Interviewing as a PHP Developer
Navigating the path to becoming a PHP Developer is an exciting journey, punctuated by the pivotal moment of the job interview. In this high-stakes conversation, you're not only showcasing your technical prowess with PHP but also demonstrating your problem-solving skills, adaptability, and potential for growth within a development team.
Our comprehensive guide is tailored to demystify the PHP Developer interview process. We delve into the array of questions you might encounter, from the intricacies of PHP-specific technical challenges to broader inquiries about your development philosophy and collaborative style. We'll equip you with the insights to craft compelling responses, strategies for effective preparation, and the critical questions to pose to your prospective employers. This guide is your ally, designed to sharpen your interview acumen and elevate your candidacy in the competitive field of PHP development.
Types of Questions to Expect in a PHP Developer Interview
PHP Developer interviews are designed to probe not only your technical knowledge but also your problem-solving abilities, communication skills, and overall fit for the developer role. Understanding the various types of questions you may encounter can help you prepare effectively and demonstrate your full range of abilities. Here's an overview of the key question categories to help you navigate the interview process with confidence.
Technical Proficiency Questions
Technical questions form the backbone of a PHP Developer interview, as they directly assess your coding skills and understanding of PHP concepts. Expect to answer questions about PHP syntax, functions, error handling, and best practices. These questions may include writing code on the spot, explaining the output of a given code snippet, or discussing the nuances of PHP 7 versus PHP 8. They are intended to evaluate your expertise and comfort level with the language.
Conceptual Understanding Questions
Beyond syntax, you'll be asked about your grasp of underlying concepts such as object-oriented programming (OOP), design patterns, and software architecture. These questions might cover topics like inheritance, polymorphism, MVC frameworks, and the SOLID principles. The goal is to understand how deeply you comprehend these concepts and can apply them to build scalable and maintainable applications.
Database and Web Technologies Questions
PHP is often used in conjunction with databases and other web technologies, so expect questions about SQL, data modeling, and interactions with MySQL or other database systems. You may also be asked about your experience with front-end technologies, RESTful APIs, and web services. These questions assess your ability to integrate PHP with various components of a web application and handle data effectively.
Problem-Solving and Debugging Questions
Problem-solving questions test your analytical skills and ability to troubleshoot issues. You might be given a bug-ridden code snippet to debug or a scenario requiring a logical solution. These questions gauge your critical thinking, attention to detail, and persistence in resolving complex programming challenges.
Behavioral and Communication Questions
Interpersonal skills are crucial for a PHP Developer, as you'll often work in teams and communicate with stakeholders. Behavioral questions explore your past experiences, work ethic, and how you handle conflict or stress. You may be asked to describe a difficult project, how you managed deadlines, or collaborated with non-technical team members. These questions aim to uncover your soft skills and cultural fit within the organization.
Performance and Security Questions
With the importance of web application performance and security, you may face questions about optimizing PHP code, caching strategies, and securing applications against common vulnerabilities. These questions test your awareness of performance trade-offs and your proactive approach to security in the development process.
By familiarizing yourself with these question types and reflecting on your experiences and knowledge, you can approach a PHP Developer interview with the assurance that you're ready to showcase your qualifications effectively. Tailoring your preparation to these categories can help you articulate your responses in a way that aligns with the role's expectations.
Preparing for a PHP Developer Interview
Preparing for a PHP Developer interview is a critical step in showcasing your technical abilities, problem-solving skills, and understanding of web development concepts. It's not just about proving your coding prowess; it's about demonstrating your capacity to contribute effectively to a development team, understand project requirements, and deliver robust, maintainable code. A well-prepared candidate stands out by displaying a deep understanding of PHP and related technologies, as well as the ability to communicate effectively and adapt to the specific needs of the hiring company.
How to do Interview Prep as a PHP Developer
- Review PHP Fundamentals: Ensure you have a strong grasp of PHP syntax, data types, functions, and object-oriented programming concepts. Be prepared to write code on the spot or explain how you would solve specific problems using PHP.
- Understand the Full Stack: Familiarize yourself with the entire web development stack that PHP interacts with, including HTML, CSS, JavaScript, and SQL. Know how PHP fits within the client-server model and be ready to discuss web technologies like AJAX and JSON.
- Study Frameworks and Libraries: If the job description mentions specific frameworks like Laravel or Symfony, make sure you understand their structure, conventions, and benefits. Be able to articulate why and when you would use a particular framework over plain PHP.
- Practice with Real-World Scenarios: Be ready to discuss past projects and how you addressed various challenges. Prepare to solve real-world problems that you might encounter in the role, such as optimizing performance, handling security issues, or managing data persistence.
- Brush Up on Development Tools: Familiarize yourself with the tools and environments used in PHP development, such as Composer, PHPUnit, Docker, and version control systems like Git. Be able to explain your workflow and how you use these tools to improve code quality and collaboration.
- Prepare for Behavioral Questions: Reflect on your experiences to share examples of how you've worked in teams, dealt with tight deadlines, or continued learning new technologies. Employers are looking for developers who are not only technically proficient but also fit well within their team culture.
- Know the Latest PHP Trends: Stay updated on the latest PHP versions and features, as well as current best practices in the industry. This shows your commitment to keeping your skills relevant and your interest in the evolution of PHP.
- Develop Questions for the Interviewer: Prepare thoughtful questions about the company's development practices, technology stack, and culture. This demonstrates your genuine interest in the role and helps you assess if the company is the right fit for you.
- Conduct Mock Interviews: Practice answering questions and discussing your work with a peer or mentor. This can help you refine your communication skills and receive constructive feedback on your technical explanations.
By following these steps, you'll enter your PHP Developer interview with the confidence that comes from thorough preparation. You'll be ready to engage in detailed discussions about your technical skills, past experiences, and how you can contribute to the success of the company's projects.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free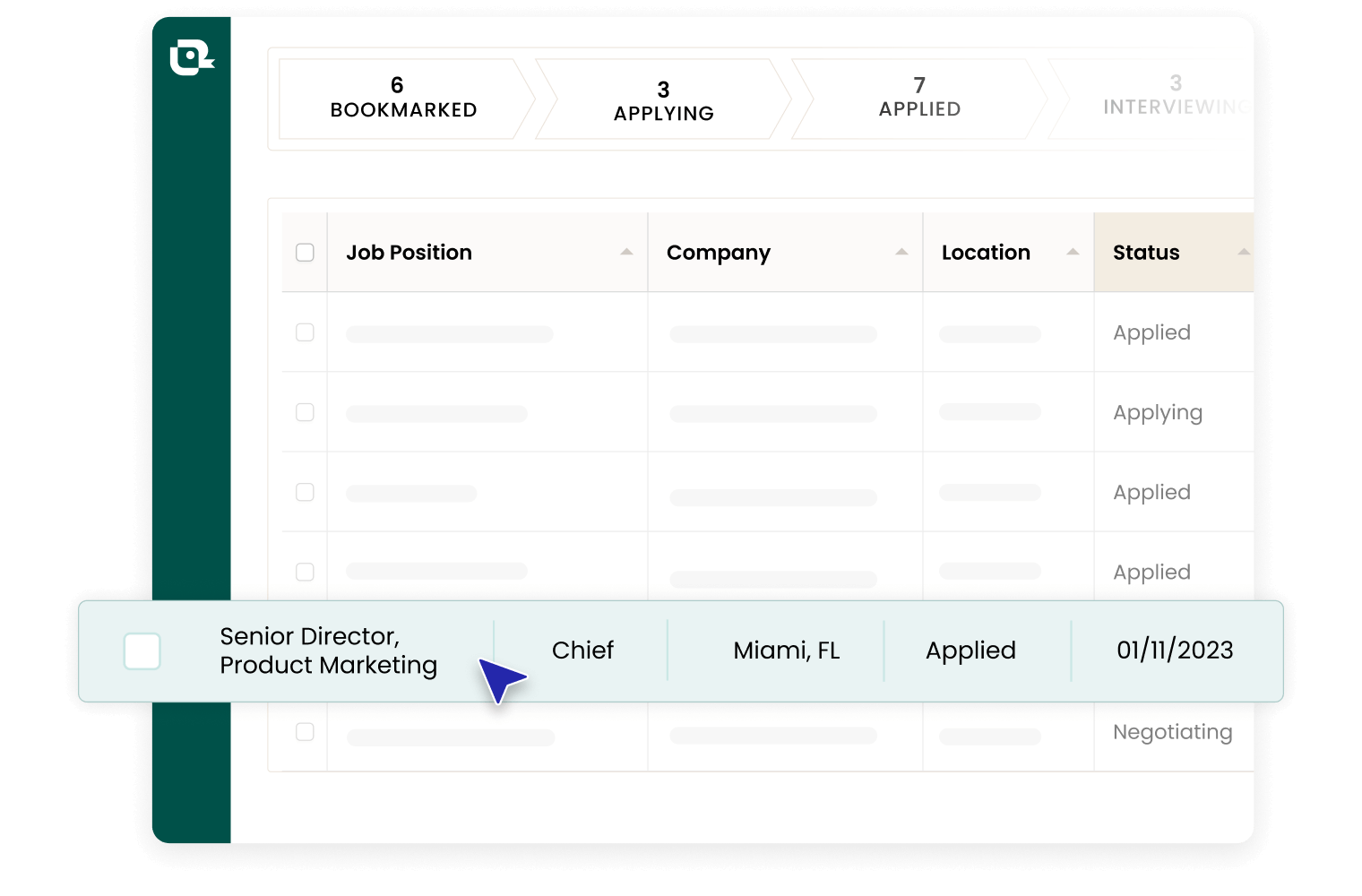
PHP Developer Interview Questions and Answers

"What are some of the major changes in PHP 7 compared to previous versions?"
This question assesses your knowledge of PHP's evolution and your ability to adapt to new features and performance improvements.
How to Answer It
Discuss the key improvements introduced in PHP 7, such as speed, type declarations, error handling, and new operators. Show that you're up to date with the language's development.
Example Answer
"In PHP 7, significant performance enhancements were introduced, including the new Zend Engine 3.0, which roughly doubled the speed of its predecessor. PHP 7 also added scalar type declarations and return type declarations, which help with code quality and readability. The null coalescing operator and spaceship operator are also notable additions. Furthermore, error handling was improved with the introduction of Throwable exceptions."

"How do you manage state in a PHP application?"
This question evaluates your understanding of state management and your ability to handle user sessions and data persistence.
How to Answer It
Explain the mechanisms PHP provides for state management, such as sessions, cookies, and databases. Describe a scenario where you implemented a secure and efficient state management system.
Example Answer
"In PHP, state management can be handled using sessions and cookies. For user authentication, I typically use sessions because they are stored server-side and are more secure. I ensure that session data is stored in a secure location and that session IDs are regenerated upon login to prevent session hijacking. For persistent data that needs to be accessed across sessions, I use a database, implementing proper data sanitization and validation to prevent SQL injection attacks."

"Can you explain the MVC architecture and how it's implemented in PHP frameworks?"
This question tests your understanding of software design patterns and your experience with PHP frameworks that utilize MVC architecture.
How to Answer It
Describe the Model-View-Controller (MVC) architecture and its components. Give an example of a PHP framework you've worked with that follows MVC principles.
Example Answer
"MVC stands for Model-View-Controller, a design pattern that separates an application into three interconnected components. The Model represents the data and business logic, the View is the output representation of the data, and the Controller handles input and converts it to commands for the Model or View. In PHP, frameworks like Laravel and Symfony implement MVC to encourage good coding practices and maintainable code. In my last project, I used Laravel, which streamlined development with its elegant syntax and powerful ORM."

"How do you ensure security in PHP applications?"
This question gauges your awareness of security best practices and your ability to safeguard PHP applications against common vulnerabilities.
How to Answer It
Discuss various security threats such as SQL injection, XSS, and CSRF, and the strategies you employ to mitigate these risks in your PHP applications.
Example Answer
"To ensure security in PHP applications, I follow several best practices. I use prepared statements with PDO to prevent SQL injection. For XSS, I escape output and use content security policies. To protect against CSRF, I implement anti-CSRF tokens in forms. Additionally, I keep PHP and its libraries up to date, use strong hashing algorithms for passwords, and implement proper error handling to avoid revealing sensitive information."

"What is object-oriented programming in PHP, and can you give an example of when you've used it?"
This question examines your understanding of OOP concepts in PHP and your ability to apply them in real-world scenarios.
How to Answer It
Explain the principles of object-oriented programming and how PHP supports OOP. Provide an example of a project where OOP made your code more reusable and maintainable.
Example Answer
"Object-oriented programming in PHP allows developers to create classes, which are blueprints for objects, and to encapsulate data and functions that operate on that data. PHP supports key OOP features like inheritance, interfaces, and polymorphism. In a recent e-commerce project, I used OOP to create a Product class, which served as a base for more specific product types. This approach allowed for code reuse and easier maintenance as the project evolved."

"How do you handle error and exception handling in PHP?"
This question assesses your ability to write robust PHP code that can gracefully handle unexpected conditions.
How to Answer It
Discuss the use of PHP's built-in error handling functions and exception handling with try-catch blocks. Mention how you log errors and monitor for issues in production environments.
Example Answer
"In PHP, I handle errors by setting an error handler function using seterrorhandler() to process runtime errors. For exceptions, I use try-catch blocks to catch exceptions and handle them appropriately. I also use finally blocks to ensure that any necessary cleanup is performed. In production, I log errors to a file or a monitoring system like Sentry, which helps me to quickly identify and address issues."

"What are some common performance issues in PHP applications and how do you optimize them?"
This question explores your ability to identify bottlenecks and improve the efficiency of PHP applications.
How to Answer It
Describe common performance pitfalls such as database query inefficiencies or memory leaks, and explain the strategies you use to optimize PHP code.
Example Answer
"Common performance issues in PHP applications include inefficient database queries, excessive memory usage, and slow functions. To optimize these, I use query optimization techniques like indexing and avoiding N+1 problems by eager loading related data. I also profile the application using tools like Xdebug to identify memory leaks and slow functions. Implementing caching with solutions like Redis or Memcached can also significantly improve performance by reducing the load on the database."

"Describe your experience with Composer and Packagist in PHP development."
This question checks your familiarity with PHP's package management system and your ability to manage dependencies in a project.
How to Answer It
Talk about how you've used Composer and Packagist to manage third-party packages and handle autoloading in PHP projects.
Example Answer
"In my PHP projects, I use Composer as a dependency manager to streamline the installation and updating of third-party packages from Packagist. It allows me to define the required libraries for a project and ensures that all team members are working with the same versions. Composer's autoloading capabilities also simplify namespace management and class loading. For example, in a recent project, I used Composer to manage dependencies like Guzzle for HTTP requests and PHPUnit for testing, which improved our development workflow and ensured consistency across our development environments."Which Questions Should You Ask in a PHP Developer Interview?
In the competitive field of PHP development, the questions you ask during an interview are as crucial as the technical expertise you bring to the table. They serve a dual purpose: showcasing your analytical skills and genuine interest in the role, while also helping you to determine if the position aligns with your career goals and values. For PHP Developers, the inquiries made can reflect your understanding of the company's technical stack, your eagerness to engage with their development practices, and your potential fit within the team. By asking insightful questions, you not only leave a positive impression on your potential employers but also take an active role in assessing whether the opportunity is conducive to your professional growth and satisfaction.
Good Questions to Ask the Interviewer
"Can you describe the current PHP projects the development team is working on and the technologies involved?"
This question demonstrates your interest in the company's PHP projects and gives you insight into the technical environment you might be working in. It also allows you to gauge the complexity and scope of the projects, helping you understand if they match your skills and interests.
"How does the team manage code quality and what practices are in place for code reviews and testing?"
Asking about code quality management shows that you care about best practices and are proactive about maintaining high standards. This question can also reveal the company's commitment to technical excellence and whether they have a culture of continuous improvement that aligns with your own professional standards.
"What opportunities are available for professional development and career advancement for PHP Developers within the company?"
This question indicates your ambition and desire for growth, and it helps you understand if the company supports skill enhancement and career progression. It's important to know if you can evolve as a professional in the organization and if there are clear pathways to advance your career.
"Could you share how the development team collaborates with other departments, such as product management and design?"
Understanding the cross-functional dynamics of the company is crucial for a PHP Developer. This question can shed light on the collaborative culture of the organization and how integrated the development process is with other aspects of product creation, which is essential for delivering cohesive and effective software solutions.
What Does a Good PHP Developer Candidate Look Like?
In the realm of web development, PHP developers play a critical role in crafting dynamic and interactive websites. A good PHP developer candidate is not only proficient in PHP and its associated technologies but also demonstrates a deep understanding of web architecture and database design. Employers and hiring managers are on the lookout for candidates who are problem solvers, can write clean and maintainable code, and possess a solid grasp of both front-end and back-end development practices.
A strong PHP developer candidate is someone who can balance technical know-how with practical application, ensuring that the websites and applications they develop are both efficient and user-friendly. They are expected to be agile, adaptable, and collaborative, able to integrate seamlessly with cross-functional teams and contribute to the overall success of projects.
Technical Proficiency
A good candidate has a comprehensive understanding of PHP programming, including knowledge of various PHP frameworks like Laravel or Symfony. They should be adept at using version control systems like Git and have experience with API integration and database technologies such as MySQL.
Problem-Solving and Analytical Skills
The ability to troubleshoot and debug complex issues is essential. A good PHP developer candidate should have strong analytical skills and be able to approach problems methodically to find efficient solutions.
Understanding of Web Technologies
A well-rounded PHP developer should be familiar with front-end technologies like HTML, CSS, and JavaScript, as well as understand the principles of responsive design and web security practices.
Effective Communication
Clear communication is key in a collaborative environment. Candidates should be able to articulate technical concepts to non-technical stakeholders and work effectively within a team.
Adaptability and Continuous Learning
The tech field is ever-evolving, and a good PHP developer must be willing to learn and adapt to new technologies and methodologies to stay relevant and effective.
Attention to Detail
Quality code requires meticulous attention to detail. Candidates should demonstrate the ability to write clean, well-documented code that follows industry standards and best practices.
By embodying these qualities, a PHP developer candidate can stand out to employers as a valuable asset who is capable of delivering robust, scalable, and secure web applications that meet the needs of users and businesses alike.
Interview FAQs for PHP Developers
What is the most common interview question for PHP Developers?
"How do you manage state in a PHP application?" This question probes your understanding of session management and data persistence, crucial for web application functionality. A well-rounded response should highlight your knowledge of PHP sessions, cookies, and database-driven state management, while also considering security practices like session hijacking prevention and proper use of secure cookies. It's an opportunity to showcase your technical proficiency and awareness of best practices in maintaining user state across different requests in PHP.
What's the best way to discuss past failures or challenges in a PHP Developer interview?
To exhibit problem-solving skills in a PHP Developer interview, detail a complex coding challenge you faced. Explain your methodical approach, how you dissected the problem, and the innovative coding solutions you implemented. Highlight your use of PHP frameworks or libraries, collaboration with other developers, and the positive outcomes, such as enhanced functionality or performance improvements. This underscores not just your technical prowess but also your systematic and collaborative problem-solving methodology.
How can I effectively showcase problem-solving skills in a PHP Developer interview?
To exhibit problem-solving skills in a PHP Developer interview, detail a complex coding challenge you faced. Explain your methodical approach, how you dissected the problem, and the innovative coding solutions you implemented. Highlight your use of PHP frameworks or libraries, collaboration with other developers, and the positive outcomes, such as enhanced functionality or performance improvements. This underscores not just your technical prowess but also your systematic and collaborative problem-solving methodology.
Up Next
PHP Developer Job Title Guide
Copy Goes Here.
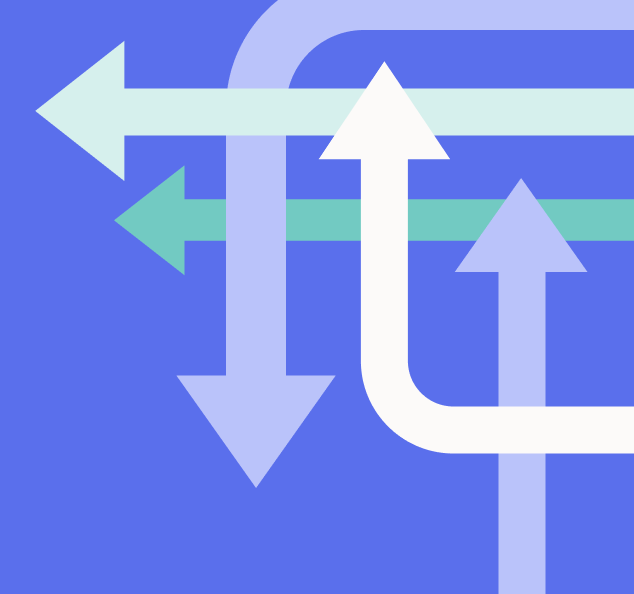