Interviewing as a Golang Developer
Embarking on the journey to become a Golang Developer is an exciting venture in the ever-evolving landscape of software engineering. With Go's increasing popularity for its efficiency and concurrency capabilities, interviews for Golang Developers are designed to probe not only your technical prowess but also your ability to solve complex problems with elegant, scalable solutions.
In this guide, we'll unravel the layers of a Golang Developer interview, from dissecting the intricacies of technical questions to mastering coding challenges and system design. We'll provide you with the insights needed to showcase your proficiency in Go, your understanding of software development principles, and the traits that make a 'great' Golang Developer. This resource is meticulously crafted to equip you with the knowledge and confidence required to excel in your interviews and propel your career forward as a skilled Golang Developer.
Types of Questions to Expect in a Golang Developer Interview
Golang Developer interviews are designed to probe not only your technical expertise with the language but also your problem-solving abilities, understanding of systems design, and your approach to coding and collaboration. Recognizing the types of questions you may encounter will help you prepare effectively and demonstrate your qualifications for the role. Here's an overview of the common question categories and what they aim to uncover about your potential as a Golang Developer.
Language-Specific Technical Questions
These questions delve into your knowledge of Go's syntax, features, and idioms. Expect to answer questions about Go's type system, concurrency model (goroutines and channels), memory management, and other language-specific details. These questions assess your fluency in Go and your ability to write efficient, idiomatic code.
Code Review and Optimization Questions
You may be asked to review snippets of Go code for correctness, performance, and adherence to best practices. This evaluates your attention to detail, your understanding of Go's performance characteristics, and your ability to optimize and refactor code. It also tests your ability to communicate code improvements clearly and constructively.
System Design and Architecture Questions
Interviewers will be interested in your ability to design scalable and maintainable systems. Questions in this category might involve designing a backend service, API, or other components using Go. They test your architectural knowledge, including how to handle concurrency, manage state, and integrate with other systems and services.
Problem-Solving and Algorithm Questions
These questions are common in software engineering interviews and involve data structures and algorithms. You'll be expected to solve problems using Go, which will demonstrate your analytical thinking and proficiency in translating solutions into code. While they may not be Go-specific, they show your foundational computer science knowledge.
Behavioral and Collaboration Questions
Golang Developers often work as part of a team, so expect questions about past projects, team interactions, and how you've handled conflicts or challenges. These questions evaluate your soft skills, including communication, teamwork, and your ability to learn from and contribute to a collective effort.
Practical Coding Tasks
You might be given a real-world coding task to complete in a certain amount of time, either during or before the interview. This hands-on test will assess your coding skills in a practical context, including how you approach a problem, organize your code, test your solution, and handle edge cases.
Understanding these question types and preparing for them can help you showcase the depth of your Golang expertise and your readiness to tackle the challenges of a developer role. Tailoring your study and practice to these categories will enable you to approach your interview with confidence and clarity.
Preparing for a Golang Developer Interview
Preparing for a Golang Developer interview is a critical step in showcasing your technical expertise and passion for the language. It's not just about proving your coding abilities; it's about demonstrating a deep understanding of Go's features, best practices, and how to apply them effectively in real-world scenarios. A well-prepared candidate stands out by displaying a strong grasp of the language's nuances, concurrency model, and performance optimization techniques. This preparation not only reflects your technical acumen but also your commitment to personal growth and professional development in the field of Go programming.
How to do Interview Prep as a Golang Developer
- Master the Go Language: Ensure you have a solid understanding of Go's syntax, data structures, interfaces, and error handling. Be prepared to write idiomatic Go code and explain why it's efficient and effective.
- Understand Concurrency Patterns: Go is known for its concurrency model. Familiarize yourself with goroutines, channels, and the sync package. Be ready to discuss how you would handle concurrent tasks and potential race conditions.
- Review Go Tooling and Ecosystem: Be knowledgeable about the Go toolchain, including gofmt, govet, and godoc. Understand how to manage dependencies with tools like Go Modules and be aware of popular Go frameworks and libraries.
- Practice with Go Code Challenges: Solve coding problems using Go on platforms like LeetCode, HackerRank, or Exercism. This will help you think algorithmically and improve your coding fluency in Go.
- Study Common Design Patterns: While Go may not use design patterns in the same way as some other languages, it's still important to understand common patterns like singleton, factory, and worker pool within the context of Go.
- Prepare for System Design Questions: You may be asked to design a system or architecture using Go. Be ready to discuss how you would structure your code, handle dependencies, and ensure scalability and maintainability.
- Brush Up on Testing: Understand the importance of testing in Go. Be familiar with writing unit tests using the built-in testing package and discuss the role of benchmarking and profiling in Go applications.
- Review Your Past Go Projects: Be prepared to talk about your previous work with Go, including challenges you faced and how you overcame them. Highlight any contributions to open-source Go projects or unique solutions you've implemented.
- Prepare Your Own Questions: Develop insightful questions about the company's use of Go, their development practices, or any specific Go-based projects they have. This shows your genuine interest and curiosity about the role.
- Mock Interviews: Practice with a peer or mentor who is familiar with Go. They can provide valuable feedback on your coding style, problem-solving approach, and communication skills.
By following these steps, you'll be able to demonstrate not just your technical skills, but also your holistic understanding of Go and how it fits within the larger context of software development. This preparation will help you to engage confidently in discussions during your interview and prove that you are a well-rounded and proficient Golang Developer.
Stay Organized with Interview Tracking
Worry less about scheduling and more on what really matters, nailing the interview.
Simplify your process and prepare more effectively with Interview Tracking.
Sign Up - It's 100% Free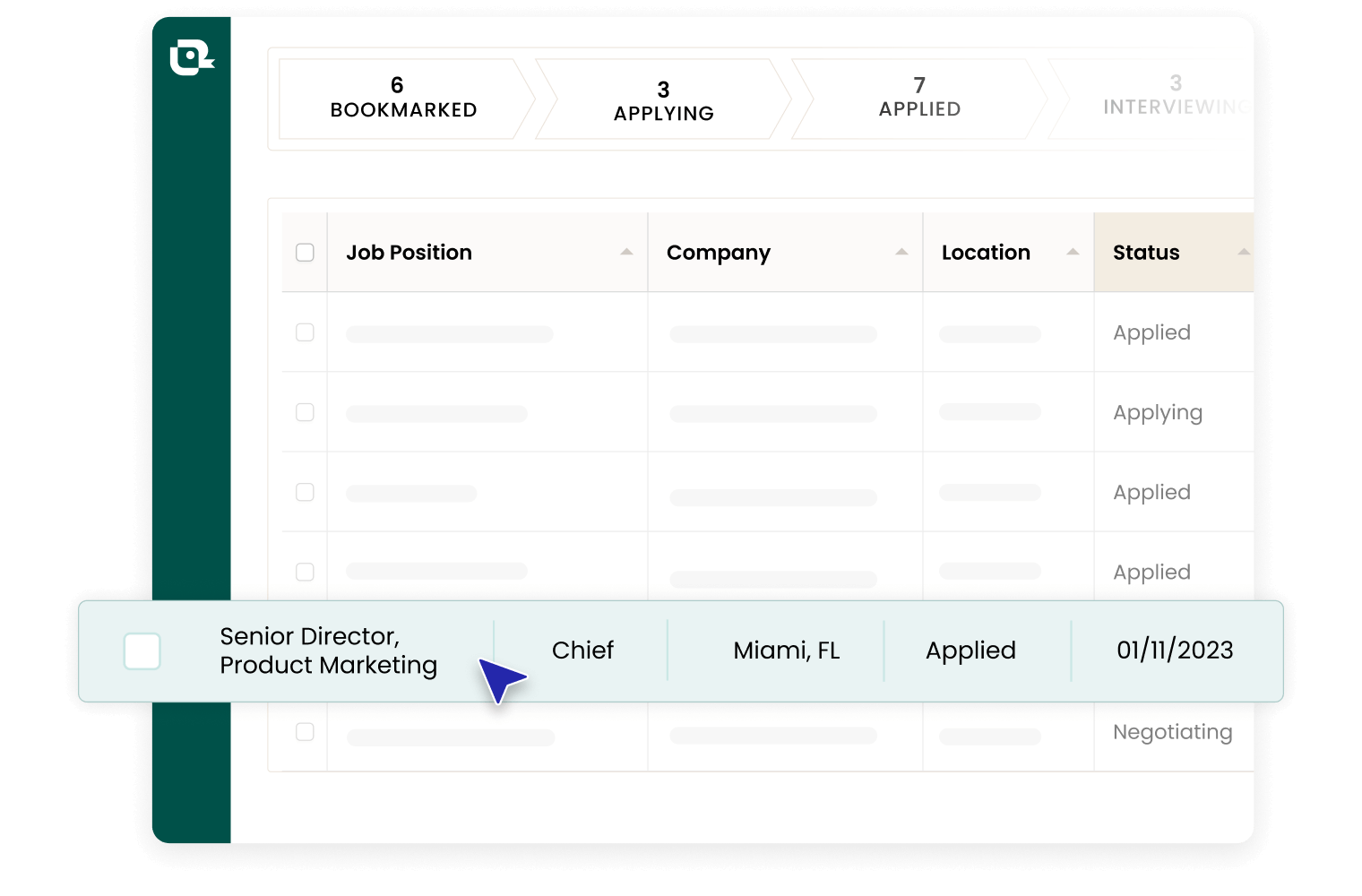
Golang Developer Interview Questions and Answers

"What are some advantages of using Go, and how have you utilized these in your past projects?"
This question assesses your understanding of Go's strengths and how you can leverage them in practical scenarios. It's an opportunity to demonstrate your knowledge of the language and its application in real-world development.
How to Answer It
Discuss specific features of Go that you find beneficial, such as its simplicity, concurrency support, or performance. Provide examples of how these features have positively impacted your past projects.
Example Answer
"In my previous role, I took advantage of Go's concurrency model, which is built around goroutines and channels, to develop a high-performance data processing tool. This allowed us to handle large volumes of data with minimal latency. Go's simplicity and powerful standard library also enabled rapid development and easy maintenance of the codebase."

"How do you manage dependencies in a Go project?"
This question evaluates your familiarity with Go's package management and your ability to organize and maintain a clean project environment.
How to Answer It
Explain the tools and practices you use for dependency management in Go, such as Go Modules, and discuss how you ensure reproducible builds and manage versioning.
Example Answer
"I use Go Modules for dependency management, which provides a reliable and efficient way to handle project dependencies. In my last project, I ensured that all dependencies were specified with version tags to avoid breaking changes and to maintain reproducibility. I also regularly updated dependencies to keep the project secure and up-to-date."

"Can you explain how interface works in Go and provide an example of how you've used it?"
This question tests your understanding of Go's type system and your ability to use interfaces to design flexible and reusable code.
How to Answer It
Describe the concept of interfaces in Go and how they differ from other languages. Provide a concrete example of how you've implemented an interface to abstract functionality in a project.
Example Answer
"In Go, interfaces are a way to specify the behavior of an object: if something can do this, then it can be used here. I've used interfaces to create a decoupled system where the core logic was independent of the data source. For instance, I designed a DataReader interface to abstract the reading of data, which allowed us to switch from a file-based system to a database without altering the core processing logic."

"What is a goroutine, and how does it differ from a thread?"
This question probes your understanding of Go's concurrency model and your ability to articulate the differences between its lightweight goroutines and traditional OS threads.
How to Answer It
Explain what goroutines are and how they are scheduled. Compare them with threads in terms of resource usage and context switching.
Example Answer
"A goroutine is a lightweight thread managed by the Go runtime. Unlike OS threads, goroutines have a smaller stack that grows and shrinks as needed, which allows for the creation of thousands of goroutines at a minimal cost. I've used goroutines to build a concurrent web scraper that could handle multiple downloads simultaneously without overwhelming system resources."

"How do you ensure your Go code is performant and efficient?"
This question assesses your ability to write optimized code and your knowledge of Go's tools and best practices for performance tuning.
How to Answer It
Discuss the strategies and tools you use to profile and improve the performance of Go applications, such as benchmarking and the pprof tool.
Example Answer
"To ensure code performance, I regularly write benchmarks using Go's built-in testing package. I also use the pprof tool to profile the application and identify bottlenecks. For example, in a recent API project, profiling revealed excessive memory allocations in a critical path. I optimized the code by reusing objects and reducing allocations, which significantly improved the API's response times."

"Describe how you handle error handling in Go."
This question explores your approach to one of Go's core concepts: error handling. It's a chance to demonstrate your understanding of idiomatic Go practices.
How to Answer It
Explain Go's explicit error handling philosophy and how you apply it in your code to ensure robustness and clarity.
Example Answer
"Go encourages explicit error handling rather than exceptions. I follow the convention of returning errors as the last return value from functions and handling them immediately. In a REST API I developed, I created a centralized error handling middleware that could interpret different error types and return the appropriate HTTP status code and message, ensuring consistency and ease of debugging."

"What strategies do you use for testing in Go?"
This question evaluates your experience with testing in Go and your commitment to ensuring code quality.
How to Answer It
Discuss the testing tools and methodologies you use in Go, such as the built-in testing package, table-driven tests, and mocking.
Example Answer
"I use Go's built-in testing package to write unit tests, often employing table-driven tests to cover a wide range of input scenarios. For integration tests, I use the httptest package to simulate HTTP requests and responses. In my last project, I also used a mocking library to isolate and test the interactions with external services, which helped us catch several potential bugs early in the development process."

"How do you manage state in a concurrent Go application?"
This question tests your ability to design concurrent systems in Go that are safe and free of race conditions.
How to Answer It
Explain the techniques and patterns you use to manage shared state in concurrent Go applications, such as using channels or sync primitives.
Example Answer
"In concurrent Go applications, managing state safely is crucial to avoid race conditions. I prefer using channels to communicate between goroutines, as they provide a clear and idiomatic way to synchronize access to shared resources. For example, in a recent project, I used a channel to implement a worker pool pattern, which allowed multiple goroutines to process tasks concurrently without stepping on each other's toes. When channels aren't suitable, I use the sync package's Mutex or atomic functions to protect shared state."Which Questions Should You Ask in a Golang Developer Interview?
In the competitive field of software development, Golang developers are often assessed not just on their technical prowess but also on their engagement and fit within a team. Asking insightful questions during an interview can significantly influence the interviewer's perception of you as a proactive and thoughtful candidate. Moreover, it's a strategic move to ensure the role aligns with your career goals and values. For Golang developers, the questions you ask can highlight your understanding of Go's ecosystem, your foresight into the industry's trends, and your compatibility with the company's engineering culture. By asking targeted questions, you can uncover crucial details about the company's technical challenges, growth opportunities, and the nuances of your potential role, helping you make an informed decision about your next career move.
Good Questions to Ask the Interviewer
"Can you describe the current Go projects and what role the new hire will play in these initiatives?"
This question demonstrates your eagerness to understand the specifics of what you'll be working on and how you can contribute. It also gives you insight into the team's current priorities and the expectations for your position.
"How does the team manage code reviews and ensure code quality for Go applications?"
Asking about code review practices shows your commitment to maintaining high standards and your interest in the team's approach to collaboration and mentorship. It can also reveal the company's commitment to best practices in software development.
"What are the biggest technical challenges the team is facing with Go, and how do you foresee a new developer helping to address these?"
This question allows you to gauge the complexity of the problems the team is tackling and how your skills might be applied. It also provides a window into the company's problem-solving culture and the technical debt they might be dealing with.
"How does the company stay current with the evolving Go ecosystem, and what opportunities are there for professional development?"
Inquiring about professional development opportunities and how the company keeps up with the Go ecosystem shows your intent to grow and stay updated in your field. It also helps you understand if the company values continuous learning and supports their developers' growth.
What Does a Good Golang Developer Candidate Look Like?
In the realm of software development, Golang, also known as Go, has emerged as a powerful language favored for its simplicity and efficiency in handling concurrent tasks and complex back-end systems. A good Golang developer candidate is not only proficient in the language itself but also exhibits a strong understanding of concurrent programming, system design, and performance optimization. They are analytical thinkers with a knack for writing clean, maintainable code that scales well. Employers and hiring managers are on the lookout for candidates who can demonstrate practical coding skills along with the ability to collaborate effectively with teams, adapt to evolving project requirements, and contribute to the continuous improvement of the codebase.
A good Golang developer is expected to be a problem-solver who can leverage Go's features to build robust and efficient applications. They should be able to work independently as well as part of a team, showing initiative and a commitment to best practices.
Proficiency in Go and Its Ecosystem
A strong candidate has a deep understanding of Go's syntax, idioms, and toolchain. They are familiar with the standard library, go routines, channels, and the overall ecosystem, including popular frameworks and third-party libraries.
Concurrent Programming Skills
Given Go's emphasis on concurrency, a good developer must be adept at writing concurrent code that is safe and efficient. They should understand how to manage state and synchronize processes in a concurrent environment.
Understanding of System Design
Candidates should demonstrate the ability to design scalable and maintainable systems. This includes knowledge of design patterns, microservices architecture, and the principles of clean code.
Performance Optimization
A proficient Golang developer is skilled in profiling and optimizing code for performance. They should be able to identify bottlenecks and apply best practices to improve the efficiency of applications.
Collaboration and Communication
The ability to work well with others and communicate effectively is crucial. This includes experience with version control systems like Git, code reviews, and a willingness to engage in pair programming and other collaborative practices.
Adaptability and Continuous Learning
Technology is always advancing, and a good Golang developer is one who stays updated with the latest developments in the language and related technologies. They are eager to learn and adapt to new tools and methodologies.
By embodying these qualities, a Golang developer candidate can demonstrate their value to potential employers and stand out in the competitive field of software development.
Interview FAQs for Golang Developers
What is the most common interview question for Golang Developers?
"How do you optimize Go code for performance?" This question probes your proficiency in writing efficient Go applications. A compelling answer should highlight your understanding of Go's concurrency patterns, memory management, and profiling tools. Discuss your experience with goroutines and channels for parallelism, the importance of minimizing garbage collection, and how you utilize benchmarks and the `pprof` tool to identify and address performance bottlenecks.
What's the best way to discuss past failures or challenges in a Golang Developer interview?
To demonstrate problem-solving in a Golang interview, detail a complex coding challenge you faced. Explain your methodical approach, how you broke down the problem, and the Go-specific tools or features you utilized. Discuss your iterative testing process, how you optimized for performance, and the outcome's impact on the project. This highlights your technical proficiency in Go, strategic thinking, and commitment to quality code.
How can I effectively showcase problem-solving skills in a Golang Developer interview?
To demonstrate problem-solving in a Golang interview, detail a complex coding challenge you faced. Explain your methodical approach, how you broke down the problem, and the Go-specific tools or features you utilized. Discuss your iterative testing process, how you optimized for performance, and the outcome's impact on the project. This highlights your technical proficiency in Go, strategic thinking, and commitment to quality code.
Up Next
Golang Developer Job Title Guide
Copy Goes Here.
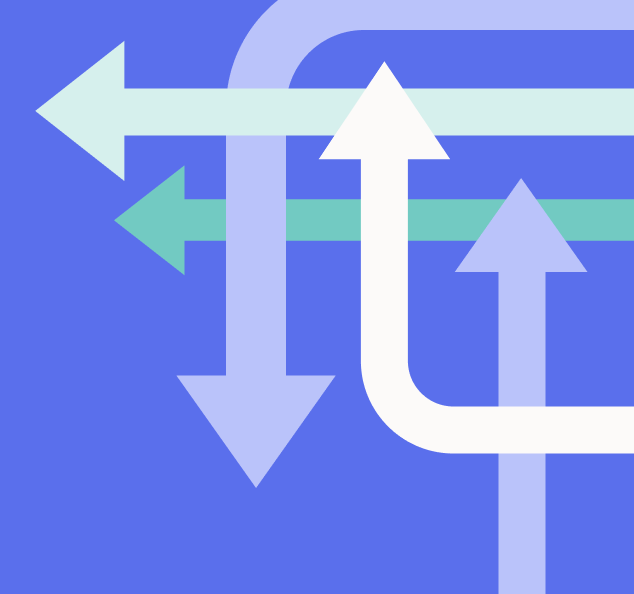