Interviewing as a React Developer
Navigating the interview process as a React Developer is akin to demonstrating your expertise in a language that's constantly evolving. With React's prominence in building dynamic user interfaces, interviews for React Developers are designed to assess not only your technical prowess but also your ability to adapt and innovate within this versatile ecosystem.
In this guide, we will dissect the array of questions you might encounter, from intricate coding challenges to conceptual queries that probe your understanding of React's core principles. We'll provide insights into crafting articulate responses that showcase your problem-solving skills and your fluency in React's latest features. By equipping you with knowledge of what a 'good' React Developer looks like to employers, and the questions to ask to align with your career aspirations, this guide is your comprehensive companion for excelling in your upcoming interviews.
Types of Questions to Expect in a React Developer Interview
React Developer interviews are designed to probe not only your technical knowledge but also your problem-solving abilities, teamwork, and adaptability to change. Like any specialized role, there are certain question types that are particularly relevant to React Developers. These questions help interviewers assess a candidate's depth of understanding, practical experience, and approach to common challenges faced in the field. Here's an overview of the types of questions you should be prepared to answer.
Core React Concepts
Questions in this category will test your understanding of fundamental React concepts. Expect to answer questions about JSX, components, state, props, lifecycle methods, hooks, and the virtual DOM. These questions are designed to gauge your foundational knowledge and your ability to apply React principles in building efficient, dynamic user interfaces.
State Management and Data Flow
State management is crucial in React applications, and you will likely encounter questions about handling application state. This could involve discussions on useState, useReducer, context API, and external libraries like Redux or MobX. Interviewers want to see your approach to state management and how you ensure smooth data flow between components.
Performance Optimization
React Developers must be adept at optimizing application performance. Questions may delve into topics such as shouldComponentUpdate, PureComponent, React.memo, lazy loading, code splitting, and using the Profiler API. These questions assess your ability to write high-performance applications and your knowledge of React's performance optimization techniques.
Testing and Debugging
You should be ready to discuss your experience with testing and debugging React applications. Questions could cover unit testing, integration testing, end-to-end testing, and the tools you use, such as Jest, Enzyme, or React Testing Library. Interviewers are looking for your proficiency in ensuring code quality and your methodology for tracking down and fixing bugs.
Project and Code Structure
Interviewers may ask about your preferred project structure, component organization, and best practices for writing clean, maintainable code. These questions aim to understand your approach to code organization and your ability to work within a team where code readability and consistency are important.
Real-world Problem-solving
Expect to be given hypothetical scenarios or actual problems that require you to think on your feet. You might be asked to design a component on a whiteboard, refactor a piece of code, or discuss how you would handle a specific challenge in a React project. These questions test your practical skills and how you apply React knowledge to solve real-world issues.
Soft Skills and Cultural Fit
Lastly, React Developer interviews often include questions about teamwork, communication, and your ability to adapt to the company's culture. You might be asked about past team experiences, how you handle conflict, or your willingness to learn new technologies. These questions are intended to determine if you'll be a good fit for the team and the company as a whole.
Understanding these question types and preparing thoughtful, articulate responses can greatly improve your chances of success in a React Developer interview. It's not just about showing what you know, but also demonstrating how you think, collaborate, and contribute to a project's success.
Preparing for a React Developer Interview
Preparing for a React Developer interview is a critical step in showcasing your technical prowess and your passion for front-end development. It's not just about proving your coding skills; it's about demonstrating a deep understanding of React principles, best practices, and the ability to solve real-world problems. A well-prepared candidate can articulate how their experience and knowledge align with the needs of the role and the company's tech stack. By investing time in preparation, you signal your professionalism and commitment to the role, setting the stage for a successful interview.
How to do Interview Prep as a React Developer
- Brush Up on React Fundamentals: Ensure you have a strong grasp of React's core concepts, such as JSX, components, state, props, and the component lifecycle. Be prepared to discuss how these concepts work together to create efficient, dynamic user interfaces.
- Understand State Management: Be familiar with state management in React and the differences between local component state, Context API, and external libraries like Redux or MobX. Be ready to discuss scenarios where you might choose one approach over another.
- Review Hooks and New Features: Since the introduction of hooks, functional components have become more prevalent. Make sure you understand hooks like useState, useEffect, and custom hooks. Also, stay updated on the latest features released in recent React versions.
- Practice Building Components: Hands-on practice is invaluable. Build reusable components and small applications to reinforce your understanding of React patterns and practices. This will also give you concrete examples to discuss during your interview.
- Explore the React Ecosystem: Familiarize yourself with important tools and libraries commonly used with React, such as React Router for routing, Axios or Fetch for HTTP requests, and testing libraries like Jest and React Testing Library.
- Understand Performance Optimization: Be prepared to talk about performance in React applications. Understand concepts like memoization, lazy loading, virtualization, and how to use React's built-in tools like React.memo, useCallback, and useMemo to optimize performance.
- Prepare for Behavioral Questions: Reflect on your past experiences working with React and be ready to discuss challenges you've faced, how you've collaborated with team members, and projects you're particularly proud of.
- Review the Company's Tech Stack: Research the company's technology stack and any specific React practices they might use. This will help you tailor your responses to show how your skills and experience align with their needs.
- Prepare Your Own Questions: Develop thoughtful questions about the company's development processes, culture, and expectations for the role. This demonstrates your genuine interest in the position and the company.
- Mock Interviews: Practice with mock interviews focusing on technical questions, coding exercises, and system design. Use platforms like Pramp or LeetCode, or practice with a peer to simulate the interview experience.
By following these steps, you'll be able to enter your React Developer interview with confidence, equipped with the knowledge and experience to impress your potential employers and secure the role you're aiming for.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free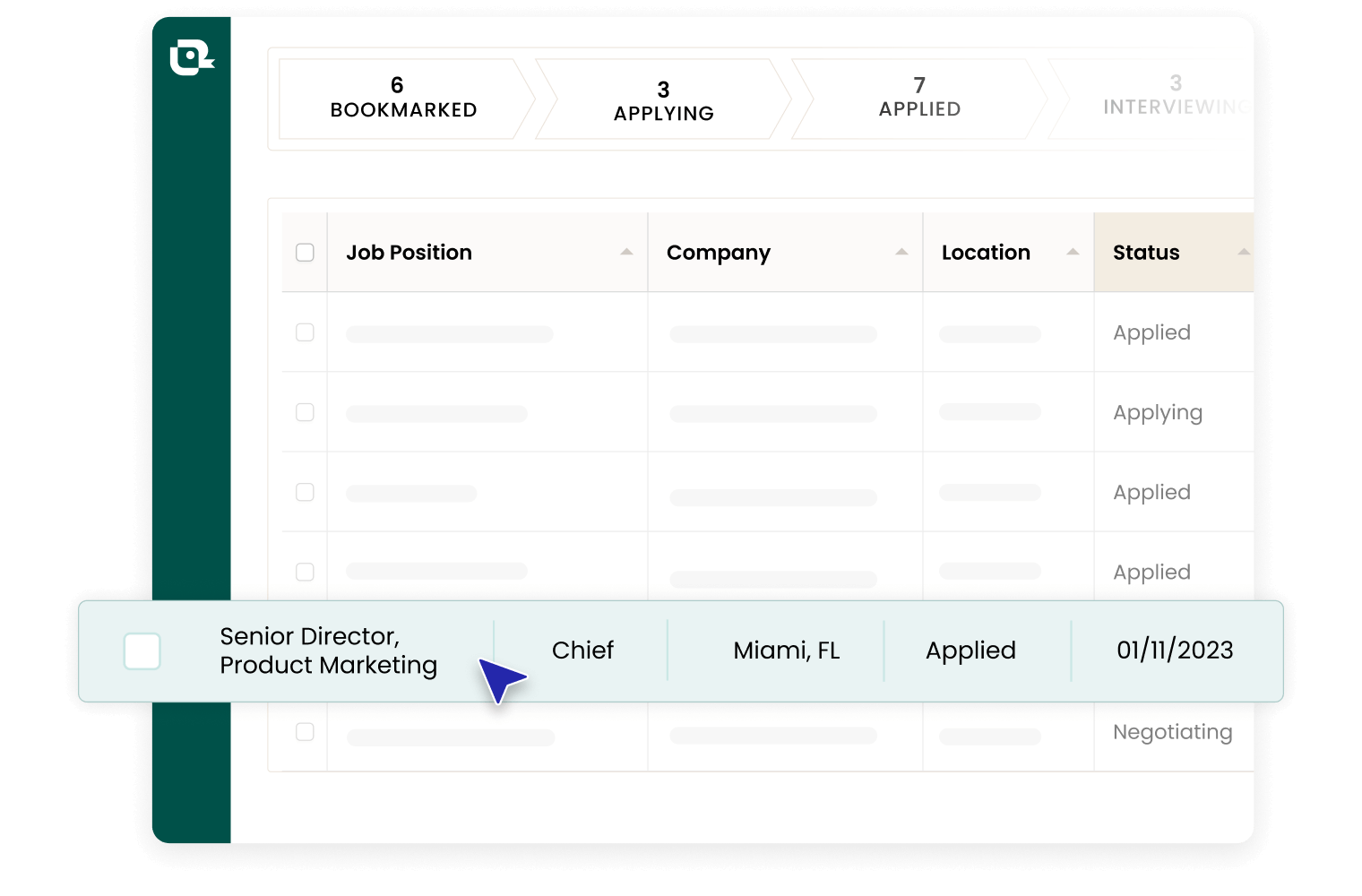
React Developer Interview Questions and Answers

"Can you explain the virtual DOM and its benefits in React?"
This question assesses your understanding of core React concepts and your ability to articulate technical advantages in a simple manner.
How to Answer It
Discuss what the virtual DOM is, how it differs from the real DOM, and why it's beneficial for performance and efficiency in React applications.
Example Answer
"The virtual DOM is a lightweight copy of the actual DOM. It allows React to perform diffing algorithms to detect changes and update only those elements in the real DOM that have actually changed, rather than re-rendering the entire DOM tree. This results in improved performance and a smoother user experience, especially for complex applications."

"How do you manage state in React components?"
This question evaluates your knowledge of state management and your ability to choose the right approach for different scenarios.
How to Answer It
Explain the concept of state in React and the various ways to manage it, including the useState hook, Redux, or the Context API.
Example Answer
"In React, state refers to the data that determines the rendering and behavior of components. For local state management, I use the useState hook. For global state or more complex scenarios, I prefer using Redux for its predictable state container or the Context API for passing data without prop drilling."

"What are lifecycle methods in React, and can you give an example of how you've used one?"
This question probes your understanding of component lifecycle and your practical experience in utilizing lifecycle methods.
How to Answer It
Describe the component lifecycle phases and provide an example of a lifecycle method you've used, explaining why and how you used it.
Example Answer
"Lifecycle methods are hooks that allow us to run code at specific points in a component's life. For example, I've used componentDidMount to fetch data from an API and then set the component's state with that data, ensuring the data is available when the component is first rendered."

"How do you optimize performance in a React application?"
This question tests your ability to identify performance bottlenecks and apply optimization techniques.
How to Answer It
Discuss common performance issues in React apps and the strategies you use to address them, such as memoization, lazy loading, or using PureComponent/React.memo.
Example Answer
"To optimize performance, I analyze components with performance bottlenecks using React Developer Tools. Then, I implement memoization with useCallback or useMemo to prevent unnecessary re-renders. For large lists, I use windowing with react-window. Additionally, I apply code-splitting and lazy loading for components that aren't immediately needed."

"Explain the concept of higher-order components (HOCs) in React."
This question gauges your understanding of advanced React patterns and their practical applications.
How to Answer It
Define HOCs and describe how they can be used to enhance component functionality or reuse logic across components.
Example Answer
"A higher-order component is a function that takes a component and returns a new component with additional properties or logic. I've used HOCs to add common functionality, like analytics tracking or user authentication checks, to several components without duplicating code."

"How do you handle forms in React?"
This question explores your approach to a common task in React development and your familiarity with form handling patterns.
How to Answer It
Explain the methods you use for form handling, such as controlled components or libraries like Formik, and the reasons for choosing one over the other.
Example Answer
"For simple forms, I use controlled components to handle form data through React state. For more complex forms, I prefer using Formik because it simplifies form validation, handles form submission, and manages form state out-of-the-box, which makes the code cleaner and easier to maintain."

"What is React Hooks, and how have they changed component development?"
This question assesses your knowledge of recent React features and their impact on writing functional components.
How to Answer It
Discuss what React Hooks are and how they enable state and other React features in functional components, which were previously only possible in class components.
Example Answer
"React Hooks are functions that let us hook into React state and lifecycle features from functional components. They've made it possible to write fully functional components without classes, leading to simpler code and better reuse of stateful logic. For example, I've used the useState and useEffect hooks to replace the state and lifecycle methods in class components."

"Can you describe the process of lifting state up in React and why it's useful?"
This question tests your understanding of component hierarchy and state management in React applications.
How to Answer It
Explain the concept of lifting state up and provide a scenario where it's beneficial to share state across multiple components.
Example Answer
"Lifting state up involves moving state to a common ancestor of components that need to share the same data. This is useful when multiple components need to access or modify the same state. In a project, I lifted the state up to allow sibling components to be in sync with each other, ensuring a consistent user experience and avoiding prop drilling."Which Questions Should You Ask in a React Developer Interview?
In the competitive field of React development, the questions you ask in an interview can be as telling as the answers you provide. They serve a dual purpose: firstly, they demonstrate your technical insight, engagement, and passion for React development, which can significantly influence the interviewer's perception of you as a candidate. Secondly, they are a strategic tool for you to determine if the role and the company are a good match for your career goals and values. By asking thoughtful and relevant questions, you not only showcase your expertise but also take an active role in assessing the opportunity at hand. This ensures that the position aligns with your professional aspirations and that you are making an informed decision about your future.
Good Questions to Ask the Interviewer
"Can you describe the current React project(s) I would be working on and the team's development methodology?"
This question indicates your eagerness to understand the specifics of what you'll be working on and how the team operates. It also gives you insight into the company's project management style, whether it's Agile, Scrum, or another methodology, and how you would fit into the existing workflow.
"How does the team stay updated with the latest React features and industry best practices?"
Asking this shows your commitment to continuous learning and improvement, which is crucial in the ever-evolving landscape of React development. It also helps you gauge the company's dedication to keeping its technology stack current and its developers well-informed.
"What are the biggest challenges the development team has faced with React, and how were they addressed?"
This question demonstrates your problem-solving mindset and interest in understanding how the company overcomes technical obstacles. It can also reveal the team's approach to troubleshooting and whether they have a proactive or reactive stance on dealing with issues.
"Can you tell me about the opportunities for professional growth and advancement for a React Developer within the company?"
Inquiring about growth prospects shows that you're looking for a long-term opportunity and are interested in how the company supports the career development of its employees. It also allows you to evaluate whether the company's growth opportunities align with your personal career goals.
What Does a Good React Developer Candidate Look Like?
In the realm of web development, a proficient React Developer stands out not only for their technical prowess with the React library but also for their ability to adapt to the evolving landscape of web technologies. Employers and hiring managers are on the lookout for candidates who are not just code-savvy, but who also bring a blend of strategic thinking, problem-solving skills, and effective collaboration to the table. A good React Developer candidate is someone who can build efficient, scalable front-end applications while keeping user experience at the forefront of their design choices. They are expected to be innovative, proactive, and able to contribute to a team's success with a deep understanding of both the technical and user-centric aspects of web development.
Proficient Understanding of React Fundamentals
A strong candidate has a solid grasp of React principles such as JSX, components, state, and props, as well as hooks and the React lifecycle. They should be able to create clean, maintainable code and troubleshoot common issues effectively.
State Management Expertise
Knowledge of state management patterns and libraries (such as Redux or Context API) is essential. The ability to manage and predict application state behaviors is crucial for building robust applications.
Performance Optimization
A good React Developer understands the importance of optimizing application performance, including the use of React's built-in tools and best practices to minimize load times and improve user experience.
Testing and Debugging Skills
Candidates should be adept at writing tests for their code using frameworks like Jest and Enzyme. They should also be skilled in debugging and using developer tools to ensure the reliability and stability of applications.
Modern JavaScript and ES6+ Proficiency
A strong command of modern JavaScript features is expected, as React development relies heavily on ES6+ syntax and concepts such as classes, modules, destructuring, and arrow functions.
Responsive and User-Friendly Design
React Developers must have an eye for design and user experience, ensuring that applications are accessible, responsive, and provide a seamless experience across different devices and browsers.
Version Control and Workflow Experience
Experience with version control systems like Git is essential, as well as familiarity with workflow methodologies such as feature branching and pull requests, which are integral to collaborative development.
Effective Communication and Teamwork
The ability to communicate clearly with team members, stakeholders, and non-technical audiences is key. This includes discussing technical issues, proposing solutions, and articulating the rationale behind development choices.
Continuous Learning and Adaptability
The tech field is constantly evolving, and a good React Developer candidate is one who demonstrates a commitment to continuous learning and the flexibility to adapt to new tools, frameworks, and best practices as they emerge.
Interview FAQs for React Developers
What is the most common interview question for React Developers?
"How do you manage state in React applications?" This question probes your understanding of stateful logic and its impact on user interface behavior. An effective answer should highlight your proficiency with React's built-in state management, context API, and hooks like useState and useReducer, as well as familiarity with external libraries like Redux or MobX, demonstrating a strategic approach to state management based on the complexity and scale of the application.
What's the best way to discuss past failures or challenges in a React Developer interview?
To demonstrate problem-solving skills in a React Developer interview, detail a complex component or application issue you tackled. Explain your debugging process, how you isolated the problem, and the creative coding solutions you implemented. Highlight your use of React-specific tools or patterns, such as hooks or context API, and the positive outcomes, like improved performance or user experience, showing your technical acumen and results-oriented mindset.
How can I effectively showcase problem-solving skills in a React Developer interview?
To demonstrate problem-solving skills in a React Developer interview, detail a complex component or application issue you tackled. Explain your debugging process, how you isolated the problem, and the creative coding solutions you implemented. Highlight your use of React-specific tools or patterns, such as hooks or context API, and the positive outcomes, like improved performance or user experience, showing your technical acumen and results-oriented mindset.
Up Next
React Developer Job Title Guide
Copy Goes Here.
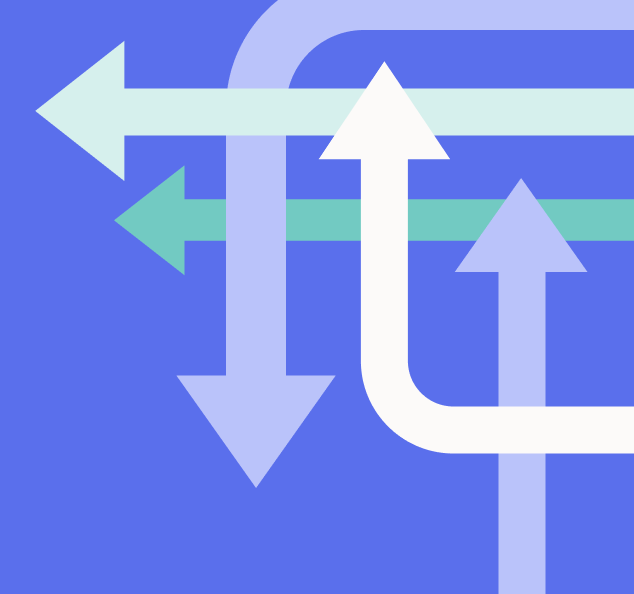