Interviewing as a Python Django Developer
Embarking on the journey to become a Python Django Developer is both exhilarating and demanding, with interviews serving as pivotal milestones in your career path. These interviews are more than mere conversations; they are rigorous evaluations of your technical prowess, problem-solving skills, and understanding of Django's intricacies.
In this guide, we will navigate the landscape of questions that Python Django Developers face, from intricate coding challenges to conceptual queries that probe your depth of knowledge in Django and Python. We'll provide insights into crafting compelling responses, preparing effectively for technical assessments, and the hallmarks of a standout candidate. This resource is meticulously designed to sharpen your interview acumen, ensuring you're equipped to impress potential employers and secure your place in the dynamic field of web development.
Types of Questions to Expect in a Python Django Developer Interview
Python Django Developer interviews are designed to probe not only your technical knowledge but also your problem-solving abilities, your understanding of web development principles, and your capacity to work within a team. Recognizing the different types of questions you may encounter can help you prepare more effectively and demonstrate your full range of skills. Here's an overview of the question categories that are commonly featured in such interviews.
Technical Proficiency Questions
Technical questions form the backbone of any Python Django Developer interview. These questions assess your understanding of Python and the Django framework, including your knowledge of models, views, templates, URL routing, ORM, and middleware. You might be asked to write code on the spot, explain the workings of Django's request-response cycle, or discuss database design principles. These questions test your hands-on experience and your ability to apply Django's features to real-world problems.
Problem-Solving and Algorithm Questions
To gauge your analytical skills, you may be presented with problem-solving or algorithmic challenges. These questions require you to demonstrate your ability to think logically and efficiently. You might be asked to optimize a piece of code, debug an issue, or design an algorithm to solve a specific problem. These questions reveal your critical thinking abilities and your approach to tackling complex coding challenges.
System Design and Architecture Questions
Understanding the bigger picture is crucial for a Python Django Developer. System design questions evaluate your ability to architect scalable and maintainable web applications. You might be asked about RESTful API design, microservices architecture, or how to handle high traffic on a web application. These questions test your architectural knowledge and your capacity to design systems that meet both technical and business requirements.
Behavioral and Situational Questions
Interpersonal skills and cultural fit are just as important as technical prowess. Behavioral questions explore your past experiences, how you've handled team dynamics, or dealt with tight deadlines. Situational questions might place you in a hypothetical scenario to see your response to conflict, collaboration, or project management challenges. These questions assess your soft skills, such as communication, teamwork, and adaptability.
Development Practices and Tooling Questions
A competent Python Django Developer should be familiar with best practices in software development and the tools that support them. You may be asked about version control with Git, testing strategies, or continuous integration/continuous deployment (CI/CD) processes. These questions evaluate your proficiency with the tools and methodologies that ensure code quality, collaboration, and efficient deployment.
By understanding these question types and preparing for each category, you can approach your Python Django Developer interview with confidence. Tailoring your study and practice to these areas will help you showcase the depth and breadth of your expertise, making you a standout candidate for the role.
Preparing for a Python Django Developer Interview
Preparing for a Python Django Developer interview requires a blend of technical expertise, understanding of web development principles, and familiarity with the Django framework. It's not just about proving your coding skills; it's about demonstrating your ability to build efficient, scalable web applications and your knowledge of the entire web development process. A well-prepared candidate can articulate how they've used Django's features in past projects and how they can leverage its capabilities to meet future challenges. This preparation not only conveys your technical proficiency but also your commitment to the role and your passion for web development.
How to do Interview Prep as a Python Django Developer
- Review Django's Documentation: Refresh your knowledge of Django's official documentation, focusing on its ORM, templates, views, URL routing, and forms. Understanding Django's batteries-included features is essential.
- Understand Web Development Concepts: Ensure you have a solid grasp of web development principles, including HTTP, RESTful APIs, MVC architecture, and front-end technologies like HTML, CSS, and JavaScript.
- Practice with Coding Challenges: Sharpen your problem-solving skills with coding challenges, especially those that involve Python and Django. This will help you think critically and articulate your thought process during the interview.
- Brush Up on Python: As Django is a Python-based framework, make sure your Python skills are polished. Be prepared to discuss Python-specific concepts like decorators, generators, and context managers.
- Prepare Your Portfolio: Have a portfolio of your work ready to showcase, including any Django projects you've worked on. Be prepared to discuss the challenges you faced and how you overcame them.
- Understand Deployment and Testing: Be knowledgeable about deploying Django applications and the testing tools available in the framework. Discussing your experience with deployment platforms and continuous integration can be a plus.
- Study Up on Version Control: Be comfortable with version control systems like Git. You may be asked how you've used them in collaboration and to manage code changes in your past projects.
- Prepare for Behavioral Questions: Reflect on your past experiences and be ready to discuss how you've worked in teams, handled conflicts, and stayed motivated during challenging projects.
- Ask Insightful Questions: Develop thoughtful questions about the company's development practices, the projects you'll be working on, and the team you'll be joining. This shows your genuine interest in the role and the company.
- Mock Interviews: Practice with mock interviews to get comfortable with the format and receive feedback. This can help you refine your responses and reduce interview anxiety.
By following these steps, you'll be able to demonstrate not just your technical abilities, but also your comprehensive understanding of web development and your readiness to contribute as a Python Django Developer. This preparation will help you stand out as a knowledgeable and passionate candidate, ready to tackle the challenges of the role.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free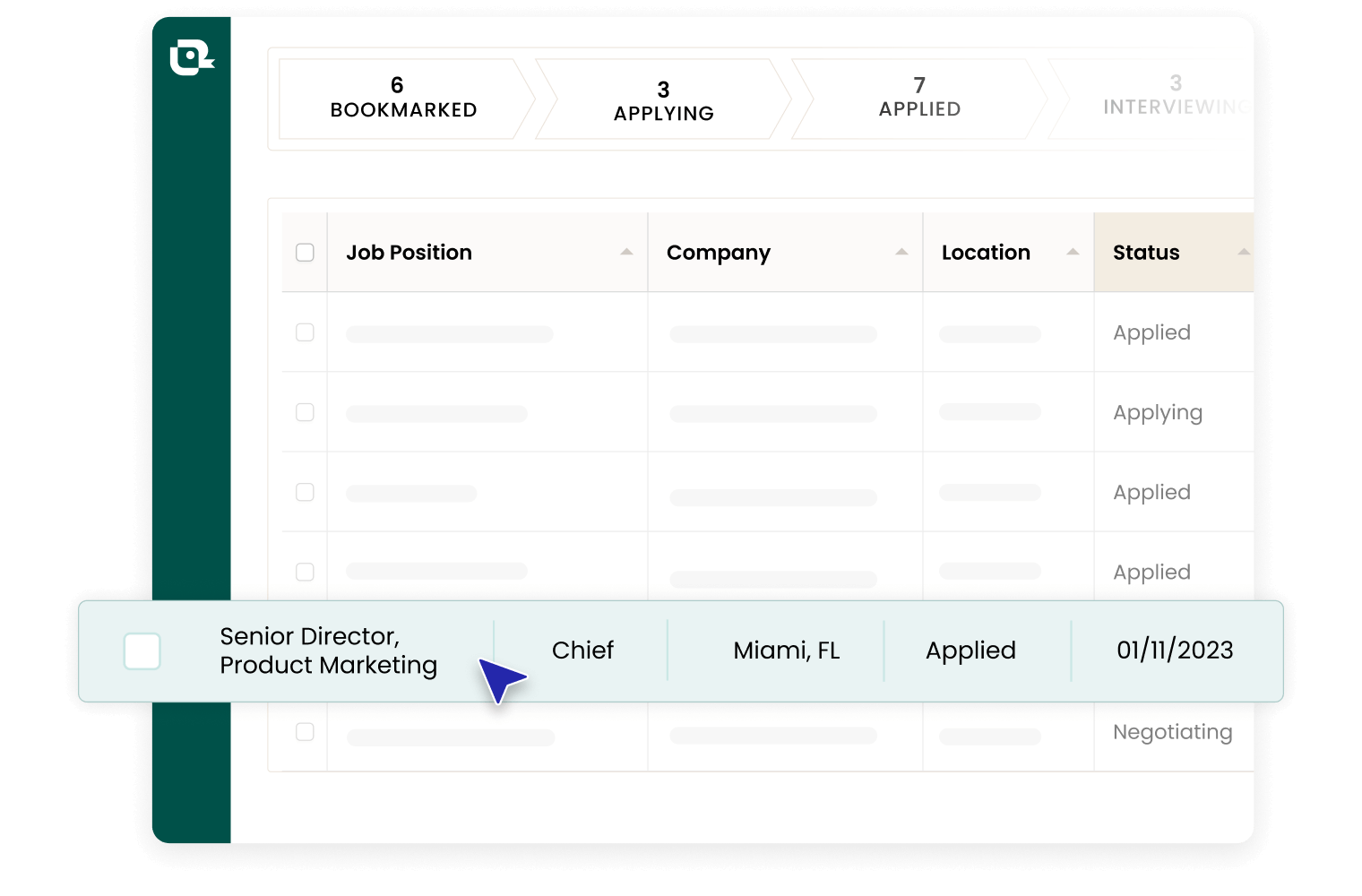
Python Django Developer Interview Questions and Answers

"Can you explain the Model-View-Template (MVT) architecture in Django?"
This question assesses your understanding of Django's core architecture. It's crucial to show your grasp of Django's components and how they interact to deliver web applications.
How to Answer It
Describe each component of the MVT architecture and its role in a Django application. Use examples to illustrate how you've worked with models, views, and templates in the past.
Example Answer
"In Django, the Model-View-Template architecture is a variation of the MVC framework. The Model defines the data structure, the View handles the business logic and interacts with the model, and the Template specifies the structure of the output. In my last project, I created a user authentication system where the User model stored user information, the view handled login and logout functionality, and the template displayed the user profile page."

"How do you ensure the security of a Django web application?"
This question evaluates your knowledge of web security practices within the Django framework. It's an opportunity to demonstrate your proactive approach to security.
How to Answer It
Discuss Django's built-in security features and any additional measures you take to secure your applications. Mention specific examples of security practices you've implemented.
Example Answer
"Django provides several security features out of the box, such as protection against CSRF, SQL injection, and XSS attacks. I ensure security by using Django's user authentication system, regularly updating Django to the latest version, and employing HTTPS. For instance, in my recent project, I implemented two-factor authentication to enhance user account security."

"How do you optimize database queries in Django?"
This question tests your understanding of Django's ORM and your ability to write efficient database queries.
How to Answer It
Explain the techniques you use to optimize queries, such as indexing, query set methods like select_related and prefetch_related, and minimizing database hits.
Example Answer
"To optimize database queries in Django, I use indexing to speed up lookups and select_related for foreign key relationships to reduce the number of queries. For example, in a recent project, I reduced the query count on a dashboard page from 100+ to just 10 by using prefetch_related to fetch related objects in a single query."

"Describe how you would implement RESTful APIs in a Django project."
This question gauges your experience with API development and your familiarity with Django REST framework (DRF).
How to Answer It
Discuss your approach to building APIs using DRF, including serialization, authentication, and permission classes. Provide an example of an API you've built.
Example Answer
"I use Django REST framework for building RESTful APIs because of its modularity and ease of use. I define serializers for data representation, use viewsets to handle CRUD operations, and apply permission classes for access control. In my last project, I developed a product API that allowed for seamless integration with a front-end application, providing a full CRUD interface for product data."

"How do you handle migrations in Django?"
This question assesses your ability to manage database schema changes in Django projects.
How to Answer It
Explain the migration process in Django and how you manage migrations in a team environment or when deploying to production.
Example Answer
"Django's migration system allows for smooth schema changes without losing data. I create migrations whenever I modify the models and apply them with manage.py migrate. In a team setting, I coordinate with other developers to ensure migrations are applied in the correct order and use version control to manage migration files. For production, I test migrations on a staging server before deploying to avoid any disruptions."

"Explain how you would use Django signals."
This question explores your knowledge of Django's signals framework, which allows for decoupled applications.
How to Answer It
Describe what signals are and provide scenarios where you would use them. Highlight the benefits and potential drawbacks.
Example Answer
"Django signals allow for sending and receiving notifications when certain actions occur. They're useful for executing code in response to model changes without altering the model's code. I've used signals to send email notifications when a new user signs up. However, I use them sparingly to avoid hidden side-effects and maintain code clarity."

"What are class-based views in Django, and when would you use them?"
This question tests your understanding of Django's class-based views and your ability to choose the right tool for the job.
How to Answer It
Explain the concept of class-based views and compare them with function-based views. Discuss the scenarios in which you would prefer one over the other.
Example Answer
"Class-based views in Django encapsulate common patterns into classes for reusability and extensibility. I use them when I need to handle more complex workflows or when I want to take advantage of inheritance to reduce code duplication. For example, I used a ListView to display a paginated list of products and a DetailView to show individual product details."

"How do you manage static files in Django?"
This question checks your understanding of serving static files in a Django application, which is essential for handling CSS, JavaScript, and images.
How to Answer It
Discuss how Django handles static files in development and production environments. Mention any third-party storage systems you've used.
Example Answer
"In development, Django serves static files using its own static file view. For production, I configure Django to use a more efficient static file server like Nginx or a CDN. I also use collectstatic to gather all static files into a single directory. In my previous role, I integrated Amazon S3 to store and serve static files, which improved our application's load time and scalability."Which Questions Should You Ask in a Python Django Developer Interview?
In the competitive field of Python Django development, the questions you ask during an interview are a testament to your technical acumen, engagement with the role, and your strategic thinking. They not only leave a lasting impression on the interviewer, reflecting your depth of knowledge and interest in the position, but they also serve as a critical tool for you to determine if the job aligns with your career goals and values. As a Python Django Developer, your inquiries should delve into the specifics of the company's development practices, the technical stack, and the challenges you might face, as well as the opportunities for growth and learning. By asking insightful questions, you position yourself as a discerning candidate, keen to understand the nuances of the role and the company culture, ensuring that the job is the right fit for you.
Good Questions to Ask the Interviewer
"Can you describe the current projects the development team is working on and how Django is being utilized within these projects?"
This question demonstrates your interest in the practical application of Django in the company's projects and can give you insight into the complexity and scale of the work you would be involved in. It also hints at your desire to understand the company's commitment to Django as part of their tech stack.
"What are the most significant challenges the development team has faced with Django, and how were they addressed?"
Asking about challenges not only shows that you are realistic about the fact that problems occur, but it also gives you an idea of the company's problem-solving culture. It can reveal the level of support you might expect and the opportunities for innovation and learning.
"How does the team stay updated with the latest Django developments and Python best practices?"
This question indicates your commitment to continuous learning and professional development. The answer will help you gauge whether the company values staying current with technology trends and whether it provides opportunities for skill enhancement.
"Could you explain the company's code review process and how it contributes to the quality of the software developed?"
Understanding the code review process is crucial as it reflects on the company's dedication to code quality, mentorship, and collaborative working environment. It also shows your concern for maintaining high standards in your work and your willingness to engage with peers constructively.
What Does a Good Python Django Developer Candidate Look Like?
In the realm of web development, a proficient Python Django developer is a blend of technical prowess and practical problem-solving skills. Employers and hiring managers are on the lookout for candidates who not only have a firm grasp of the Django framework and Python language but also exhibit a keen understanding of web technologies and user experience. A strong candidate is someone who can efficiently integrate back-end capabilities with front-end design, ensuring a seamless, scalable, and maintainable web application. They are expected to be agile, adaptive, and collaborative, capable of working in fast-paced environments and contributing to all phases of the development lifecycle.
Deep Understanding of Django and Python
A good Django developer must have an in-depth knowledge of the Django framework and the Python language. This includes familiarity with Django's ORM, class-based views, templates, forms, authentication, and the REST framework for API development.
Web Development Expertise
Candidates should demonstrate a strong understanding of web development principles, including HTTP, client-server architecture, and RESTful design patterns. They should also be proficient in front-end technologies such as HTML, CSS, and JavaScript to ensure they can work effectively with front-end developers.
Database Proficiency
Proficiency in database design and management is crucial. This includes experience with relational databases such as PostgreSQL or MySQL, as well as an understanding of data modeling, indexing, and query optimization.
Version Control and Collaboration
Experience with version control systems like Git is essential. A good candidate knows how to collaborate with other developers using these tools, manage branches, resolve merge conflicts, and maintain a clean commit history.
Testing and Debugging Skills
A strong candidate will prioritize writing tests and be adept at using Django's test framework to ensure code quality and reliability. They should also be skilled in debugging and able to quickly identify and resolve issues.
Performance Optimization
Knowledge of performance optimization techniques is important. Candidates should understand how to use Django's caching framework and other strategies to improve application speed and efficiency.
Security Awareness
A good Django developer is always conscious of security best practices, including understanding common vulnerabilities in web applications and how to defend against them using Django's built-in security features.
Effective Communication
Clear and articulate communication skills are vital. This includes the ability to document code effectively, communicate complex technical concepts to non-technical team members, and collaborate with stakeholders to refine requirements.
A candidate who embodies these qualities is not only technically equipped to handle the demands of a Django development role but is also a versatile team player who can contribute to the overall success of projects in a dynamic and collaborative tech environment.
Interview FAQs for Python Django Developers
What is the most common interview question for Python Django Developers?
"How do you structure your Django models?" This question assesses your grasp of database design and Django ORM. A solid answer should highlight your understanding of model relationships, data normalization, and Django's model field types. It's beneficial to discuss practices like using `ForeignKey` for one-to-many relationships, `ManyToManyField` for many-to-many, and incorporating `Meta` options for model customization, reflecting a thoughtful approach to efficient and maintainable database architecture.
What's the best way to discuss past failures or challenges in a Python Django Developer interview?
To demonstrate problem-solving skills in a Django interview, detail a complex web development issue you faced. Explain your systematic debugging process, how you isolated the problem within Django's framework, and the creative coding solutions you implemented. Highlight your use of Django's built-in tools or external libraries to resolve the issue efficiently. This shows your technical proficiency, resourcefulness, and familiarity with Django's ecosystem, underscoring your ability to tackle challenges effectively.
How can I effectively showcase problem-solving skills in a Python Django Developer interview?
To demonstrate problem-solving skills in a Django interview, detail a complex web development issue you faced. Explain your systematic debugging process, how you isolated the problem within Django's framework, and the creative coding solutions you implemented. Highlight your use of Django's built-in tools or external libraries to resolve the issue efficiently. This shows your technical proficiency, resourcefulness, and familiarity with Django's ecosystem, underscoring your ability to tackle challenges effectively.
Up Next
Python Django Developer Job Title Guide
Copy Goes Here.
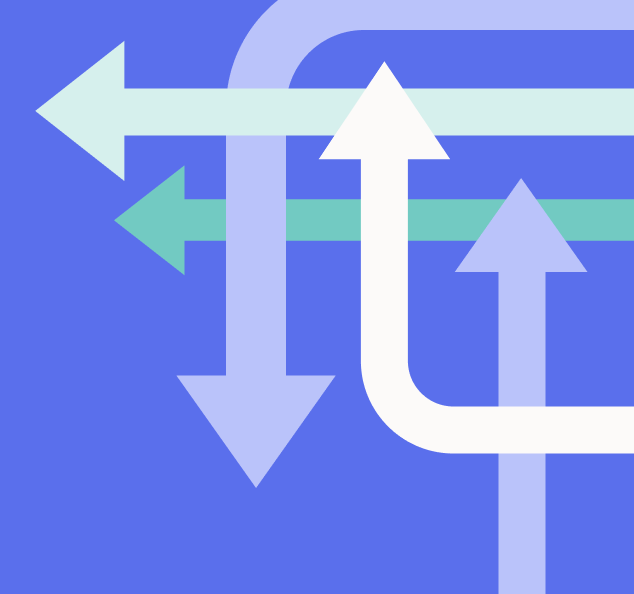