Interviewing as a Python Developer
Navigating the path to becoming a Python Developer is an intricate journey, with the interview stage serving as a pivotal moment in your career progression. Python Developers are expected to possess a robust blend of programming prowess, problem-solving skills, and a deep understanding of software development principles. Interviews for these roles are designed to probe not only your technical expertise but also your ability to collaborate, innovate, and adapt in a fast-paced environment.
In this guide, we'll dissect the array of questions that Python Developer candidates may encounter, from practical coding challenges to conceptual inquiries that reveal your thought process and proficiency. We'll provide insights into crafting compelling responses, preparing effectively for technical evaluations, and the characteristics that define a standout Python Developer. This resource is your strategic partner, equipping you with the knowledge to excel in your interviews and propel your career forward.
Types of Questions to Expect in a Python Developer Interview
Python Developer interviews are designed to probe not only your technical knowledge but also your problem-solving abilities, coding proficiency, and understanding of software development principles. Recognizing the different types of questions you may encounter can help you prepare more effectively and demonstrate your full range of skills. Here's an overview of the question categories that are commonly presented in Python Developer interviews.
Technical Proficiency Questions
Technical proficiency questions are the cornerstone of any Python Developer interview. These questions assess your understanding of Python syntax, data structures, algorithms, and libraries. You might be asked to write code on the spot, debug a piece of code, or explain how certain functions work. These questions test your coding skills and your ability to apply Python to solve complex problems.
Conceptual Understanding Questions
Beyond syntax, you'll likely face questions that delve into your grasp of programming concepts. These can range from object-oriented programming principles to understanding design patterns and memory management. Such questions evaluate your foundational knowledge and your ability to apply abstract concepts to real-world programming challenges.
Practical Application Questions
Interviewers want to see how you translate theory into practice. Practical application questions might involve working through a problem statement to create a functional piece of software or modifying existing code to add new features. These questions assess your hands-on development skills, efficiency, and the quality of your code.
System Design and Architecture Questions
For more senior Python Developer roles, expect questions about system design and architecture. These will test your ability to design scalable and maintainable systems. You might be asked to outline how you would structure a new application or improve an existing system's architecture, showcasing your strategic thinking and planning abilities.
Behavioral and Communication Questions
Python Developers often work in teams, so it's important to demonstrate soft skills. Behavioral questions explore your past experiences, how you've handled conflicts, and your approach to teamwork and collaboration. Communication questions may involve explaining complex technical concepts to non-technical stakeholders, revealing your ability to bridge the gap between technical and non-technical realms.
Problem-Solving and Critical Thinking Questions
These questions are designed to assess your logical thinking and problem-solving approach. You might be presented with a coding puzzle or a complex algorithmic challenge to solve. The goal is to understand your thought process, creativity, and persistence in tackling difficult problems.
Preparing for these question types will not only help you feel more confident during the interview but also provide a structured way to showcase the breadth and depth of your skills as a Python Developer. Tailoring your study and practice to these categories can greatly improve your chances of success.
Preparing for a Python Developer Interview
Preparing for a Python Developer interview requires a blend of technical expertise, problem-solving skills, and a deep understanding of Python's ecosystem. It's not just about knowing the language syntax; it's about demonstrating your ability to apply Python to solve real-world problems. A well-prepared candidate stands out by showing proficiency in Python, familiarity with common libraries and frameworks, and the ability to think critically about software design and development. By investing time in preparation, you not only increase your confidence but also exhibit a commitment to your craft and the potential role.
How to do Interview Prep as a Python Developer
- Master Core Python Concepts: Ensure you have a strong grasp of Python basics such as data types, control structures, functions, classes, and exception handling. Be prepared to discuss more advanced topics like decorators, generators, context managers, and comprehension.
- Understand Python Libraries and Frameworks: Familiarize yourself with commonly used libraries such as NumPy, Pandas, Requests, and frameworks like Django or Flask, depending on the job description. Be ready to discuss when and why you would use them.
- Practice Coding Problems: Sharpen your problem-solving skills by practicing coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Focus on algorithms, data structures, and Pythonic ways to solve tasks.
- Review Version Control Systems: Be comfortable with version control systems, especially Git. Understand how to clone repositories, create branches, merge changes, and resolve conflicts.
- Prepare for Technical Questions: Be ready to answer technical questions that test your understanding of software development principles, design patterns, and best practices in Python coding.
- Understand Testing: Know how to write unit tests in Python using frameworks like unittest or pytest. Discuss the importance of testing and possibly Test-Driven Development (TDD).
- Brush Up on System Design: Be prepared to discuss system design principles and how you would architect a scalable, maintainable application in Python.
- Prepare Your Own Questions: Develop insightful questions about the company's tech stack, development processes, and culture. This demonstrates your genuine interest in the role and the organization.
- Mock Interviews: Practice with mock interviews to get comfortable with the format and receive feedback. Use resources like Pramp or interviewing.io, or practice with peers or mentors.
By following these steps, you'll be able to showcase not only your technical knowledge but also your problem-solving abilities and your readiness to contribute as a Python Developer. This preparation will help you to engage confidently in discussions and prove that you are a strong fit for the role.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free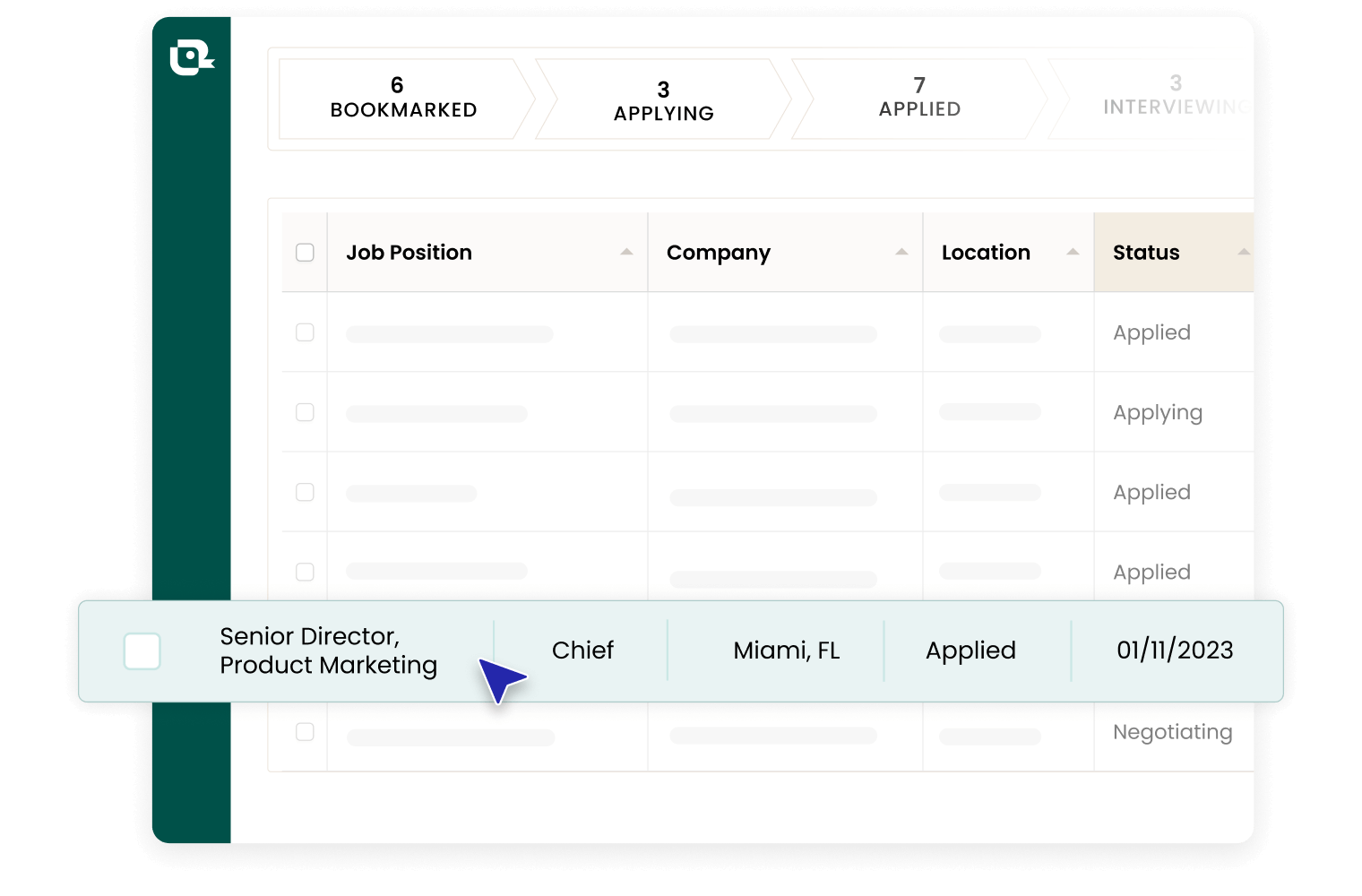
Python Developer Interview Questions and Answers

"What are some of the key differences between Python 2 and Python 3?"
This question assesses your knowledge of Python's evolution and your ability to adapt to the language's updates. It also shows whether you're up-to-date with current Python standards.
How to Answer It
Discuss the major changes from Python 2 to Python 3, such as print function, integer division, Unicode, and error handling. Explain how these changes impact code development and maintenance.
Example Answer
"In Python 3, print became a function, requiring parentheses. Integer division now results in a float, and strings are Unicode by default, enhancing internationalization support. Error handling changed from 'except Exception, e:' to 'except Exception as e:', which is clearer. I've adapted to these changes by consistently using Python 3 for new projects and converting Python 2 code when necessary."

"How do you manage memory in Python?"
This question evaluates your understanding of Python's memory management, including garbage collection and memory optimization techniques.
How to Answer It
Explain Python's automatic memory management, reference counting, and garbage collection. Mention any best practices you follow to write memory-efficient code.
Example Answer
"Python uses automatic memory management with reference counting and a garbage collector to clean up unreferenced objects. To manage memory, I use generators for large data sets, avoid circular references, and utilize 'with' statements to ensure that resources are properly released. Additionally, I sometimes use the 'gc' module to manually intervene in the garbage collection process when necessary."

"Can you explain the Global Interpreter Lock (GIL) and how it affects concurrency in Python?"
This question probes your understanding of Python's concurrency model and the challenges posed by the GIL.
How to Answer It
Describe what the GIL is and its impact on multi-threaded Python programs. Discuss strategies to work around GIL limitations, such as using multiprocessing or other concurrency libraries.
Example Answer
"The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes at once. This can be a bottleneck in CPU-bound multi-threaded programs. To mitigate this, I use the multiprocessing module to run code across multiple processes that each have their own Python interpreter and memory space, or I use libraries like asyncio for IO-bound tasks."

"What is a decorator in Python, and can you provide an example of how you have used one?"
This question assesses your knowledge of advanced Python features and your ability to write reusable and maintainable code.
How to Answer It
Explain the concept of decorators and how they can be used to modify or enhance the behavior of functions or methods. Provide a clear example from your experience.
Example Answer
"A decorator is a higher-order function that wraps another function or method, allowing you to add functionality before or after the wrapped function runs. I've used decorators to implement cross-cutting concerns like logging, authorization checks, and caching. For example, I created a @login_required decorator that verifies if a user is authenticated before allowing access to certain web endpoints."

"How do you ensure that your Python code is both efficient and maintainable?"
This question explores your coding practices and your ability to balance performance with code quality.
How to Answer It
Discuss your approach to writing clean code, including the use of coding standards, code reviews, and profiling tools. Mention specific techniques you use to optimize Python code.
Example Answer
"To ensure efficiency, I follow the Zen of Python principles, write modular code, and use profiling tools like cProfile to identify bottlenecks. For maintainability, I adhere to PEP 8 style guidelines, write comprehensive tests, and document my code thoroughly. For example, in a recent project, I used list comprehensions and generator expressions for better performance and readability, and I refactored complex functions into smaller, testable units."

"What is your approach to testing in Python?"
This question gauges your commitment to code quality and your familiarity with Python's testing frameworks.
How to Answer It
Describe your experience with testing frameworks like unittest or pytest. Explain how you integrate testing into your development workflow and the types of tests you write (unit, integration, etc.).
Example Answer
"I use pytest for its simplicity and powerful features. My approach includes writing unit tests for individual components and integration tests to ensure they work together correctly. I practice test-driven development (TDD), where I write tests before implementing functionality, which leads to more reliable and maintainable code. For instance, on my last project, I achieved 90% test coverage, which significantly reduced bugs in production."

"Explain how you would use Python's data structures to solve a problem. Provide an example."
This question tests your problem-solving skills and your ability to choose the right data structure for a given task.
How to Answer It
Discuss the various data structures available in Python, such as lists, tuples, sets, and dictionaries, and when to use each. Provide a specific example of how you've used one to solve a problem.
Example Answer
"In Python, choosing the right data structure is crucial for performance and readability. For example, I used a dictionary in a recent project to count the frequency of words in a document because it provides constant-time complexity for lookups and updates. This choice allowed for an efficient solution that was easy to implement and understand."

"How do you handle version control and collaborative coding in Python projects?"
This question assesses your experience with collaborative development practices and tools.
How to Answer It
Talk about your proficiency with version control systems like Git and collaborative platforms such as GitHub or GitLab. Mention any branching strategies or collaborative coding practices you've used.
Example Answer
"I use Git for version control, adhering to the feature branch workflow. I create a new branch for each feature or bug fix, which keeps the master branch stable. Pull requests are used for code reviews, ensuring that multiple eyes check the code before it's merged. For example, in my last team, we used GitHub for collaboration, which streamlined our development process and improved code quality through peer reviews."Which Questions Should You Ask in a Python Developer Interview?
In the competitive field of Python development, the questions you ask during an interview can be as telling as the answers you provide. They serve a dual purpose: showcasing your analytical skills and deep understanding of Python, and ensuring the role aligns with your career objectives and personal values. For Python Developers, the inquiries made can reflect your technical acumen, your foresight into the development lifecycle, and your potential fit within the team. By asking insightful questions, you not only convey your genuine interest in the position but also gather essential information to determine if the opportunity is conducive to your professional growth and aspirations.
Good Questions to Ask the Interviewer
"Can you describe the development workflow and the role of Python developers within it?"
This question demonstrates your eagerness to understand how you would integrate into the existing workflow and how Python is utilized within the team. It indicates that you are considering how to effectively collaborate within their structure and contribute to the team's success.
"What are the primary challenges that the development team is facing, and how can a Python developer help address them?"
Asking about challenges shows that you're not just looking for any job, but one where you can make a real impact. It also gives you insight into the current state of the projects and the team's approach to problem-solving, which can be crucial for your role.
"How does the company foster professional development for its technical staff, particularly for those specializing in Python?"
This question underscores your commitment to continuous learning and professional growth. It helps you gauge whether the company values and supports skill enhancement, which is vital for staying relevant in the ever-evolving field of Python development.
"Could you share an example of a project that the Python team recently worked on and what made it successful?"
Inquiring about specific projects and their outcomes allows you to understand the types of tasks you might be handling and the metrics for success within the organization. It also shows your interest in the company's achievements and the strategies they employ, aligning your expectations with the company's standards and practices.
What Does a Good Python Developer Candidate Look Like?
In the realm of software development, Python developers are in high demand due to the language's versatility and readability. A strong Python developer candidate is not only proficient in writing clean, efficient code but also exhibits a deep understanding of Python's ecosystems, such as its libraries and frameworks. Employers seek individuals who can think algorithmically and solve problems effectively. Beyond technical prowess, good Python developers are expected to collaborate with cross-functional teams, adapt to new technologies, and contribute to the design and architecture of software solutions.
A good Python developer candidate is someone who can leverage Python's strengths to create scalable and maintainable applications while also demonstrating the soft skills necessary to thrive in a team environment. They are expected to be proactive in learning and applying best practices, and they must be able to communicate complex technical concepts to non-technical stakeholders.
Technical Expertise
A strong candidate has a solid grasp of Python's syntax, data structures, and object-oriented programming principles. They should be familiar with Python's standard library and commonly used frameworks like Django or Flask.
Problem-Solving Skills
The ability to think through problems and come up with efficient solutions is crucial. This includes writing algorithms that are both effective and optimized for performance.
Understanding of Development Best Practices
Knowledge of coding standards, version control (e.g., Git), testing (unit, integration), and debugging is expected. A good Python developer also understands the importance of code readability and maintainability.
Experience with Databases
Proficiency in integrating Python applications with various database technologies, whether SQL (like PostgreSQL or MySQL) or NoSQL (like MongoDB), is highly valued.
Collaboration and Communication
Successful Python developers must work well in teams, often collaborating with other developers, data scientists, and stakeholders. They need to communicate technical details effectively.
Adaptability and Continuous Learning
The tech field is always evolving, and so are Python and its associated technologies. A good candidate is someone who is committed to continuous learning and can adapt to new tools and methodologies.
Understanding of Full Development Lifecycle
Experience with the full software development lifecycle, including requirements analysis, system design, implementation, testing, deployment, and maintenance, is a significant asset.
By embodying these qualities, a Python developer candidate can demonstrate their value to potential employers and stand out in the competitive field of software development.
Interview FAQs for Python Developers
What is the most common interview question for Python Developers?
"What is your approach to writing efficient and maintainable Python code?" This question evaluates your coding philosophy and practical skills. A compelling answer should highlight your understanding of Pythonic principles, code readability, and reuse. Discuss practices like leveraging list comprehensions, using generators for memory efficiency, applying decorators for modularity, and adhering to PEP 8 standards. Mention tools like linters or profilers you use to refine code quality and performance.
What's the best way to discuss past failures or challenges in a Python Developer interview?
To demonstrate problem-solving skills in a Python Developer interview, detail a complex coding challenge you faced. Explain your methodical approach, how you broke down the problem, the Python tools and libraries you utilized, and the logic behind your code solution. Highlight how you optimized performance or managed resources, and discuss the positive outcome, such as improved functionality or efficiency. This shows your technical proficiency and strategic thinking in practical Python applications.
How can I effectively showcase problem-solving skills in a Python Developer interview?
To demonstrate problem-solving skills in a Python Developer interview, detail a complex coding challenge you faced. Explain your methodical approach, how you broke down the problem, the Python tools and libraries you utilized, and the logic behind your code solution. Highlight how you optimized performance or managed resources, and discuss the positive outcome, such as improved functionality or efficiency. This shows your technical proficiency and strategic thinking in practical Python applications.
Up Next
Python Developer Job Title Guide
Copy Goes Here.
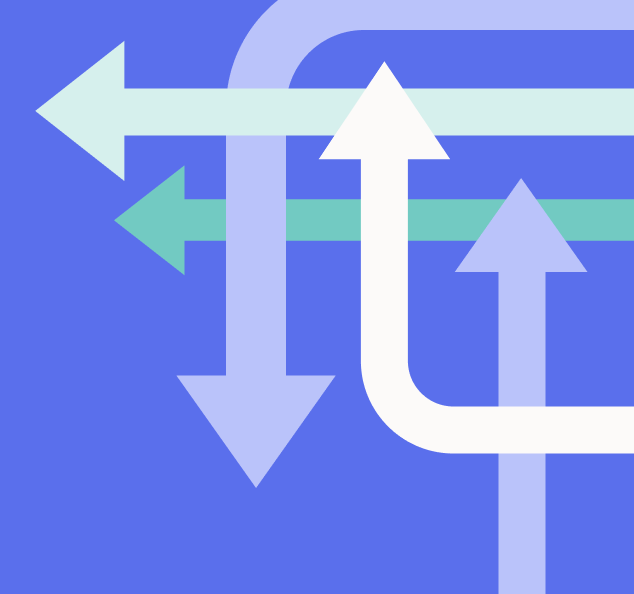