Interviewing as a Android Developer
Navigating the path to becoming a successful Android Developer often hinges on the pivotal moment of the interview—a stage where technical prowess meets the art of communication. Android Developer interviews are multifaceted, designed to assess not only your coding skills and familiarity with the Android ecosystem but also your problem-solving mindset and adaptability to rapidly evolving technologies.
In this guide, we'll dissect the array of questions that Android Developers face, from the intricacies of Java and Kotlin to the architectural patterns that underpin robust app development. We'll provide insights into crafting responses that showcase your expertise, the preparation necessary to anticipate the trends in Android development, and the strategic questions to pose to your potential employers. This resource is tailored to elevate your interview readiness, positioning you to impress as a candidate who is not only technically adept but also deeply in tune with the demands of the Android development landscape.
Types of Questions to Expect in a Android Developer Interview
Android Developer interviews are designed to probe not only your technical expertise but also your problem-solving abilities and collaboration skills. As an Android Developer, you should be prepared for a range of questions that test your knowledge of the Android platform, coding proficiency, design principles, and your ability to work within a team. Here's an overview of the types of questions you can expect and what they aim to uncover about your qualifications for the role.
Technical Knowledge and Coding Questions
Technical questions form the backbone of any Android Developer interview. These questions assess your familiarity with the Android ecosystem, including core APIs, libraries, and development tools. Expect to write code on a whiteboard or in an IDE, demonstrating your ability to solve programming challenges and debug issues. You'll likely encounter questions on Java and Kotlin, the two primary languages for Android development, as well as inquiries about Android SDK components, such as Activities, Services, and Broadcast Receivers.
Architecture and Design Pattern Questions
A solid understanding of software architecture and design patterns is crucial for creating robust and scalable Android applications. Interviewers will ask about your experience with architectural patterns like MVC, MVP, and MVVM, which are commonly used in Android app development. These questions test your ability to design a maintainable and testable codebase, as well as your knowledge of best practices for app architecture.
Behavioral Questions
Behavioral questions aim to understand how you operate within a team and handle real-world work situations. You'll be asked about past projects, how you've dealt with conflicts, and your approach to problem-solving. These questions are intended to gauge your soft skills, such as communication, teamwork, and adaptability, which are as important as your technical skills in a collaborative environment.
Performance Optimization and Testing Questions
Performance is key in mobile applications, and Android Developers must be adept at optimizing app speed and efficiency. Interviewers will ask about your strategies for reducing app load times, managing memory usage, and minimizing power consumption. Additionally, expect questions on testing practices, including unit testing, integration testing, and UI testing, to evaluate your commitment to quality and your proactive approach to catching and fixing bugs.
Practical Application and Problem-Solving Questions
To assess your practical skills, you may be given a problem to solve or a feature to implement on the spot. These exercises demonstrate your thought process, coding proficiency, and ability to apply your knowledge in a real-world context. They also test your problem-solving skills and your ability to work under time constraints, which are common in a fast-paced development environment.
By understanding these question types and preparing for them, you can showcase your full range of skills and qualities as an Android Developer. Tailoring your study and practice to these areas will help you enter the interview with confidence and improve your chances of success.
Preparing for a Android Developer Interview
Preparing for an Android Developer interview is a critical step in showcasing your technical abilities, problem-solving skills, and passion for mobile development. A well-prepared candidate stands out by demonstrating a deep understanding of Android development principles, current best practices, and the ability to apply this knowledge to real-world scenarios. This preparation not only helps you answer technical questions with confidence but also shows your commitment to the craft and your potential as a valuable team member.
How to do Interview Prep as an Android Developer
- Review Android Fundamentals: Ensure you have a strong grasp of core concepts such as Android life cycle, views, layouts, manifest, intents, and services. Revisit the official Android Developer documentation to refresh your knowledge.
- Understand the Latest Android Technologies: Stay updated with the latest Android updates, libraries, and components such as Jetpack, Kotlin Coroutines, and LiveData. Knowing current trends and tools can give you an edge in the interview.
- Practice Coding and Algorithm Questions: Sharpen your coding skills by practicing algorithm and data structure questions. Use platforms like LeetCode or HackerRank to get accustomed to the types of coding challenges you might face.
- Work on a Personal Project: Having a personal Android project to discuss can be a strong testament to your skills and interest. It allows you to demonstrate practical experience with app development and problem-solving.
- Prepare for Behavioral Questions: Reflect on your past experiences and be ready to discuss how you've handled challenges, worked in teams, and managed projects. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
- Understand Design Patterns and Best Practices: Be prepared to discuss common design patterns used in Android development, such as MVC, MVP, and MVVM, and explain why and how you would use them.
- Review the Company's Apps: If the company has Android apps, download and use them. Be prepared to provide constructive feedback or discuss how you could contribute to these apps.
- Prepare Your Own Questions: Develop insightful questions to ask the interviewer about the company's development practices, team structure, and product roadmap. This shows your genuine interest in the role and the company.
- Mock Interviews: Practice with mock interviews, either with peers or through services that offer interview simulations. This can help you get comfortable with the format and receive feedback on your performance.
By following these steps, you'll be able to demonstrate not just your technical expertise, but also your proactive approach and dedication to your development career. Remember, an interview is a two-way street; it's as much about you assessing the company as it is about them assessing you. Good preparation ensures you can make the most of this opportunity.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free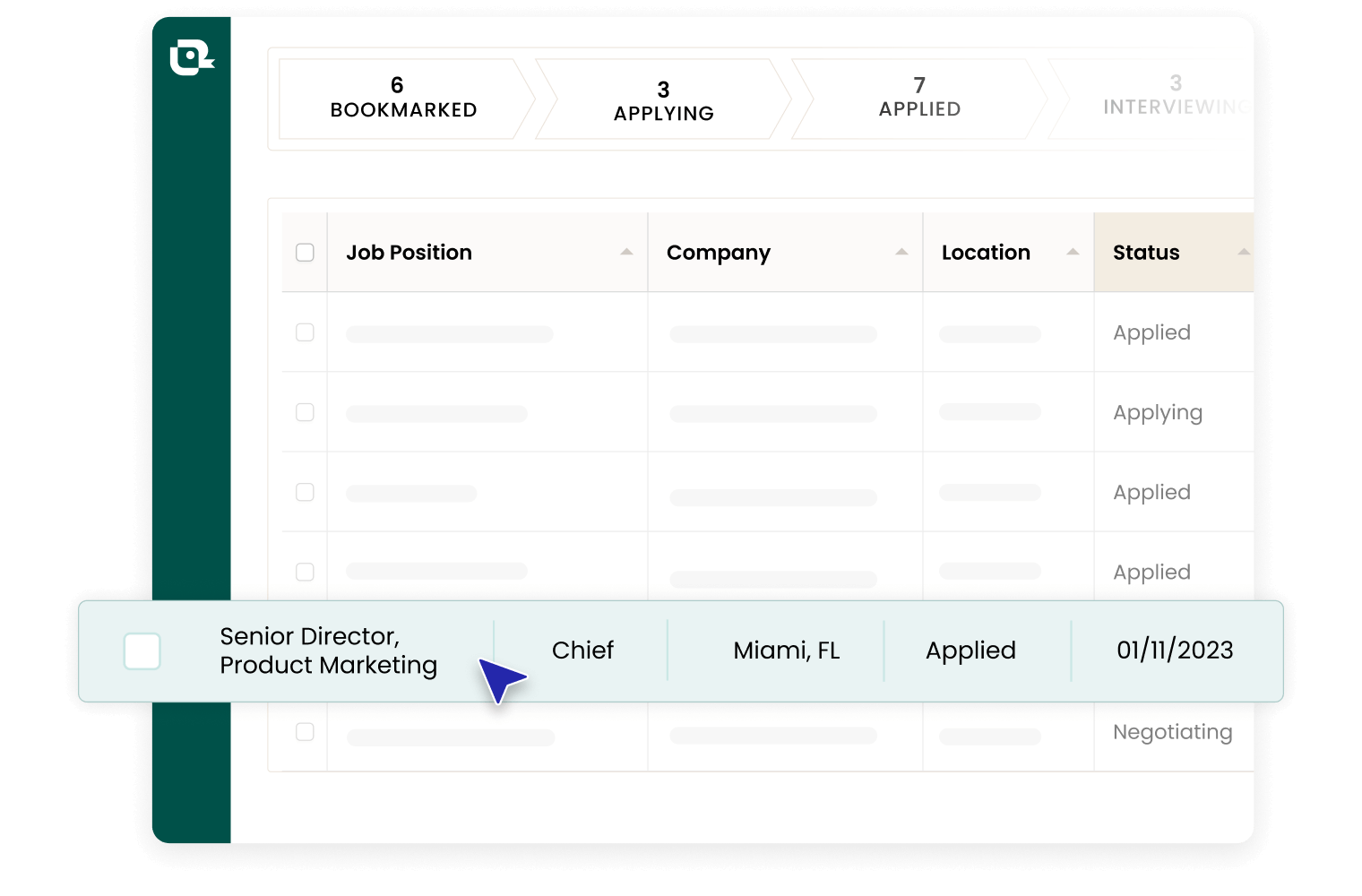
Android Developer Interview Questions and Answers

"What are the key components of the Android application architecture?"
This question assesses your understanding of the Android framework and your ability to design robust and maintainable applications.
How to Answer It
Discuss the four main components (Activities, Services, Broadcast Receivers, Content Providers) and how they interact within the Android application architecture. Explain the role of the Android Manifest file and how you use Intents to facilitate communication between components.
Example Answer
"In Android, the main components are Activities, Services, Broadcast Receivers, and Content Providers. Activities provide the UI for users to interact with, Services handle background processing, Broadcast Receivers listen for system events, and Content Providers manage shared data. The Android Manifest declares these components and their permissions. Intents are used to request functionality from other components, such as starting an Activity or Service."

"How do you manage memory leaks in Android applications?"
This question evaluates your knowledge of common issues in Android development and your ability to troubleshoot and optimize app performance.
How to Answer It
Explain what memory leaks are, why they occur in Android, and the tools and practices you use to detect and prevent them, such as LeakCanary or Android Profiler.
Example Answer
"To manage memory leaks, I first identify them using tools like LeakCanary and the Android Profiler. Common causes include static references to Activities or Views and anonymous classes holding a reference to the enclosing class. To prevent leaks, I use WeakReferences when necessary and ensure that all listeners and callbacks are unregistered when an Activity or Fragment is destroyed."

"Describe the Activity lifecycle. How do you handle configuration changes in Android?"
This question tests your understanding of the Activity lifecycle and your ability to maintain a seamless user experience during configuration changes.
How to Answer It
Detail the Activity lifecycle methods and their purposes. Explain how you handle configuration changes, such as screen rotations, by preserving UI state and using lifecycle-aware components.
Example Answer
"The Activity lifecycle consists of methods like onCreate(), onStart(), onResume(), onPause(), onStop(), and onDestroy(). To handle configuration changes, I use onSaveInstanceState() to save the Activity's state and restore it in onCreate() or onRestoreInstanceState(). For complex UI states, I use ViewModel to retain the state across configuration changes."

"Explain how you implement secure data storage in Android applications."
This question probes your knowledge of security best practices and your ability to protect sensitive information within an app.
How to Answer It
Discuss the various storage options in Android and how you choose the most secure method for different types of data. Mention the use of encryption and Android's Keystore system.
Example Answer
"For secure data storage, I use SharedPreferences for small amounts of data with encryption, and for larger datasets, I use the Room database with full-disk encryption. I also utilize Android's Keystore system to securely generate and store cryptographic keys, ensuring that sensitive data is protected both at rest and in transit."

"How do you optimize the performance of an Android application?"
This question assesses your ability to write efficient code and optimize app performance to enhance the user experience.
How to Answer It
Describe the strategies and tools you use to profile and optimize app performance, such as reducing memory usage, optimizing layouts, and using background threads for long-running operations.
Example Answer
"To optimize performance, I focus on efficient memory usage, reducing overdraw by simplifying layouts, and using RecyclerView for list-based UIs. I also profile the app with the Android Profiler to identify bottlenecks. For long-running operations, I use WorkManager or AsyncTaskLoader to perform tasks in the background without blocking the UI thread."

"Can you discuss how you use threading in Android?"
This question explores your understanding of concurrency in Android and your ability to write responsive applications.
How to Answer It
Explain the importance of threading in Android, the problems it solves, and the mechanisms you use for background processing, such as AsyncTask, HandlerThread, or Kotlin Coroutines.
Example Answer
"In Android, threading is crucial for performing time-consuming tasks without freezing the UI. I use AsyncTask for short operations and HandlerThread for longer tasks that require a looper. Recently, I've been using Kotlin Coroutines, which simplify asynchronous programming and make the code more readable and maintainable."

"What is Dependency Injection and how do you implement it in Android?"
This question gauges your understanding of software design patterns and your ability to write modular, testable code.
How to Answer It
Define Dependency Injection and its benefits. Discuss how you implement it in Android using popular libraries like Dagger or Hilt, and provide examples from your experience.
Example Answer
"Dependency Injection is a design pattern that allows for loose coupling and easier testing by providing dependencies to components rather than having them construct dependencies themselves. In Android, I use Hilt, which is built on top of Dagger, to automate this process. It simplifies the setup and ensures that dependencies are properly managed and scoped."

"How do you ensure the backward compatibility of your Android applications?"
This question checks your ability to develop applications that work across various Android versions and device configurations.
How to Answer It
Discuss the use of Support Libraries, AndroidX, and testing strategies to ensure your app functions correctly on different Android versions and screen sizes.
Example Answer
"To ensure backward compatibility, I use the AndroidX libraries, which provide a consistent API across different Android versions. I also use the @RequiresApi annotation to guard against calls to newer APIs on older devices. For testing, I use a combination of unit tests, UI tests with Espresso, and manual testing on a range of devices and emulators to cover various screen sizes and Android versions."Which Questions Should You Ask in a Android Developer Interview?
In the competitive field of Android development, the questions you ask in an interview can be as revealing as the answers you provide. They not only demonstrate your technical acumen and enthusiasm for the role but also your strategic thinking and cultural fit within the organization. For aspiring Android Developers, asking incisive questions is a chance to stand out as a candidate who is both reflective and proactive. It's also your opportunity to ensure the position aligns with your career objectives, work style, and values. By asking thoughtful questions, you convey a strong interest in the role while also gathering essential information to determine if the opportunity is the right one for you.
Good Questions to Ask the Interviewer
"Can you describe the current tech stack for Android development in the company and how it has evolved?"
This question showcases your interest in the company's technology choices and your understanding of how tech stacks can evolve to meet changing needs. It also gives you insight into the company's adaptability and willingness to adopt new technologies.
"What is the process for design and code reviews, and how does the team handle collaboration and knowledge sharing?"
Asking about the review process and collaboration indicates that you value quality and teamwork. It helps you understand the company's approach to maintaining code quality and how you would fit into their development ecosystem.
"How does the company approach testing for Android applications?"
Inquiring about testing practices reveals your commitment to delivering robust applications. It also provides you with an understanding of the company's quality assurance processes and how seriously they take app stability and user experience.
"What are the most significant challenges the Android development team has faced recently, and how were they overcome?"
This question allows you to gauge the complexity of the projects you might be working on and the company's problem-solving culture. It also demonstrates your willingness to engage with challenges and contribute to future solutions.
What Does a Good Android Developer Candidate Look Like?
In the realm of Android development, a standout candidate is someone who not only possesses a deep understanding of the technical aspects of building Android applications but also exhibits a strong sense of design, user experience, and problem-solving skills. Employers and hiring managers are on the lookout for candidates who can demonstrate a robust knowledge of Android SDKs, programming languages, and development tools, while also showing an ability to adapt to new technologies and methodologies. A good Android developer candidate is expected to be a team player, capable of collaborating with UI/UX designers, product managers, and other developers to create seamless and efficient applications that cater to the needs of users and align with the company's objectives.
Technical Proficiency
A strong candidate will have a solid grasp of Android fundamentals, including activities, fragments, and services, as well as a proficiency in programming languages such as Java and Kotlin. They should be familiar with Android development tools like Android Studio, version control systems like Git, and have experience with databases and RESTful APIs.
Design and User Experience Sensibility
Understanding the importance of UI/UX design in mobile applications is crucial. Good candidates can follow Material Design guidelines and create intuitive, user-friendly interfaces that enhance the user experience.
Problem-Solving and Critical Thinking
The ability to troubleshoot and solve complex technical issues is highly valued. Candidates should demonstrate strong analytical skills and the capacity to think critically to overcome development challenges.
Adaptability and Continuous Learning
Technology evolves rapidly, and so do best practices in Android development. A desirable candidate is one who shows a commitment to continuous learning and adaptability to new tools, languages, and frameworks.
Collaboration and Communication
Android development is often a team effort. A good candidate must be able to effectively communicate technical concepts to team members of varying technical expertise and collaborate with cross-functional teams to achieve project goals.
Performance Optimization
Candidates should understand the intricacies of Android performance, including memory management, efficient use of resources, and application responsiveness. They should be able to diagnose performance bottlenecks and implement optimizations.
By embodying these qualities, an Android developer candidate can position themselves as a valuable asset to potential employers, demonstrating not only their technical capabilities but also their ability to contribute to the creation of high-quality, user-centric mobile applications.
Interview FAQs for Android Developers
What is the most common interview question for Android Developers?
"How do you ensure your Android app performs well across different devices?" This question evaluates your understanding of Android's fragmented ecosystem. A comprehensive answer should highlight your approach to testing on various screen sizes, resolutions, and OS versions, along with your proficiency in using tools like Android Studio's profiler and the importance of optimizing layouts and resources for performance and battery efficiency.
What's the best way to discuss past failures or challenges in a Android Developer interview?
To demonstrate problem-solving skills in an Android Developer interview, recount a complex coding issue you faced. Explain your systematic debugging process, how you isolated the problem, and your rationale for the chosen solution. Highlight how you optimized app performance or user experience as a result. This shows your technical acumen, attention to detail, and commitment to delivering a high-quality product.
How can I effectively showcase problem-solving skills in a Android Developer interview?
To demonstrate problem-solving skills in an Android Developer interview, recount a complex coding issue you faced. Explain your systematic debugging process, how you isolated the problem, and your rationale for the chosen solution. Highlight how you optimized app performance or user experience as a result. This shows your technical acumen, attention to detail, and commitment to delivering a high-quality product.
Up Next
Android Developer Job Title Guide
Copy Goes Here.
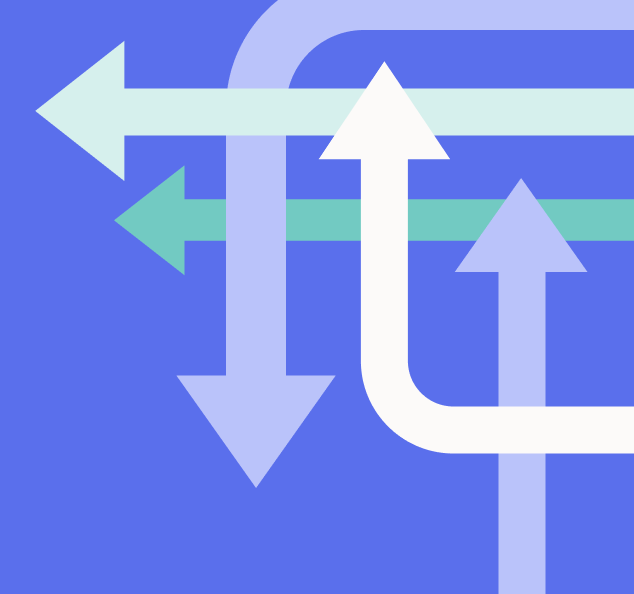