also communicating effectively with team members and stakeholders.
Technical Expertise in Angular and Related Technologies
A good candidate has a strong command of Angular and its ecosystem, including familiarity with TypeScript, RxJS, and Angular CLI. They should understand core concepts such as components, services, and modules, as well as advanced features like lazy loading and Angular Universal for server-side rendering.
Understanding of Web Fundamentals
Proficiency in HTML, CSS, and JavaScript is essential. The candidate should also be knowledgeable about responsive design principles and accessibility standards to ensure that applications are user-friendly and inclusive.
Proficiency in Application Architecture
The ability to design and implement scalable and maintainable application architectures is crucial. This includes understanding state management, implementing clean code practices, and ensuring that the application is structured in a way that facilitates easy updates and feature additions.
Performance Optimization
A strong candidate is skilled in optimizing application performance, which includes techniques like change detection strategies, ahead-of-time compilation, and tree shaking to reduce load times and improve the user experience.
Testing and Debugging
Candidates should demonstrate expertise in testing frameworks such as Jasmine and Karma, and be able to write unit and end-to-end tests to ensure application stability and reliability. Debugging skills are also vital for quickly resolving issues that arise.
Version Control and Collaboration
Experience with version control systems like Git is important for collaborative development. The candidate should be comfortable working in a team environment, merging code, resolving conflicts, and contributing to a shared codebase.
Continuous Learning and Adaptability
The technology landscape is ever-evolving, and a good Angular developer must be committed to continuous learning. They should stay updated with the latest Angular versions and web development trends, and be adaptable to new tools and methodologies.
By embodying these qualities and skills, an Angular developer candidate can demonstrate their readiness to contribute to a team and deliver high-quality applications that meet both user needs and business goals. Hiring managers and recruiters will look for these attributes to identify candidates who can thrive in a fast-paced development environment and add value to their organization.
Interview FAQs for Angular Developers
What is the most common interview question for Angular Developers?
"How do you manage state in Angular applications?" This question probes your architectural understanding and problem-solving skills. A comprehensive answer should highlight your familiarity with core concepts and tools like services, RxJS Observables, or NgRx, and how you apply them to maintain a predictable state, handle side effects, and optimize performance. It's essential to articulate a clear strategy that demonstrates your ability to design scalable and maintainable state management solutions.
What's the best way to discuss past failures or challenges in a Angular Developer interview?
To demonstrate problem-solving skills in an Angular Developer interview, detail a complex coding challenge you faced. Explain your systematic debugging process, the innovative coding techniques or design patterns you employed, and how you optimized application performance or user experience. Highlight collaboration with team members, incorporation of user feedback, and the successful resolution's impact on the project, showcasing your technical acumen and solution-oriented mindset.
How can I effectively showcase problem-solving skills in a Angular Developer interview?
To demonstrate problem-solving skills in an Angular Developer interview, detail a complex coding challenge you faced. Explain your systematic debugging process, the innovative coding techniques or design patterns you employed, and how you optimized application performance or user experience. Highlight collaboration with team members, incorporation of user feedback, and the successful resolution's impact on the project, showcasing your technical acumen and solution-oriented mindset.
Up Next
Angular Developer Job Title Guide
Copy Goes Here.
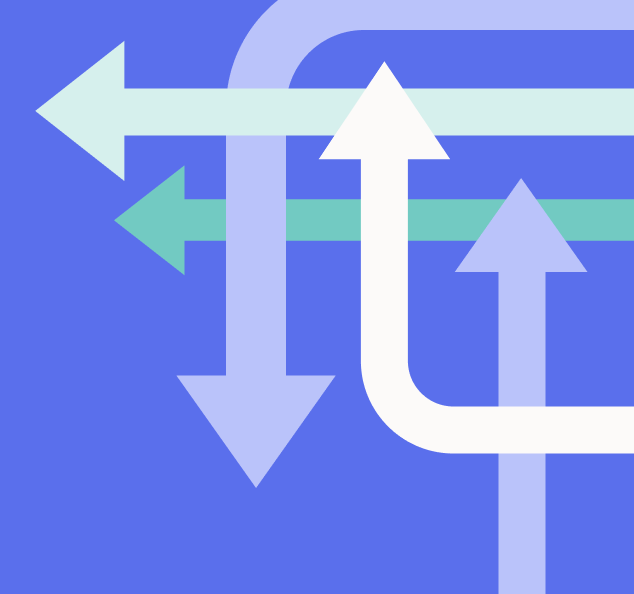