Interviewing as a Java Backend Developer
Navigating the landscape of Java Backend Developer interviews can be as intricate as the systems you aspire to build and maintain. These interviews are more than a mere assessment of your technical prowess; they are a multifaceted evaluation of your coding acumen, architectural understanding, and your ability to contribute to the backend of scalable applications.
In this guide, we'll dissect the array of questions that Java Backend Developers face, from the core Java concepts that underpin robust server-side solutions to the design patterns essential for crafting efficient, maintainable code. We'll provide insights into crafting responses that showcase your depth of knowledge, problem-solving skills, and readiness for the challenges of backend development. By delving into what makes a standout Java Backend Developer candidate, we'll equip you with the knowledge to not only respond to your interviewer's queries but to pose your own, demonstrating your industry acumen and engagement.
Types of Questions to Expect in a Java Backend Developer Interview
Java Backend Developer interviews are designed to probe not only your technical expertise but also your problem-solving abilities, design thinking, and collaboration skills. As a Java Backend Developer, you'll face a range of questions that test your knowledge of Java and its ecosystem, as well as your ability to apply this knowledge in real-world scenarios. Understanding the types of questions you may encounter will help you prepare effectively and demonstrate your full potential to prospective employers. Here's a look at the common question categories you should be ready for.
Core Java and Object-Oriented Programming (OOP) Questions
Questions in this category will assess your foundational knowledge of Java and OOP principles. Expect to answer queries about Java's core features, such as inheritance, encapsulation, polymorphism, and abstraction. You might also be tested on exception handling, collections, generics, and concurrency. These questions aim to gauge your understanding of Java's syntax and basic programming constructs.
System Design and Architecture Questions
These questions evaluate your ability to design scalable, maintainable, and efficient systems. You may be asked to outline the architecture for a new application or to improve an existing one. Interviewers are looking for your thought process on choosing the right frameworks, databases, and design patterns, as well as your understanding of microservices, RESTful APIs, and cloud services.
Data Structures and Algorithms Questions
Your knowledge of data structures and algorithms is crucial for writing optimized code. You'll likely face questions that require you to demonstrate your ability to solve complex problems using the most appropriate data structures (like arrays, lists, stacks, queues, trees, graphs) and algorithms (such as sorting, searching, recursion). These questions test your analytical skills and your proficiency in writing efficient, scalable code.
Database and SQL Questions
As a backend developer, interacting with databases is a key part of your role. Interviewers will test your understanding of database concepts, including normalization, indexing, transactions, and query optimization. You'll need to show your ability to write complex SQL queries and possibly discuss NoSQL alternatives, highlighting when and why you would use them.
Spring Framework and Java Enterprise Edition (JEE) Questions
Given the popularity of the Spring Framework and JEE in enterprise applications, you can expect questions on these topics. You might be asked about dependency injection, aspect-oriented programming, transaction management, and security. These questions assess your practical knowledge of the frameworks and libraries that are commonly used in Java backend development.
Behavioral and Communication Questions
These questions delve into your soft skills, which are as important as your technical abilities. Interviewers will ask about past projects, team experiences, and how you've overcome challenges. They aim to understand your teamwork, communication, and leadership qualities, as well as your ability to learn from mistakes and handle feedback.
Preparing for these question types will not only help you feel more confident going into your Java Backend Developer interview but will also allow you to showcase the breadth and depth of your skills. Remember, each question is an opportunity to demonstrate your expertise and how you can contribute to the success of the team and the company.
Preparing for a Java Backend Developer Interview
Preparing for a Java Backend Developer interview is a critical step in showcasing your technical expertise, problem-solving abilities, and understanding of backend systems. It's not just about proving you can code; it's about demonstrating your capacity to build scalable, efficient, and secure applications. A well-prepared candidate can effectively communicate their knowledge of Java and backend technologies, as well as their ability to contribute to the team and the project's success.
How to do Interview Prep as a Java Backend Developer
- Master Core Java Concepts: Ensure you have a strong grasp of Java fundamentals, including object-oriented programming, design patterns, exception handling, collections, streams, and concurrency. These are often the foundation of technical discussions.
- Understand Frameworks and Technologies: Be familiar with popular Java frameworks and technologies such as Spring (including Spring Boot), Hibernate, and JPA. Understand how they work and their use cases.
- Review Data Structures and Algorithms: Refresh your knowledge of data structures (like trees, graphs, stacks, queues) and algorithms (sorting, searching, recursion). These are common topics in coding interviews.
- Practice Coding Problems: Solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal to sharpen your coding skills and speed. Focus on problems related to backend development.
- Brush Up on System Design: Be prepared to discuss system design principles and demonstrate your ability to design scalable systems. This might include understanding RESTful API design, microservices architecture, and distributed systems.
- Know Your Databases: Understand different types of databases (SQL vs. NoSQL), transactions, indexing, and normalization. Be ready to write and optimize SQL queries.
- Prepare for Behavioral Questions: Reflect on your past experiences and be ready to discuss how you've handled technical challenges, worked in a team, and contributed to project success.
- Demonstrate Version Control Proficiency: Be comfortable with Git or other version control systems, as they are essential tools for any developer.
- Understand DevOps Basics: Familiarize yourself with the basics of DevOps practices, CI/CD pipelines, and tools like Jenkins, Docker, and Kubernetes, as they are increasingly relevant in backend development.
- Prepare Your Own Questions: Develop insightful questions about the company's technology stack, development processes, and culture. This shows your interest in the role and helps you assess if the company is the right fit for you.
- Mock Interviews: Practice with mock interviews, ideally with someone experienced in Java backend development, to get feedback on your technical and communication skills.
By following these steps, you'll be able to enter your Java Backend Developer interview with confidence, ready to demonstrate your technical prowess and your readiness to contribute to the company's backend development efforts.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free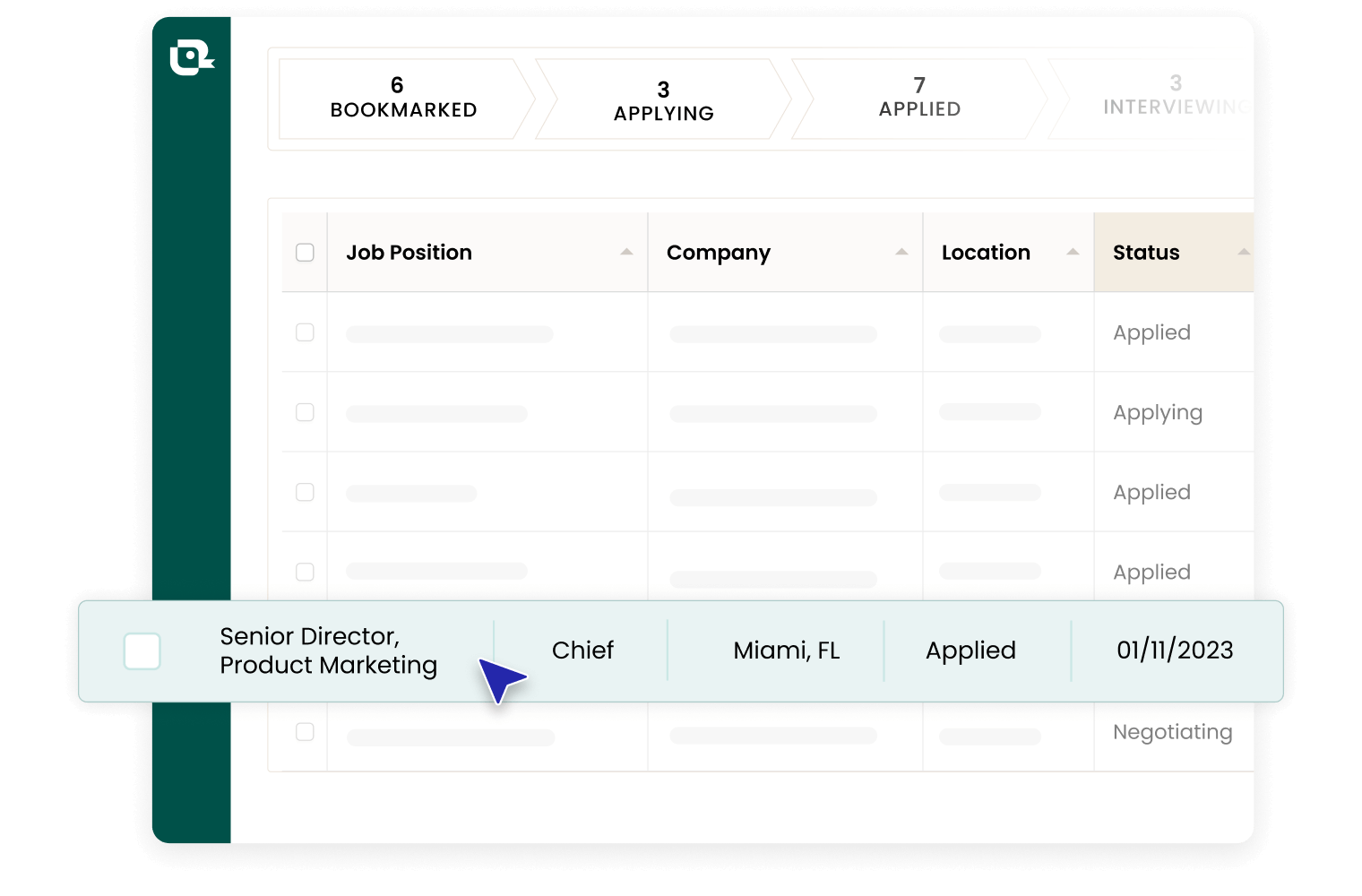
Java Backend Developer Interview Questions and Answers

"What are some of the key principles of Object-Oriented Programming (OOP) and how do you apply them in Java?"
This question assesses your understanding of OOP, a fundamental concept in Java programming. It evaluates your ability to write clean, modular, and reusable code.
How to Answer It
Discuss the four main principles of OOP: encapsulation, abstraction, inheritance, and polymorphism. Provide examples of how you've used these principles in past projects to solve specific problems.
Example Answer
"In OOP, encapsulation allows me to keep the internal state of an object hidden from the outside to prevent unauthorized access. For instance, I use private access modifiers for class attributes and provide public getter and setter methods. Abstraction helps me to define object interfaces, separating the implementation details from the usage. Inheritance enables code reuse by extending existing classes, and polymorphism allows for defining methods in a superclass and overriding them in subclasses to provide specific behaviors. In my last project, I used inheritance to create a hierarchy of shape objects and polymorphism to implement various area calculation methods for each shape."

"How do you manage dependencies in a Java project?"
This question examines your knowledge of build tools and dependency management, which are crucial for maintaining scalable and manageable codebases.
How to Answer It
Explain the tools you use, such as Maven or Gradle, and how you use them to manage project dependencies. Discuss version control and how you ensure compatibility and avoid conflicts.
Example Answer
"I typically use Maven for dependency management in Java projects. I define project dependencies in the pom.xml file, which Maven uses to download the required libraries from the Maven Central Repository. I ensure that the versions of the dependencies are compatible with each other and regularly update them to incorporate security patches and new features. For a recent project, I used Maven profiles to manage dependencies for different deployment environments, which streamlined our build process."

"Can you explain the Java Memory Model and how garbage collection works?"
This question tests your understanding of Java's memory management, which is vital for writing efficient and high-performance applications.
How to Answer It
Describe the Java Memory Model, including the stack and heap, and explain the role of garbage collection. Mention how you monitor and optimize memory usage in your applications.
Example Answer
"The Java Memory Model consists of the heap, where objects are allocated, and the stack, which holds local variables and method call information. Garbage collection in Java automatically removes objects that are no longer referenced, which helps prevent memory leaks. I use tools like VisualVM and profiling in IDEs to monitor heap usage and garbage collection activity. In a past project, I optimized memory usage by minimizing object creation in a critical section of the code, which significantly improved the application's performance."

"How do you ensure thread safety in a Java application?"
This question evaluates your knowledge of concurrency and your ability to write safe, concurrent code in a multi-threaded environment.
How to Answer It
Discuss the strategies you use to prevent race conditions and ensure thread safety, such as synchronization, immutable objects, and concurrent data structures.
Example Answer
"To ensure thread safety, I use various mechanisms depending on the context. For shared resources, I use synchronized blocks or methods to prevent concurrent access. When possible, I design classes to be immutable, which are inherently thread-safe since their state cannot change after creation. Additionally, I leverage concurrent collections from the java.util.concurrent package, which are designed for thread-safe operations. In a recent project, I used a ConcurrentHashMap to manage shared data among threads without explicit synchronization."

"Describe the RESTful web services architecture and how you implement it in Java."
This question probes your understanding of RESTful principles and your experience in developing web services with Java.
How to Answer It
Explain the key concepts of REST, such as statelessness, uniform interface, and resource-oriented architecture. Describe how you use frameworks like Spring Boot or Jersey to create RESTful services.
Example Answer
"RESTful web services are based on REST principles, which use HTTP methods to perform CRUD operations on resources identified by URIs. They are stateless, meaning each request from the client contains all the information needed to process it. In Java, I use Spring Boot to create RESTful services due to its ease of use and comprehensive ecosystem. For example, I use annotations like @RestController, @RequestMapping, and @PathVariable to define resource controllers and map HTTP requests to handler methods."

"What is the Spring Framework, and how do you leverage it in your Java applications?"
This question assesses your experience with the Spring Framework, a widely-used framework for building Java applications.
How to Answer It
Discuss the core features of Spring, such as Dependency Injection and Aspect-Oriented Programming. Give examples of how you've used Spring to simplify application development.
Example Answer
"The Spring Framework simplifies Java development by providing comprehensive infrastructure support. Its core feature, Dependency Injection, allows me to decouple object creation from business logic, making my applications more modular and testable. I also use Spring's Aspect-Oriented Programming to separate cross-cutting concerns like logging and transaction management. In my last project, I used Spring Boot to quickly bootstrap and develop a microservices-based application, taking advantage of its auto-configuration and standalone deployment capabilities."

"How do you handle exceptions in Java?"
This question explores your approach to error handling and your ability to write robust, fault-tolerant code.
How to Answer It
Explain the difference between checked and unchecked exceptions and how you use try-catch blocks, throws declarations, and custom exception classes.
Example Answer
"In Java, checked exceptions must be handled or declared in the method signature, while unchecked exceptions, which are subclasses of RuntimeException, can be optionally handled. I use try-catch blocks to handle exceptions where I can recover or take corrective action. If a method is unable to handle an exception, I propagate it using the throws keyword. For better error granularity, I create custom exception classes. For instance, in a recent project, I implemented a custom ResourceNotFoundException to handle cases where a database query returned no results."

"Explain how you optimize database interactions in a Java application."
This question checks your ability to write efficient database code, which is crucial for backend performance.
How to Answer It
Discuss techniques like connection pooling, prepared statements, batch processing, and the use of ORMs like Hibernate.
Example Answer
"To optimize database interactions, I use connection pooling to reduce the overhead of establishing connections for each request. Prepared statements help prevent SQL injection and improve performance by pre-compiling SQL queries. Batch processing minimizes the number of round trips to the database. I also use Hibernate ORM for object-relational mapping, which allows me to interact with the database using high-level objects rather than SQL queries. Hibernate's caching and lazy loading features help to further optimize database access. In a previous project, these techniques reduced the database load by 30% and improved the overall response time of the application."Which Questions Should You Ask in a Java Backend Developer Interview?
In the competitive field of Java Backend Development, the questions you ask during an interview can be as revealing as the answers you provide. They serve as a testament to your technical acumen, your engagement with the role, and your strategic thinking. For Java Backend Developers, the inquiries made should not only impress the interviewer by highlighting your depth of knowledge and interest in the company's technical challenges but also serve your own interests in determining if the position aligns with your career objectives and values. By asking incisive questions, you position yourself as a discerning candidate, one who is not just looking for any job, but the right job where you can thrive and contribute significantly.
Good Questions to Ask the Interviewer
"Could you elaborate on the current architecture of the backend systems and the rationale behind the chosen technologies?"
This question underscores your technical expertise and curiosity about the company's tech stack. It indicates that you're considering how you might integrate with or enhance their current systems, and it gives you insight into the company's technological decision-making processes.
"What are the most significant challenges your backend team is facing, and how do you anticipate a new hire would address these?"
Asking this demonstrates your willingness to engage with the company's challenges and shows that you're already thinking about how you can contribute to solutions. It also provides a window into the team's current dynamics and the expectations placed on new team members.
"How does the company approach code quality, testing, and technical debt?"
This question reflects your commitment to best practices in software development and your interest in the company's standards for maintainability and scalability. It can also reveal how the organization balances short-term results with long-term system health.
"Can you describe the process for deploying new features and how backend developers collaborate with other teams?"
Inquiring about deployment processes and cross-team collaboration highlights your understanding of the full development lifecycle and your readiness to work within a team environment. This question can also shed light on the company's approach to DevOps and Agile methodologies.
By asking these targeted questions, you not only demonstrate your technical and strategic prowess but also gain valuable insights that will help you determine if the role is the right fit for your career trajectory.
What Does a Good Java Backend Developer Candidate Look Like?
In the realm of Java backend development, a standout candidate is one who not only possesses a deep understanding of Java and its associated technologies but also exhibits a blend of problem-solving prowess, architectural insight, and collaborative spirit. Employers and hiring managers are on the lookout for individuals who can not only write efficient, maintainable code but also contribute to the design and optimization of scalable systems. A good Java backend developer is expected to be proactive, with a keen eye for identifying and resolving system bottlenecks, and to work effectively within a team to integrate complex software components into a cohesive backend solution.
A strong candidate will demonstrate a balance between technical acumen and soft skills, ensuring they can navigate the nuances of backend development while effectively communicating with team members and stakeholders. They are expected to be adaptable, continuously learning and evolving with the technological landscape, and to approach development with a security-conscious and performance-oriented mindset.
Technical Proficiency in Java and Related Technologies
A good candidate has a robust grasp of Java, including its core APIs, frameworks like Spring and Hibernate, and an understanding of JVM internals. They should be adept at using build tools like Maven or Gradle and version control systems such as Git.
System Design and Architecture Knowledge
Understanding the principles of clean, scalable system design is crucial. This includes knowledge of design patterns, microservices architecture, and the ability to design RESTful APIs that meet the needs of various client applications.
Database and Cache Management
Proficiency in database technologies, both SQL and NoSQL, is essential. A good candidate should also understand caching strategies and how to implement them to improve application performance.
Problem-Solving and Analytical Skills
The ability to troubleshoot and resolve complex technical issues is highly valued. This includes a systematic approach to debugging and the use of profiling tools to optimize performance.
Understanding of DevOps Practices
Familiarity with DevOps culture and practices, including continuous integration and deployment (CI/CD), containerization with Docker, and orchestration with Kubernetes, is becoming increasingly important.
Collaboration and Communication
Effective communication skills are vital for a backend developer. They should be able to articulate technical concepts to non-technical stakeholders and collaborate with front-end developers, designers, and product managers to deliver a cohesive product.
Security Awareness
A strong candidate is always vigilant about security, understanding common vulnerabilities in web applications and how to defend against them. They should be familiar with security best practices and frameworks such as OWASP.
By embodying these qualities, a Java backend developer candidate can position themselves as a valuable asset to any development team, capable of tackling the challenges of modern backend systems and contributing to the creation of robust, scalable, and secure web applications.
Interview FAQs for Java Backend Developers
What is the most common interview question for Java Backend Developers?
"How do you ensure the scalability of a Java application?" This question probes your architectural acumen and understanding of system design principles. A compelling response should highlight your experience with Java concurrency, efficient data structures, caching strategies, and load balancing. It's essential to mention your familiarity with profiling and optimization tools, as well as your ability to leverage frameworks and design patterns that promote scalability, such as microservices or reactive programming.
What's the best way to discuss past failures or challenges in a Java Backend Developer interview?
To demonstrate problem-solving skills in a Java Backend Developer interview, recount a complex coding issue you resolved. Detail your systematic debugging process, the tools and methodologies you employed, and how you optimized the code for efficiency. Emphasize your adaptability in using different Java frameworks or libraries and the positive outcome, such as enhanced performance or scalability, underscoring your technical acumen and solution-oriented mindset.
How can I effectively showcase problem-solving skills in a Java Backend Developer interview?
To demonstrate problem-solving skills in a Java Backend Developer interview, recount a complex coding issue you resolved. Detail your systematic debugging process, the tools and methodologies you employed, and how you optimized the code for efficiency. Emphasize your adaptability in using different Java frameworks or libraries and the positive outcome, such as enhanced performance or scalability, underscoring your technical acumen and solution-oriented mindset.
Up Next
Java Backend Developer Job Title Guide
Copy Goes Here.
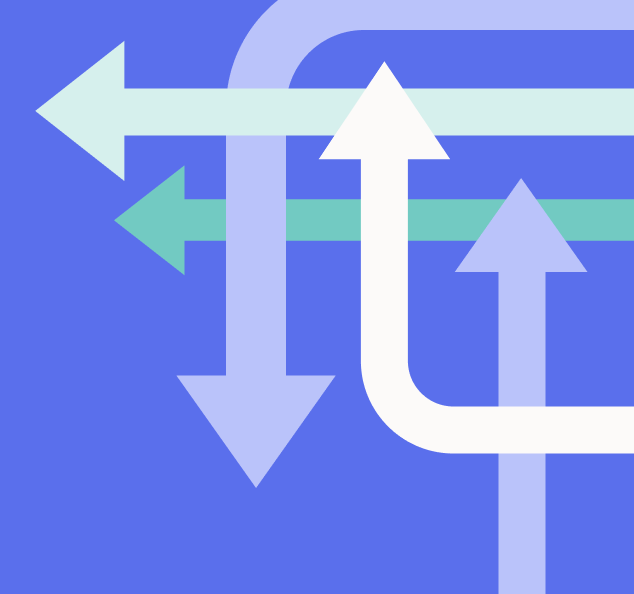