Interviewing as a iOS Developer
Navigating the landscape of iOS Developer interviews can be as intricate as the code you write. These interviews are more than a test of your technical prowess; they are a showcase of your innovative thinking, adaptability to new technologies, and your commitment to creating seamless user experiences.
In this guide, we'll dissect the array of questions that iOS Developers are likely to encounter, from the intricacies of Swift syntax to the complexities of app lifecycle and memory management. We'll provide you with the framework for crafting responses that resonate with technical depth and strategic insight. Moreover, we'll equip you with the knowledge to discern what interviewers are truly asking, how to demonstrate your value as a candidate, and the critical questions to pose to your potential employers. This guide is your blueprint to mastering the iOS Developer interview, positioning you to excel and propel your career forward.
Types of Questions to Expect in a iOS Developer Interview
iOS Developer interviews are designed to probe not only your technical expertise but also your problem-solving abilities and cultural fit within a team. The questions you'll encounter are crafted to assess a range of skills, from your coding proficiency to your understanding of design principles and user experience. By familiarizing yourself with the types of questions outlined below, you can approach your interview with confidence, ready to demonstrate the depth of your knowledge and your readiness to contribute to the development team.
Technical Proficiency Questions
Technical questions form the backbone of any iOS Developer interview. These questions will test your knowledge of Swift, Objective-C, and other relevant programming languages. You may be asked to write code on the spot, explain the reasoning behind your coding choices, or debug a piece of code. These questions assess your core skills and your ability to write clean, efficient, and maintainable code.
Framework and Tools Familiarity Questions
iOS development relies heavily on Apple's frameworks and tools such as UIKit, Core Data, and Xcode. Interviewers will ask about your experience with these to gauge your ability to build complex applications. You might be asked to describe how you've implemented certain features using these frameworks or to discuss the pros and cons of different development tools.
Design and Architecture Questions
Understanding design patterns and architecture is crucial for creating robust and scalable iOS applications. Expect questions on MVC, MVVM, and other design patterns, as well as discussions on how to structure an app's architecture. These questions evaluate your ability to design systems that are both efficient and easy to maintain.
Behavioral Questions
Behavioral questions aim to understand how you work within a team, handle pressure, and adapt to change. You might be asked about past projects, how you've dealt with conflicts, or what you do to stay updated with the latest iOS developments. These questions look for evidence of your soft skills, such as communication, teamwork, and time management.
Problem-Solving and Algorithm Questions
These questions test your logical thinking and problem-solving skills. You may be presented with hypothetical problems or algorithmic challenges that require you to think critically and articulate your thought process. They assess your ability to tackle complex issues and come up with effective solutions.
Practical Application and Coding Challenges
To assess your hands-on skills, you may be given coding challenges or asked to work on a small project. These exercises demonstrate your practical ability to apply your knowledge to real-world scenarios. They also show how you approach development tasks, manage your time, and debug your own code.
Preparing for these question types will not only help you to better understand what to expect but also allow you to present a well-rounded picture of your capabilities as an iOS Developer. Tailoring your study and practice to these areas can make a significant difference in your interview performance, showcasing your readiness to tackle the challenges of the role.
Preparing for a iOS Developer Interview
Preparing for an iOS Developer interview requires a blend of technical prowess, understanding of design principles, and the ability to communicate effectively. As an iOS Developer, you're not just coding; you're crafting experiences for millions of users. Therefore, it's essential to demonstrate not only your coding skills but also your understanding of user interface design, performance optimization, and the Apple ecosystem. A well-prepared candidate stands out by showing a deep knowledge of iOS frameworks, a problem-solving mindset, and a passion for building intuitive and efficient applications.
How to do Interview Prep as an iOS Developer
- Master the Fundamentals: Ensure you have a strong grasp of Swift or Objective-C, and understand core concepts like memory management, concurrency, and error handling. Review the latest iOS SDK and Apple's Human Interface Guidelines.
- Understand iOS-specific Technologies: Be familiar with essential frameworks and APIs such as UIKit, Core Data, Core Animation, and more. Know how to work with RESTful APIs and understand networking concepts within the context of an iOS app.
- Review Your Past Projects: Be ready to discuss your previous work, challenges you faced, and how you overcame them. Highlight any unique solutions or optimizations you implemented.
- Practice Coding Challenges: Sharpen your problem-solving skills with coding challenges on platforms like LeetCode or HackerRank, focusing on algorithms and data structures that are commonly used in mobile app development.
- Prepare for System Design Questions: Understand how to design scalable systems and be prepared to discuss architecture choices for hypothetical app scenarios, considering performance, maintainability, and user experience.
- Stay Updated on Apple's Ecosystem: Show that you're keeping up with the latest developments in the iOS world, including new features in the latest version of iOS, Swift language updates, and any changes to the App Store guidelines.
- Mock Interviews: Practice with mock interviews that focus on technical questions, system design, and behavioral questions. Use feedback to refine your answers and presentation skills.
- Prepare Your Portfolio: Have your portfolio of apps ready, ideally with links to the App Store or GitHub. Be prepared to explain your design and development process for each project.
- Develop Questions to Ask: Prepare insightful questions about the company's tech stack, development processes, and culture. This shows your interest in the role and helps you assess if the company is the right fit for you.
By following these steps, you'll not only be ready to tackle the technical aspects of the interview but also demonstrate a comprehensive understanding of the iOS platform and an eagerness to contribute to the company's projects. Your preparation will help you to confidently articulate your skills and experiences, making a strong impression on your potential employer.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free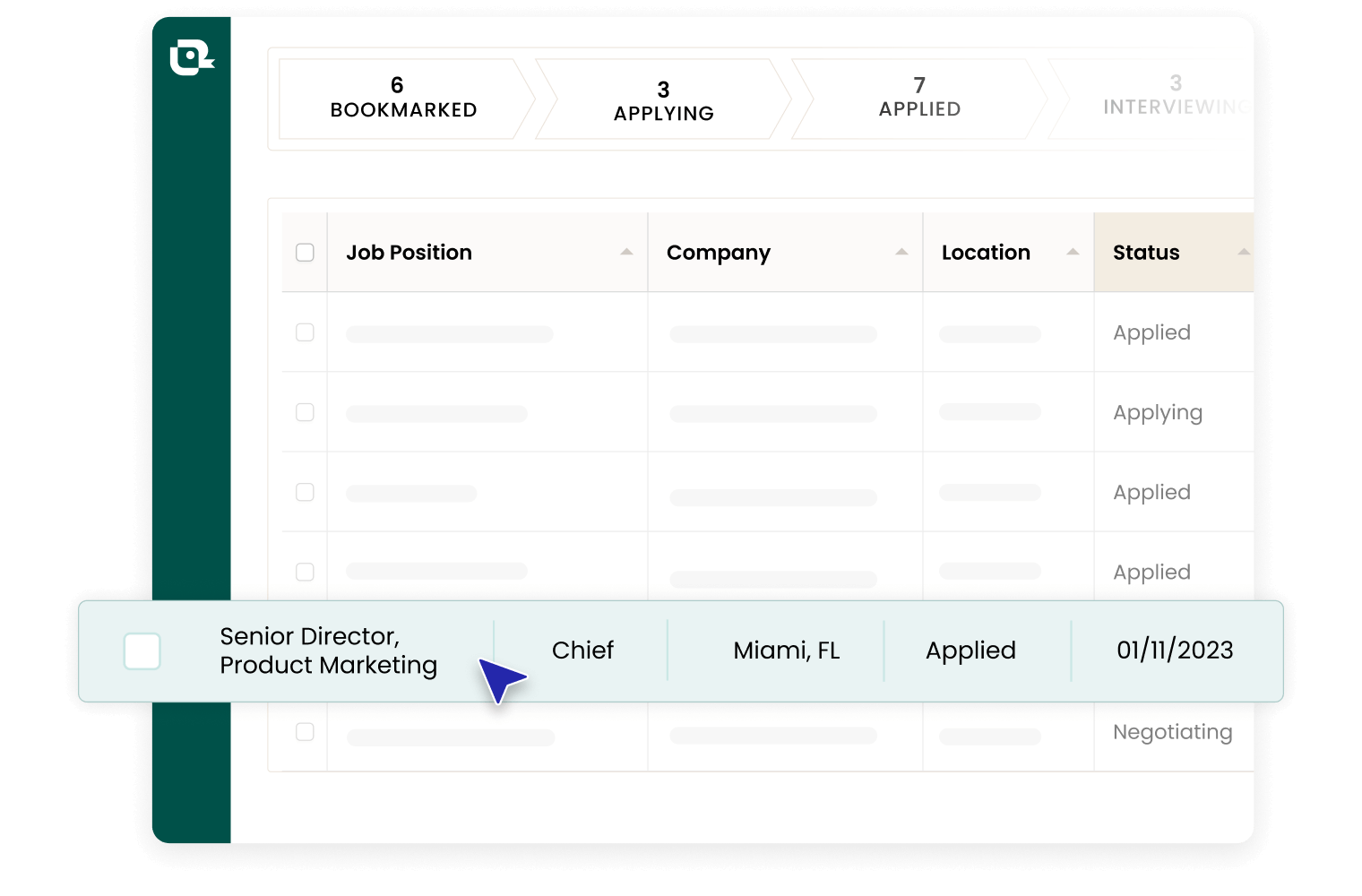
iOS Developer Interview Questions and Answers

"What are some key considerations when designing an app for multiple iOS devices?"
This question evaluates your understanding of the iOS ecosystem and your ability to create apps that provide a consistent user experience across various devices.
How to Answer It
Discuss the use of Auto Layout and Size Classes for responsive design, asset management with @1x, @2x, and @3x resolutions, and the importance of testing on different devices.
Example Answer
"In my previous projects, I've prioritized ensuring that apps look and function well on all devices. I use Auto Layout and Size Classes to manage the user interface, ensuring it adapts to different screen sizes and orientations. I also carefully manage assets to support various resolutions and regularly test on actual devices to guarantee a seamless user experience."

"How do you manage memory in an iOS app?"
This question probes your understanding of memory management and your ability to optimize app performance.
How to Answer It
Explain the concepts of Automatic Reference Counting (ARC), memory leaks, and the use of Instruments to track and resolve memory issues.
Example Answer
"I manage memory by leveraging ARC, which automatically handles most of the memory management work. However, I stay vigilant for retain cycles that can cause memory leaks. I use Instruments to profile the app's memory usage and address any leaks or excessive memory consumption to ensure optimal performance."

"Can you explain the Model-View-Controller (MVC) pattern and its importance in iOS development?"
This question assesses your knowledge of software design patterns and their practical application in iOS apps.
How to Answer It
Describe the MVC components and their roles, and explain how this pattern helps in organizing code and improving maintainability.
Example Answer
"MVC is fundamental in iOS development. It separates data (Model), user interface (View), and the business logic (Controller). This separation facilitates code reusability and testing. For example, in my last app, I used MVC to isolate the network code from the UI code, which simplified debugging and allowed for more flexible code changes."

"Describe your experience with multithreading in iOS."
This question examines your ability to write efficient, responsive iOS applications that handle concurrent operations.
How to Answer It
Discuss your familiarity with technologies like Grand Central Dispatch (GCD) and NSOperationQueue, and provide examples of how you've used them to improve app performance.
Example Answer
"In my experience, multithreading is crucial for maintaining a smooth user experience. I've used GCD and NSOperationQueue to perform complex computations and network calls in the background, preventing the UI from freezing. For instance, in a photo editing app, I used GCD to apply filters asynchronously, ensuring the UI remained responsive."

"How do you ensure the security of user data in an iOS app?"
This question tests your knowledge of security best practices in iOS development.
How to Answer It
Discuss encryption, secure data storage with Keychain, and using HTTPS for network communications. Mention any relevant security frameworks you've worked with.
Example Answer
"Security is paramount. I use Keychain to store sensitive user data securely and ensure all network communications are over HTTPS. For added security, I implement data encryption using the CommonCrypto library. In a recent project, I also integrated Touch ID and Face ID for secure authentication."

"What is your approach to testing and quality assurance in iOS app development?"
This question explores your commitment to delivering high-quality, reliable applications.
How to Answer It
Explain your use of unit tests, UI tests, and manual testing. Discuss the importance of test-driven development (TDD) or behavior-driven development (BDD) if you use them.
Example Answer
"I take a comprehensive approach to testing, starting with TDD to ensure each component functions correctly. I write unit tests for logic-heavy parts and UI tests to automate user interaction scenarios. Additionally, I conduct thorough manual testing, especially for edge cases that automated tests might miss."

"How do you handle asynchronous tasks in iOS development?"
This question assesses your understanding of asynchronous programming and its impact on app performance and user experience.
How to Answer It
Discuss the use of closures, delegates, and notification patterns to handle asynchronous events. Mention any modern approaches like Combine or async/await if applicable.
Example Answer
"I handle asynchronous tasks by using closures and delegates to receive callbacks when tasks complete. For more complex scenarios, I've started using the Combine framework for its declarative approach to handling asynchronous events. In my latest project, I've also adopted Swift's async/await syntax to write cleaner, more readable asynchronous code."

"Can you discuss a time when you optimized an iOS app's performance?"
This question allows you to demonstrate your problem-solving skills and your ability to enhance app efficiency.
How to Answer It
Provide a specific example of performance issues you've encountered and describe the steps you took to diagnose and resolve them.
Example Answer
"In a previous role, I noticed our app had slow launch times. Using the Time Profiler in Instruments, I identified that excessive disk I/O was the culprit during startup. I optimized data loading by implementing lazy loading and caching strategies, which reduced the launch time by 50%."Which Questions Should You Ask in a iOS Developer Interview?
In the competitive field of iOS development, the questions you ask in an interview can be as revealing as the answers you provide. They not only demonstrate your technical acumen and passion for the craft but also your foresight in assessing whether the role aligns with your career trajectory. For iOS Developers, asking insightful questions can convey your depth of understanding of the iOS platform, your commitment to staying abreast of technological advancements, and your ability to fit within the team's culture. Moreover, these questions enable you to actively participate in the interview process, transforming it into a two-way conversation where you can evaluate the company's practices, expectations, and environment to determine if they match your professional goals and values.
Good Questions to Ask the Interviewer
"Can you describe the current tech stack for iOS development within the company and how it has evolved?"
This question not only shows your interest in the company's technology choices but also gives you insight into how progressive and adaptable the organization is with respect to new tools and frameworks. It can also hint at potential learning opportunities or challenges you might face.
"How does the team manage code quality and what practices are in place for testing and review?"
Asking about code quality and testing practices reveals your commitment to high standards and your understanding of the importance of these processes in delivering a robust application. It also helps you understand the company's approach to quality assurance and continuous integration.
"What is the process for app design and development collaboration between iOS Developers, designers, and product managers?"
This question can shed light on the company's workflow and how cross-functional teams interact. It indicates your willingness to engage in a collaborative environment and your desire to understand how your role as an iOS Developer fits into the larger product development lifecycle.
"How does the company stay updated with the latest iOS updates and incorporate new features into existing apps?"
Inquiring about the company's approach to keeping up with iOS updates demonstrates your awareness of the platform's evolving nature. It also helps you gauge how the company balances maintenance with innovation, and whether they provide time and resources for developers to learn and implement new technologies.
What Does a Good iOS Developer Candidate Look Like?
In the realm of iOS development, a standout candidate is one who not only possesses a strong grasp of technical skills, such as proficiency in Swift and a deep understanding of the iOS ecosystem, but also exhibits a blend of creativity, problem-solving abilities, and a passion for crafting user-centric applications. Employers and hiring managers are on the lookout for candidates who can demonstrate a comprehensive approach to app development, encompassing code quality, performance optimization, and an intuitive user interface design. A good iOS developer candidate is someone who is not only technically adept but also thrives in collaborative environments, communicates effectively, and stays abreast of the latest industry trends and technologies.
Technical Proficiency
A strong candidate has a solid foundation in iOS development frameworks and languages, particularly Swift and Objective-C, and is familiar with Apple's design principles and interface guidelines. They should be skilled in using tools like Xcode and Instruments, and have experience with version control systems like Git.
User-Centric Design
An understanding of user experience (UX) design principles is crucial. This includes the ability to create apps that are not only functional but also intuitive and engaging, with a focus on crafting seamless user journeys within the app.
Problem-Solving Skills
Good iOS developer candidates excel in solving complex technical problems and can think critically about how to build scalable and maintainable codebases. They should be able to troubleshoot and debug effectively, ensuring high-quality app performance.
Adaptability and Continuous Learning
The technology landscape is ever-changing, and a good iOS developer must be adaptable and committed to continuous learning. They should be up-to-date with the latest iOS updates, features, and best practices, and be willing to quickly learn and implement new technologies and frameworks.
Collaborative Team Player
Collaboration is key in app development. A good candidate should be able to work well with cross-functional teams, including designers, product managers, and other developers, to ensure a cohesive and efficient development process.
Effective Communication
Strong communication skills are essential for a good iOS developer. They must be able to articulate technical concepts to non-technical stakeholders and work closely with teams to define requirements, set expectations, and deliver results.
Attention to Detail
Attention to detail is paramount in creating polished, bug-free apps. A good candidate pays close attention to the nuances of app design and functionality, ensuring a high-quality end product that aligns with user expectations and business objectives.
By embodying these qualities, an iOS developer candidate can demonstrate their readiness to contribute to a team and create impactful, high-quality iOS applications that stand out in the competitive app marketplace.
Interview FAQs for iOS Developers
What is the most common interview question for iOS Developers?
"How do you manage memory in an iOS app?" This question probes your understanding of memory management and performance optimization in iOS development. A comprehensive answer should highlight your proficiency with Automatic Reference Counting (ARC), your strategies for avoiding retain cycles, and your experience with memory profiling tools like Instruments. It's crucial to convey your ability to write efficient, leak-free code and your proactive approach to maintaining optimal app performance.
What's the best way to discuss past failures or challenges in a iOS Developer interview?
To demonstrate problem-solving skills in an iOS Developer interview, detail a complex coding issue you faced. Explain your methodical debugging process, how you dissected the code to isolate the problem, and the creative coding solutions you implemented. Highlight your use of Xcode tools, collaboration with team members for insights, and how your resolution optimized the app's performance or user experience, showcasing your technical acumen and results-oriented mindset.
How can I effectively showcase problem-solving skills in a iOS Developer interview?
To demonstrate problem-solving skills in an iOS Developer interview, detail a complex coding issue you faced. Explain your methodical debugging process, how you dissected the code to isolate the problem, and the creative coding solutions you implemented. Highlight your use of Xcode tools, collaboration with team members for insights, and how your resolution optimized the app's performance or user experience, showcasing your technical acumen and results-oriented mindset.
Up Next
iOS Developer Job Title Guide
Copy Goes Here.
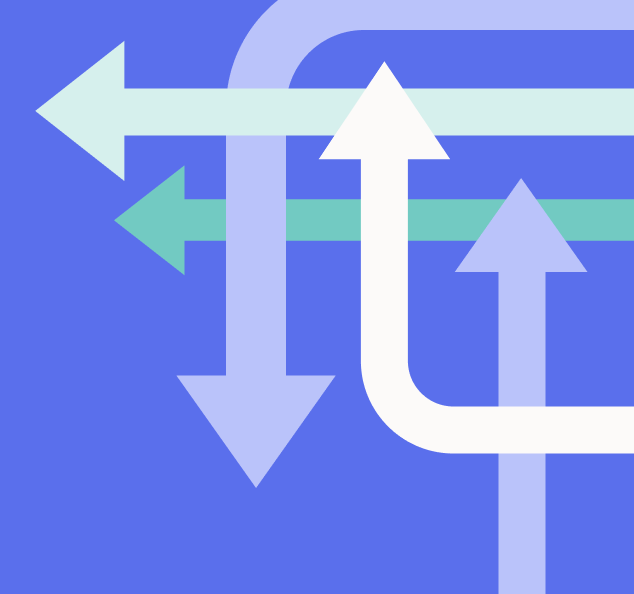