Interviewing as a Java Developer
Navigating the landscape of Java Developer interviews can be as intricate as the code you write. These interviews are more than a test of your programming prowess; they are a multifaceted examination of your technical acumen, problem-solving skills, and ability to adapt to new challenges.
In this guide, we'll dissect the array of questions that Java Developers face, from core language specifics to system design and beyond. We'll provide insights into crafting responses that showcase your expertise, the significance behind each question, and what interviewers are truly seeking in a top-tier candidate. Whether you're preparing for algorithmic challenges or situational queries, this guide is your comprehensive resource for excelling in your Java Developer interviews, positioning you to not just answer questions, but to demonstrate the depth of your knowledge and your passion for innovation.
Types of Questions to Expect in a Java Developer Interview
Java Developer interviews are designed to probe not only your technical expertise but also your problem-solving abilities, design thinking, and collaboration skills. Recognizing the different types of questions you may encounter can help you prepare more effectively and demonstrate your full range of abilities. Here's an overview of the question categories that are commonly used to assess Java Developers and what each type aims to uncover about your qualifications.
Core Java and Object-Oriented Programming Questions
Questions in this category will test your fundamental knowledge of Java and object-oriented programming (OOP) concepts. Expect to answer queries about Java's basic syntax, data types, and control structures, as well as OOP principles like inheritance, encapsulation, polymorphism, and abstraction. These questions aim to verify your understanding of the language's foundational elements and your ability to write clean, efficient code.
Java Frameworks and Libraries Questions
Given the vast ecosystem of Java, you'll likely face questions about popular frameworks and libraries such as Spring, Hibernate, or Apache Maven. These inquiries assess your familiarity with the tools that enhance Java's capabilities and your experience in using them to build scalable, maintainable applications. They also reveal your ability to leverage existing solutions to speed up development and improve software quality.
System Design and Architecture Questions
These questions evaluate your ability to design robust systems and understand architectural patterns. You might be asked to outline the architecture for a new application or to discuss the trade-offs of different design choices. This category tests your strategic thinking, your understanding of best practices in software architecture, and your foresight in planning for scalability, reliability, and security.
Problem-Solving and Algorithm Questions
Problem-solving questions often involve writing code on the spot to solve a specific algorithmic challenge. These questions measure your analytical skills, your proficiency in translating logical solutions into code, and your understanding of algorithms and data structures. They are designed to test how you approach complex problems and whether you can deliver efficient, optimized solutions.
Concurrency and Multithreading Questions
Java is known for its robust multithreading capabilities, so expect questions about concurrency, thread life cycle, synchronization, and inter-thread communication. These questions test your knowledge of concurrent programming in Java, which is crucial for developing high-performance applications that can handle multiple simultaneous processes or users.
Behavioral and Communication Questions
Beyond technical skills, interviews often include behavioral questions aimed at understanding how you work within a team, handle stress, and communicate with colleagues. Expect to discuss past projects, how you've dealt with difficult situations, or how you stay updated with new technologies. These questions seek to gauge your soft skills, which are essential for effective collaboration and project success.
By familiarizing yourself with these question types and reflecting on your experiences and knowledge in each area, you can approach a Java Developer interview with confidence. Tailoring your preparation to these categories will not only help you anticipate what's to come but also enable you to present a well-rounded picture of your capabilities as a Java Developer.
Preparing for a Java Developer Interview
Preparing for a Java Developer interview is a critical step in showcasing your technical expertise, problem-solving abilities, and understanding of object-oriented design principles. It's not just about proving that you can write code; it's about demonstrating that you can think like a Java developer, solve complex problems, and contribute effectively to a team. A well-prepared candidate stands out by displaying a deep understanding of the Java ecosystem, best practices, and the ability to apply this knowledge in a practical setting. This preparation can make the difference between landing the job and being passed over for another candidate.
How to do Interview Prep as a Java Developer
- Master Core Java Concepts: Ensure you have a strong grasp of Java fundamentals such as data types, control flow, error handling, generics, collections, concurrency, and memory management.
- Understand Object-Oriented Programming: Be prepared to discuss and apply OOP principles such as encapsulation, inheritance, abstraction, and polymorphism in Java.
- Review Design Patterns and Best Practices: Familiarize yourself with common design patterns and best practices in Java development to demonstrate your ability to write clean, maintainable code.
- Practice Coding Problems: Sharpen your problem-solving skills by practicing coding problems on platforms like LeetCode, HackerRank, or CodeSignal, focusing on algorithms and data structures.
- Study Frameworks and Libraries: Gain knowledge of popular Java frameworks and libraries such as Spring, Hibernate, and Apache Maven, which are often used in enterprise environments.
- Prepare for Technical Questions: Be ready to answer technical questions that test your understanding of Java's features, such as exception handling, multithreading, I/O streams, and Java Virtual Machine (JVM).
- Understand the Development Lifecycle: Show that you're familiar with the software development lifecycle and methodologies like Agile, Scrum, or Test-Driven Development (TDD).
- Brush Up on Database and SQL: Review basic database concepts and SQL, as Java developers often need to interact with databases.
- Review Your Past Projects: Be prepared to discuss your previous work, highlighting specific challenges you faced and how you overcame them using Java.
- Prepare Your Own Questions: Develop insightful questions to ask the interviewer about the company's tech stack, development processes, or any upcoming projects.
- Mock Interviews: Practice with mock interviews to get comfortable with the format and receive feedback on your performance.
By following these steps, you'll demonstrate not only your technical proficiency in Java but also your commitment to excellence and continuous improvement. This preparation will help you to engage confidently in discussions during your interview and prove that you are the right fit for the Java Developer role.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free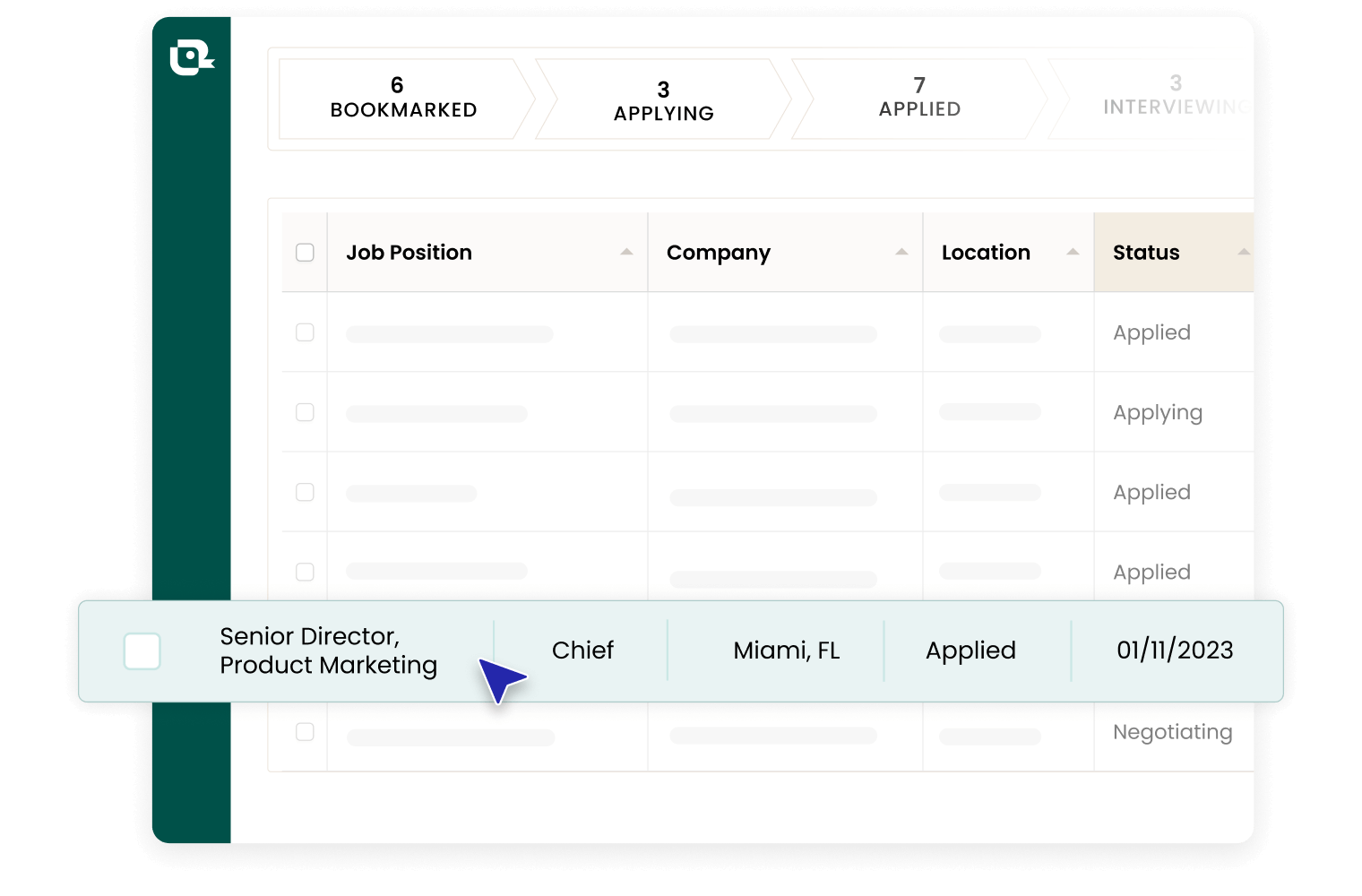
Java Developer Interview Questions and Answers

"What are the key features of Java that distinguish it from other programming languages?"
This question evaluates your understanding of Java's core concepts and its unique advantages. It's an opportunity to demonstrate your knowledge of the language and its application in software development.
How to Answer It
Discuss the fundamental features of Java such as object-oriented programming, platform independence, security, and robustness. Explain how these features make Java suitable for various types of applications.
Example Answer
"Java is an object-oriented language, which makes it ideal for creating modular programs and reusable code. One of its key features is the Write Once, Run Anywhere (WORA) capability, which means compiled Java code can run on all platforms that support Java without the need for recompilation. Java's security features are also robust, with the language designed to prevent various types of attacks. Additionally, Java's automatic memory management helps prevent memory leaks and other related issues, making it a reliable choice for large-scale applications."

"Can you explain the concept of the Java Virtual Machine (JVM) and its significance?"
This question assesses your technical knowledge of Java's runtime environment. Understanding JVM is crucial for Java developers as it affects how they write and optimize code.
How to Answer It
Describe what the JVM is, its role in running Java applications, and its impact on Java's platform independence. Mention the components of the JVM such as the classloader, the memory area, the execution engine, etc.
Example Answer
"The Java Virtual Machine (JVM) is the cornerstone of Java's platform independence. It's a virtual machine that provides a runtime environment to execute Java bytecode. The JVM performs several operations, such as loading code, verifying code, executing code, and providing runtime environment. The JVM enables Java applications to run on any device or operating system that has a JVM implementation, making Java applications highly portable."

"How do you handle exceptions in Java?"
This question tests your understanding of error handling in Java. Exception handling is a critical concept for writing robust and fault-tolerant applications.
How to Answer It
Explain the difference between checked and unchecked exceptions, the try-catch-finally construct, and the use of throw and throws keywords. Provide an example of how you have used exception handling in a past project.
Example Answer
"In Java, exceptions are handled using try-catch blocks. Checked exceptions are those that the compiler checks at compile-time, while unchecked exceptions occur at runtime. In my previous project, I used try-catch blocks to gracefully handle potential I/O exceptions when reading from a file, ensuring the program continued to run and provided the user with a meaningful error message. I also used the finally block to ensure that the file's resources were closed properly, regardless of whether an exception occurred."

"What is the difference between an abstract class and an interface in Java, and when would you use each?"
This question explores your understanding of Java's object-oriented principles and your ability to design a system using these concepts.
How to Answer It
Discuss the purpose of abstract classes and interfaces, their differences, and scenarios where one would be preferred over the other. Highlight your experience with using both in your past work.
Example Answer
"Abstract classes and interfaces are used to achieve abstraction in Java. An abstract class can provide some implementation and declare abstract methods, while an interface can only declare methods (until Java 8, after which it can also have default and static methods). I use abstract classes when I want to share code among closely related classes, and interfaces when I need to define a contract for unrelated classes. For example, in a recent project, I used an interface to define a common action across different types of database connections, while an abstract class was used to provide common methods for similar types of transactions."

"How does Java achieve memory management, and what is garbage collection?"
This question assesses your knowledge of Java's automatic memory management, which is a key feature of the language.
How to Answer It
Explain how Java manages memory through the use of the garbage collector and the importance of this process. You can also mention the different types of garbage collectors and when to use them.
Example Answer
"Java achieves memory management through its garbage collection process, which helps in reclaiming memory used by objects that are no longer referenced in the program. This is essential for preventing memory leaks and ensuring efficient use of resources. The JVM has several garbage collectors like the Serial GC, Parallel GC, CMS, and G1 GC, each designed for different types of applications and workloads. In my last project, we used the G1 Garbage Collector as it was well-suited for our low-latency application with a large heap size."

"What is multithreading in Java, and how do you implement it?"
This question tests your knowledge of concurrent programming in Java, which is crucial for building responsive and high-performance applications.
How to Answer It
Discuss the concept of threads in Java, the ways to create them using the Runnable interface or extending the Thread class, and the common issues to consider, such as synchronization and deadlock.
Example Answer
"Multithreading in Java allows concurrent execution of two or more parts of a program for maximum utilization of CPU. Threads can be created by implementing the Runnable interface or by extending the Thread class. In my experience, I prefer implementing Runnable as it allows a class to extend another class as well. I've used synchronized blocks and methods to prevent race conditions and used wait/notify mechanisms to avoid deadlocks in a high-frequency trading application I worked on."

"Explain the concept of Generics in Java and why it's used."
This question gauges your understanding of Java's type system and your ability to write flexible and type-safe code.
How to Answer It
Describe what Generics are, their benefits in terms of type safety and code reusability, and give an example of how you've used Generics in your code.
Example Answer
"Generics enable types (classes and interfaces) to be parameters when defining classes, interfaces, and methods. They provide stronger type checks at compile time and eliminate the need for type casting, which can lead to ClassCastException. Generics also enable us to implement generic algorithms. In my previous role, I used Generics to create a custom data structure that could hold any type of objects while ensuring compile-time type safety, which reduced runtime errors and improved code readability."

"Can you discuss the use of the 'final' keyword in Java?"
This question examines your understanding of Java's keyword usage and its implications on code design and behavior.
How to Answer It
Explain the different contexts in which the 'final' keyword can be used (variables, methods, and classes) and the reasons for its use in each context.
Example Answer
"The 'final' keyword in Java can be used to declare a variable as constant, preventing its value from being modified. It can also make a method unable to be overridden by subclasses, and a class unable to be subclassed. I often use 'final' for constants to ensure they are not accidentally changed, as well as to optimize performance. For example, in a recent project, I declared database connection strings as final to ensure they remained immutable throughout the application's lifecycle."Which Questions Should You Ask in a Java Developer Interview?
In the competitive field of Java development, the questions you ask in an interview can be as revealing as the answers you provide. They serve a dual purpose: they demonstrate your technical acumen, problem-solving abilities, and engagement with the role, while also allowing you to evaluate if the position aligns with your career objectives and personal values. For Java Developers, asking insightful questions can highlight your depth of understanding in software development, your foresight in technology trends, and your ability to integrate into the company's technical environment. Moreover, it's an opportunity to uncover the specifics of the projects you'll be working on, the methodologies the team employs, and the company's commitment to innovation and professional growth.
Good Questions to Ask the Interviewer
"Can you describe the current Java project I would be working on and the team's development methodology?"
This question demonstrates your eagerness to understand the specifics of your potential role and how you would fit into the existing workflow. It also gives you insight into the company's approach to software development, whether they use Agile, Scrum, or another methodology, and how you can contribute effectively.
"What are the biggest technical challenges the development team has faced recently, and how were they addressed?"
Asking about challenges shows that you're not only interested in the successes but also in how the team overcomes obstacles. It can reveal the company's problem-solving culture and resilience, as well as areas where your skills might make a significant impact.
"How does the organization stay current with the evolving Java ecosystem, and how is innovation fostered within the team?"
This question reflects your commitment to continuous learning and innovation. It helps you gauge whether the company values staying up-to-date with the latest Java technologies and practices, which is crucial for your professional development as a Java Developer.
"What opportunities for professional growth and advancement does the company offer for someone in this Java Developer role?"
Inquiring about growth opportunities shows that you're thinking long-term and are interested in a career, not just a job. It also helps you understand if the company supports skill enhancement and career progression, which can be pivotal in your decision-making process.
What Does a Good Java Developer Candidate Look Like?
In the realm of software development, Java remains a cornerstone language and platform, and the demand for skilled Java developers continues to be robust. A good Java developer candidate is not only proficient in the language itself but also exhibits a deep understanding of object-oriented programming principles, design patterns, and software engineering best practices. Employers and hiring managers are on the lookout for candidates who can demonstrate technical excellence alongside soft skills such as problem-solving, effective communication, and the ability to work collaboratively within a team. A strong Java developer is adaptable, continuously learning, and able to apply their knowledge to a diverse range of applications and industries.
A good Java developer candidate also understands the importance of writing clean, maintainable code and has a strong grasp of the Java ecosystem, including frameworks like Spring and Hibernate. They are expected to contribute to all phases of the development lifecycle and provide technical leadership whenever necessary, making them a critical asset to any development team.
Technical Proficiency
A strong candidate has a solid grasp of core Java concepts, such as concurrency, collections, and stream API. They are also familiar with the Java Virtual Machine (JVM) and understand how Java applications run and perform.
Understanding of Object-Oriented Design
Candidates should demonstrate a clear understanding of object-oriented design principles and patterns, which are fundamental to creating robust and scalable Java applications.
Experience with Frameworks and Tools
Proficiency in popular Java frameworks like Spring and Hibernate is often required, as is experience with build tools like Maven or Gradle and version control systems such as Git.
Problem-Solving Skills
Good Java developers are analytical thinkers with strong problem-solving skills. They can deconstruct complex problems and devise efficient and effective solutions.
Adaptability and Continuous Learning
The technology landscape is ever-changing, and a good Java developer is always learning and adapting to new tools, technologies, and methodologies.
Effective Communication
The ability to communicate complex technical ideas in a clear and concise manner is crucial. This includes both verbal and written communication skills, as well as the ability to work collaboratively with team members and stakeholders.
Understanding of Software Development Best Practices
A strong candidate is well-versed in software development best practices, including test-driven development (TDD), continuous integration (CI), and continuous deployment (CD), which are essential for maintaining high code quality and facilitating collaboration in modern development environments.
By embodying these qualities and skills, a Java developer candidate can stand out in the competitive job market and demonstrate their potential to contribute meaningfully to any organization's software development efforts.
Interview FAQs for Java Developers
What is the most common interview question for Java Developers?
"What are the key features of Java?" This question assesses your foundational knowledge and understanding of the language's principles. A comprehensive response should highlight Java's object-oriented nature, platform independence through the JVM, garbage collection, and strong memory management, while also touching on newer features like lambda expressions and the stream API introduced in recent updates. Your answer should reflect an appreciation for Java's evolution and its application in modern software development.
What's the best way to discuss past failures or challenges in a Java Developer interview?
To demonstrate problem-solving skills in a Java Developer interview, recount a complex coding challenge you faced. Detail your systematic debugging process, the Java tools or frameworks employed, and how you optimized the code for efficiency. Highlight your iterative testing and the incorporation of design patterns, reflecting on the successful outcome and performance improvements. This illustrates your technical acumen, strategic thinking, and commitment to quality code.
How can I effectively showcase problem-solving skills in a Java Developer interview?
To demonstrate problem-solving skills in a Java Developer interview, recount a complex coding challenge you faced. Detail your systematic debugging process, the Java tools or frameworks employed, and how you optimized the code for efficiency. Highlight your iterative testing and the incorporation of design patterns, reflecting on the successful outcome and performance improvements. This illustrates your technical acumen, strategic thinking, and commitment to quality code.
Up Next
Java Developer Job Title Guide
Copy Goes Here.
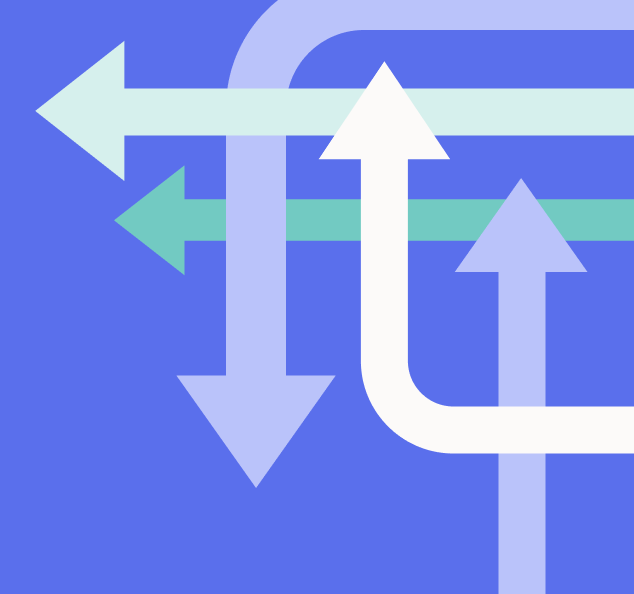